Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial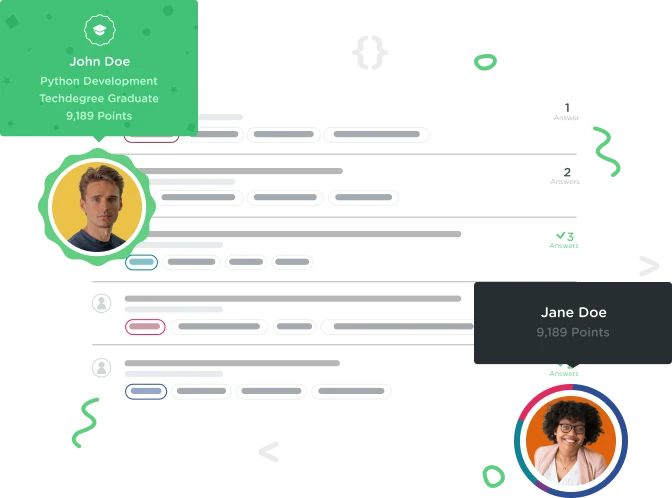
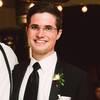
Zachary Luke
Front End Web Development Techdegree Graduate 17,646 PointsThis event listener works in preview, but still comes out wrong?
In this question, the objective is:
"Currently, the event listener applies a yellow background color to the section element and its child elements when clicked. Add a condition that changes the background of the <input> elements only."
When I check the preview window, the code works just fine. But when I click "Check Work", it still comes back incorrect, stating:
"Make sure you are filtering out all but the "INPUT" tags."
What am I missing here?
let section = document.getElementsByTagName('section')[0];
section.addEventListener('click', (e) => {
if (e.target.tagName == 'SECTION') {
e.target.style.backgroundColor = 'rgb(255, 255, 0)';
}
});
section.addEventListener('click', (e) => {
if (event.target.tagName == 'INPUT') {
event.target.style.backgroundColor = 'blue';
}
});
<!DOCTYPE html>
<html>
<head>
<title>JavaScript and the DOM</title>
</head>
<link rel="stylesheet" href="style.css" />
<body>
<section>
<h1>Making a Webpage Interactive</h1>
<p>JavaScript is an exciting language that you can use to power web servers, create desktop programs, and even control robots. But JavaScript got its start in the browser way back in 1995.</p>
<hr>
<p>Things to Learn</p>
<ul>
<li>Item One: <input type="text"></li>
<li>Item Two: <input type="text"></li>
<li>Item Three: <input type="text"></li>
<li>Item Four: <input type="text"></li>
</ul>
<button>Save</button>
</section>
<script src="app.js"></script>
</body>
</html>
11 Answers

Daniel Baker
15,369 Points"Add a condition that changes the background of the <input> elements only."
let section = document.getElementsByTagName('section')[0];
section.addEventListener('click', (e) => {
if (event.target.tagName == 'INPUT') {
e.target.style.backgroundColor = 'rgb(255, 255, 0)';
}
});
It is asking you to add the conditional to the current event. Change the current even.

Lynn Collins
10,080 PointsI'm fed up! I'm not doing this challenge anymore.

Daniel Medley
5,948 PointsDont feel bad, this course is falling apart towards the end.

sai meghanath marri
5,476 PointsBelow Code worked for me:
let section = document.getElementsByTagName('section')[0];
section.addEventListener('click', (e) => { if(e.target.tagName == 'INPUT'){ e.target.style.backgroundColor = 'rgb(255, 255, 0)'; } });

Lynn Collins
10,080 PointsI'm losing it...hate these challenges
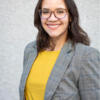
Katiuska Alicea de Leon
10,341 PointsI would get rid of the '[0]' in the variable declaration.
I did that and I still don't get the input fields to turn yellow.... nothing turns yellow ¬_¬
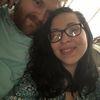
christopher abjornson
5,572 Pointsi used this but it still does not pass.
let section = document.getElementsByTagName('input');
for (var i = 0; i < section.length; i += 1) {
section[i].addEventListener('click', (e) => {
e.target.style.backgroundColor = 'rgb(255, 255, 0)';
});
}
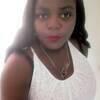
Yeukai Patience Shiripinda
8,067 Pointsi know this is so late but my guess is that your error was that you were using a for loop instead of a conditional statement.I could be wrong but i believe that that was the case .

Christian Clarke
7,963 PointsHi:
This is old but it looks like your second block of code is unnecessary and that's what's driving the confusion. If you change the code in the first block to == 'INPUT' instead of == 'SECTION' you should pass just fine!

Robert O'Toole
6,366 Pointsi think there is a bug in this problem. i wrote exactly what Daniel wrote and it wouldn't work but then when i copy and pasted his code in to the answer it worked? bizarre
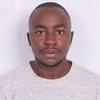
Ndhokoyo Aaron
6,589 Pointslet section = document.getElementsByTagName('section')[0];
section.addEventListener('click', (e) => { if(e.target.tagName == 'INPUT'){ e.target.style.backgroundColor = 'rgb(255, 255, 0)'; } });

Steven Steady
Front End Web Development Techdegree Student 8,658 PointsI was able to solve the challenge, but my question is why must the index ' [0] ' be included...that was were I was having an issue for it to work, I had deleted it initially because that is what Guil showed in the video lesson. Could someone explain that to me please?

Michal Czopek
15,394 PointsI am not sure but You have used e and event. I think they have to be the same. Regards Mike

Brett Bodofsky
2,102 PointsCan anybody confirm this? I used Daniels code and it worked fine. But didn't notice a difference when it came to the preview. Wish the preview didn't go away so fast after getting something correct. It should be the users choice whether to continue or not after getting an answer correct.

Daniel Baker
15,369 PointsBrett, I saw the same thing, it was actually your code I was using. I would have change it to e.target.tagName myself except it worked with out changing it. So, I wasn't going to correct you.
Vahe Gharagiozyan
10,088 PointsVahe Gharagiozyan
10,088 PointsThis works, thank you Daniel.