Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial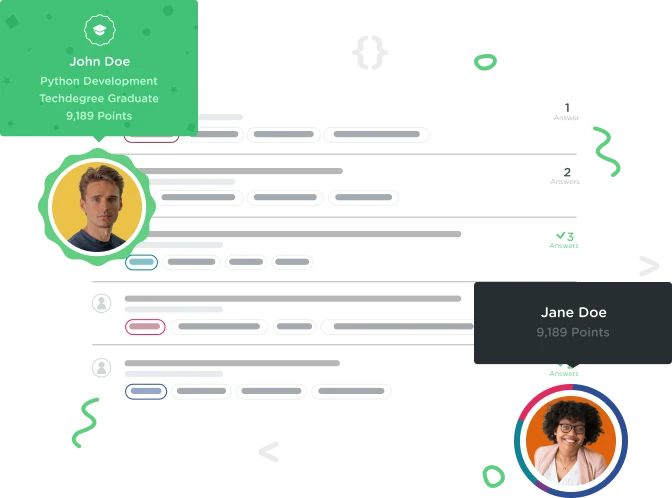

Thomas Stalcup Jr
319 PointsThis code should work but doesn't: let blueColor = UIColor(red: 0/255.0, green: 0/255.0, blue: 255/255.0, alpha: 1.0)
I've even tried:
let blueColor = UIColor(red: 0.0, green: 0.0, blue: 1.0, alpha: 1.0)
2 Answers
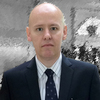
Nathan Tallack
22,160 PointsYour first code choice would not work because you cannot divide by zero. It will return an error. Your second one was close but they were asking for the blue channel to be set to 255 not 1. So the syntax was right there.
In task one you would get that code and put it at the top of the class outside of the methods declared there.
The second task in the challenge was to use that blueColor UIColor object as a parameter to change the backgroundColor of the view. The view in this case would be part of the object that is the view controller, so you would change the background color on self.view. this is what the code would look like.
class ViewController: UIViewController {
// Note we declare this at the top of the class outside of the methods. You could declare
// inside but then it could only be used within that method and not in other methods.
let blueColor = UIColor(red: 0.0, green: 0.0, blue: 255.0, alpha: 1.0)
override func viewDidLoad() {
self.view.backgroundColor = blueColor // Here we use that object as a parameter.
}
override func didReceiveMemoryWarning() {
// Dispose of any resources that can be recreated
}
}
I hope this helps. :)

Marc Schultz
23,356 PointsNathan Tallack already explained it. But here is one more thing. Don't take too much care of the "right solution" in this courses. Why, you ask? In the official documentation of UIColor, there is only one init method like init(red:green:blue:alpha:)
and all parameters are of type CGFloat. Also the parameter descriptions say something like:
The red component of the color object, specified as a value from 0.0 to 1.0.
So why should we make use of 255.0? There is no reason for this. You second solution is totally correct. But if it is not working with the challenge tests then you have to make use of the .blueColor() method.
If you really want to make use of the number 255 then use the corrected version of your first approach:
UIColor(red: 0, green: 0, blue: 255/255.0, alpha: 1.0)