Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial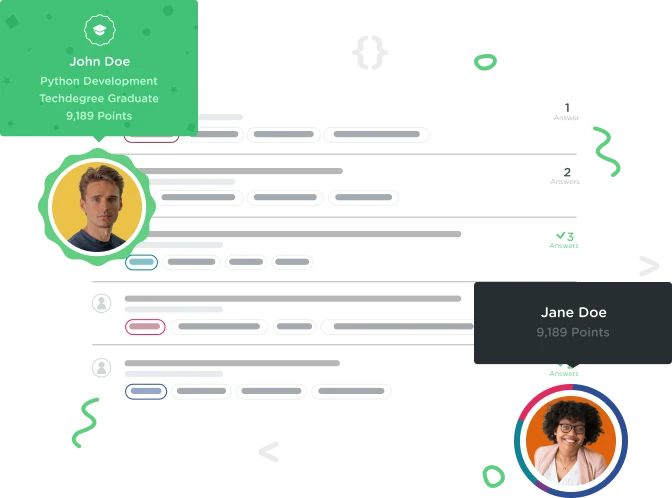

Li Zhang Tan
1,396 PointsThis disemvowel function works in the Python workspace but isn't accepted in the Code Challenge?
I don't know what's wrong with it. :(
vowels = ["a", "e", "i", "o", "u"]
def disemvowel(word):
listed = list(word)
for letter in listed:
if letter.lower() in vowels:
listed.remove(letter)
else:
pass
word = "".join(listed)
return word
3 Answers

Charlie Gallentine
12,092 PointsAs the loop runs, it alters the size of the list it is working on. I would recommend declaring a new empty list before the loop and putting letters into it which are not vowels. Thus the original list is unaltered and the new one which is only consonants can be returned.
SPOILER
vowels = ["a", "e", "i", "o", "u"]
def disemvowel(word):
listed = list(word)
new_list = [] # A new list where consonants can go
for letter in listed:
if letter.lower() not in vowels:
new_list.append(letter) # Adds letter to new_list if it is a consonant, does nothing if vowel
word = "".join(new_list)
return word

William Harrison
9,585 PointsYou are changing the size of your loop as you remove letters. If there is several vowels in a row some are missed.

Charlie Gallentine
12,092 PointsI believe that to be right. It is my understanding that in a for loop, the condition is
for [iterating piece starting with first index (0)] in [something which is iterable]:
......
By having two vowels next to each other, I think it iterates over the first one, for example the 'e' at index 2 in "Cheese", removes it, and the string becomes "Chese". The for loop has already iterated over index 2, so it moves on to index 3. With vowels next to each other, the program will miss every other one. The iterating piece of the for loop is just the name given to the incrementer.
Li Zhang Tan
1,396 PointsLi Zhang Tan
1,396 PointsSooo from what you're saying, the FOR loop, when applied to the list, doesn't remember which item it was performing the command on, instead, it remembers the position of the item it performs the command on. Which is why, when a vowel is removed from the list, the other letters shift positions, but the command doesn't realise it. Am I understanding this right?