Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial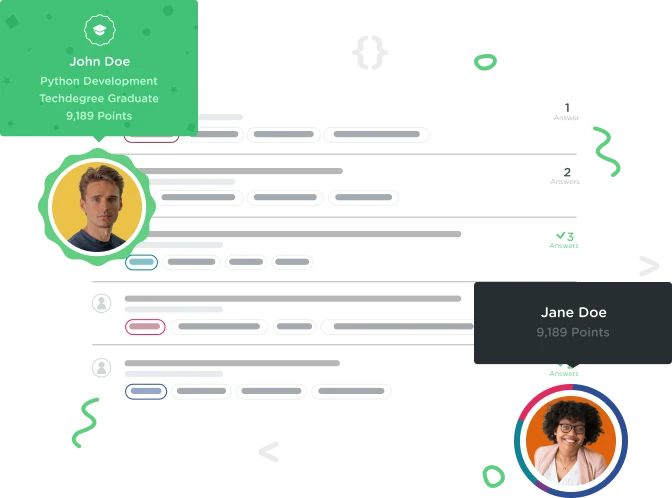

Nathan Niell
980 PointsThis code works perfectly fine in workspaces, yet it doesn't pass. What's the problem?
This is doing everything the challenge is asking for whenever I run it in workspaces. I run it in challenge and it's not getting the result it expected. Unless I'm supposed to write a try block to stop the use of numbers instead of strings I don't know what to fix.
# E.g. word_count("I do not like it Sam I Am") gets back a dictionary like:
# {'i': 2, 'do': 1, 'it': 1, 'sam': 1, 'like': 1, 'not': 1, 'am': 1}
# Lowercase the string to make it easier.
def word_count(a):
a = a.lower()
words = a.split(' ')
count = {}
for word in words:
if word in count.keys():
count[word] += 1
else:
count[word] = 1
return count
2 Answers

andren
28,558 PointsThe challenge wants you to split on all whitespace. Your code splits on spaces, which is a type of whitespace, but there are lots of other characters considered whitespace. Like line breaks, tabs, and other things like that.
The code works on the example input since that only uses spaces, but the example input the challenge shows you is often different from the value that is actually passed to the function. It often differs in order to make it harder to cheat the challenge checker by hardcoding your return to the right values.
Conveniently enough the split
method actually splits on all whitespace by default if you don't pass it any arguments, so if you remove your argument to split
like this:
def word_count(a):
a = a.lower()
words = a.split()
count = {}
for word in words:
if word in count.keys():
count[word] += 1
else:
count[word] = 1
return count
Then your code will pass.
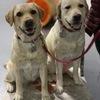
Alex Koumparos
Python Development Techdegree Student 36,887 PointsHi Nathan,
This challenge is a bit sneaky. The only clue that it gives you to what you are doing wrong is the error message:
Bummer: Hmm, didn't get the expected output. Be sure you're lowercasing the string and splitting on all whitespace!
Note that it says you need to split on 'all whitespace' and not single spaces. Your use of [split()](https://docs.python.org/3/library/stdtypes.html#str.split)
is only splitting on single spaces.
Make that one change and your code will pass.
Cheers
Alex