Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial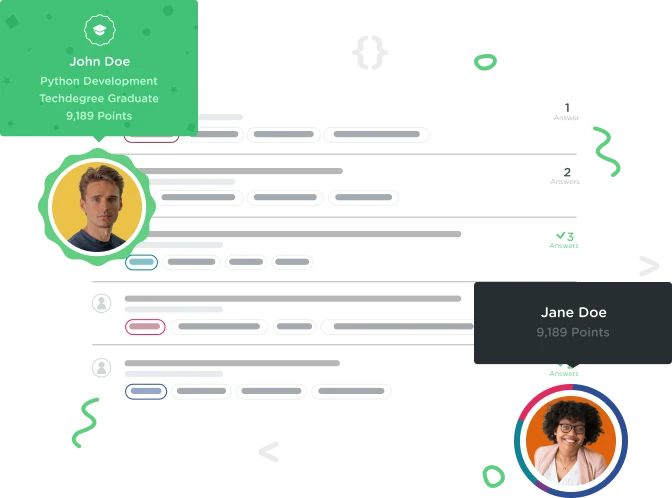

Elizabeth McInerney
3,175 PointsThis code works but I have a small question
In workspaces, this code works up until line 11. If I add line 12, I get something along the lines of "ans not defined". Why is ans not coming out of the function with my return statement?
# Handy functions:
# .upper() - uppercases a string
# .lower() - lowercases a string
# .title() - titlecases a string
# There is no function to reverse a string.
# Maybe you can do it with a slice?
test_string = "No good deed goes undone. i forgot to capitolize this."
def stringcases(test_string):
ans = test_string.upper(), test_string.lower(), test_string.title(), test_string[::-1]
return ans
stringcases(test_string)
print(ans)
5 Answers

Greg Kaleka
39,021 PointsHi Elizabeth,
On line 11, you're returning the value of ans, but then you're not doing anything with it. By the time you get to line 12 it's gone forever :). If you want to use the return value of a function, you need to store it in a variable:
test_string = "No good deed goes undone. i forgot to capitolize this."
def stringcases(test_string):
ans = test_string.upper(), test_string.lower(), test_string.title(), test_string[::-1]
return ans
caseList = stringcases(test_string)
print(caseList)
Alternatively, you could just print the return value directly if you won't need it again:
test_string = "No good deed goes undone. i forgot to capitolize this."
def stringcases(test_string):
ans = test_string.upper(), test_string.lower(), test_string.title(), test_string[::-1]
return ans
print(stringcases(test_string))
See how that works?

Elizabeth McInerney
3,175 PointsYes, I think I am struggling with the concept of variables pointing to things instead of being things. I thought I already stored it in a variable when I called it ans. Thanks!

Greg Kaleka
39,021 PointsActually, this has nothing to do with variables being or pointing to things. Rather, if a variable is defined inside a function, it is only accessible within that function. This is called variable scope.
In your example, ans
was defined in the function - this is often called a "working" variable, since it's just used to write the function. Later in your code, you're outside the function, and so ans
is "out of scope".
Here's some more detailed info about variable scope in Python. Note this is a concept in all of programming, not just Python (though it's handled somewhat differently across languages).

Elizabeth McInerney
3,175 PointsOK, thanks. I guess I figured that return was a way to move the variable out of the function. So, without a return, nothing comes out of the function. With a return, the value you created comes out, but it has no variable associated with it (until you give it a variable). So if I had used ans = stringcases(test_string), then I could have printed ans in the global part of the code.

Greg Kaleka
39,021 PointsYep - you got it! You want to be careful about variable naming, though. You could use ans = stringcases(test_string), but if you're also using ans as your working variable in the function, your code is going to be a bit confusing, since they're two completely separate variables with the same name. Better to call one of them something else :).

Elizabeth McInerney
3,175 PointsYes, that makes sense. Thanks!

Michael Pastran
4,727 Pointsdef stringcases(str):
return (str.upper(),str.lower(),str.title(), str[::-1])
this was my code. also for people who might get stuck on this code. it has to be in the order that is asked for in the question. i got stuck because i was getting the lower case version then the uppercase. lol -_-