Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial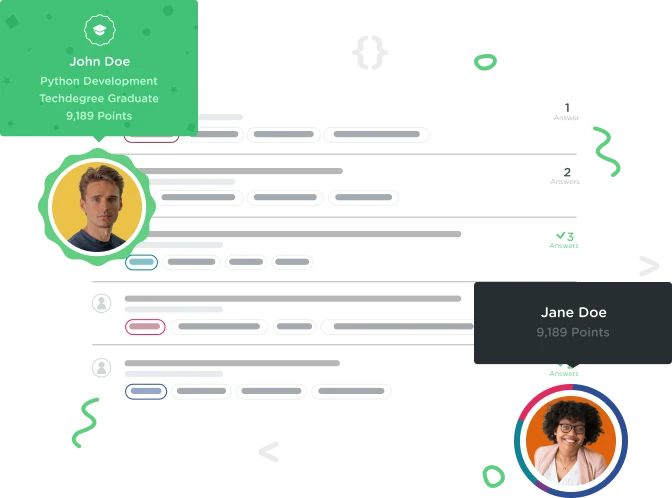
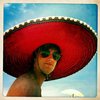
Cameron Raw
15,473 PointsThis enum stuff has gone way over my head.
I think these Java courses are great, by the way. This bit on enums, though. I just don't fully understand it on any real level.
The following code challenge talks about constructors and everything which, in classes, I do thousands of times no problem but I'm just a bit lost on enums.
5 Answers

Ken Alger
Treehouse TeacherCameron;
Enums are applicable to programming languages other than Java as well so let's do a bit of exploration on them, okay?
Enumerated Types, or Enums, allow us to define our own variables and are great for doing so to make something easier for us to read. In the Oracle documentation (link above) there is an example of playing cards. We can take that further and not only generate a deck of cards from TWO of CLUBS
to ACE of SPADES
as their sample code did, but what about actually making that useful for a card game? Sure, we can read that a TWO of CLUBS
is of a lower value than a TEN of CLUBS
, but what about making that programmatically useful?
As you likely know, the ranking value for cards in a deck is typically (or for our example):
- 2 through 10 are their face value
- Jack is 11
- Queen is 12
- King is 13
- Ace is 14
Therefore, if we made our enum
as follows we could utilize those values in a deck:
public enum CardValue
{
TWO(2),
THREE(3),
FOUR(4),
FIVE(5),
SIX(6),
SEVEN(7),
EIGHT(8),
NINE(9),
TEN(10),
JACK(11),
QUEEN(12),
KING(13),
ACE(14);
private int mCardValue;
private CardValue (int value)
{
mCardValue = value;
}
public int getCardValue() {
return mCardValue;
}
}
We can create a similar situation for our suits of cards, CLUBS
, HEARTS
, DIAMONDS
, and SPADES
, and order them as well as there are certain card games in which SPADES
is "higher" than DIAMONDS
, for example.
public enum Suit
{
HEARTS (2),
SPADES (3),
CLUBS (1),
DIAMONDS (0);
private int mSuitValue;
private SuitValue (int value)
{
mSuitValue = value;
}
public int getSuitValue() {
return mSuitValue;
}
}
With some additional code, like that on the Oracle site, we can generate a deck of cards and be able to rank them and know the value of a TWO of DIAMONDS
in comparison to a JACK of CLUBS
.
Hopefully that helped some, but please post back if you still have questions.
Happy coding,
Ken

poltexious
Courses Plus Student 6,993 PointsSPOILER! The solution will be provided below!
First, though, I have a question regarding the constructor. Why is it that the public keyword should not be entered before the constructor name? In my solution I wrote for the constructor:
// Constructor
public Brand(String displayName) {
mDisplayName = displayName;
}
instead of:
package com.example.model;
public enum Brand {
// Your code here
JVC("JVC"),
SONY("Sony"),
COBY("Coby"),
APPLE("Apple");
private String mDisplayName;
// Constructor
Brand(String displayName) {
mDisplayName = displayName;
}
// Getter
public String getDisplayName(){
return mDisplayName;
}
}
After watching the video again, I saw that Craig did not do it, so I tried it, and it worked.

Rylan Hilman
3,618 PointsI got stuck on the second step of this challenge for a bit too, and had to rewatch the video and glance at Ken's example to figure it out. The problem was I'm used to thinking of enum in an oldschool C way, where it's only there to be a custom data type that other things can use and reference to enhance code readability, not to be a class with its own internal variables and methods. Figuring out that it can also use Strings here and not just be a placeholder for (0, 1, 2, 3...) also helped too.

Amanda Lam
2,178 PointsHi, I am confused by enums too and have a question about ken's post. He used the enum to define the values of the cards. But then, how is it used? Like there is a method to set the value of the card by entering the value, but I don't get how the first part where it defined card values is used for the rest.

Ken Alger
Treehouse TeacherAmanda;
Let's look at some additional code that may help. Thus far we have values defined in our enums for card ranks and suits, so let's define a card:
public class Card
{
private Suit mSuit;
private CardValue mCardValue;
public Card (CardValue cardValue, Suit suit)
{
mCardValue = cardValue;
mSuit = suit;
}
public Suit getSuit()
{
return mSuit;
}
public void setSuit(Suit suit)
{
mSuit = suit;
}
public CardValue getCardValue()
{
return mCardValue;
}
public void setCardValue(CardValue cardValue)
{
mCardValue = cardValue;
}
}
Great! Now we can generate a deck...
import java.util.ArrayList;
import java.util.Collections;
import java.util.Iterator;
public class Deck
{
private ArrayList mDeck;
public Deck ()
{
mDeck = new ArrayList();
for (int i=0; i<13; i++)
{
CardValue value = CardValue.values()[i];
for (int j=0; j<4; j++)
{
Card card = new Card(value, Suit.values()[j]);
mDeck.add(card);
}
}
Collections.shuffle(deck);
Iterator cardIterator = deck.iterator();
while (cardIterator.hasNext())
{
Card aCard = cardIterator.next();
System.out.println(aCard.getCardValue() + " of " + aCard.getSuit());
}
}
}
That last println
statement will print out the generated deck. Since we have defined the values in the enums, we can compare the values of individual cards and their suits using the respective getValue
methods.
Make any sense?
Happy coding,
Ken

Craig Dennis
Treehouse TeacherStill stuck Cameron? Or did you pull through?
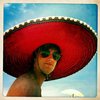
Cameron Raw
15,473 PointsOh hey guys. Sorry, I meant to reply to this! Yes, that helped a lot - thanks Ken. Great job on the videos by the way, Java seems like a pretty difficult topic to teach but after watching the videos over a few times and trying to apply it to my own mini projects, I usually understand what's happening!
Thanks again.