Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial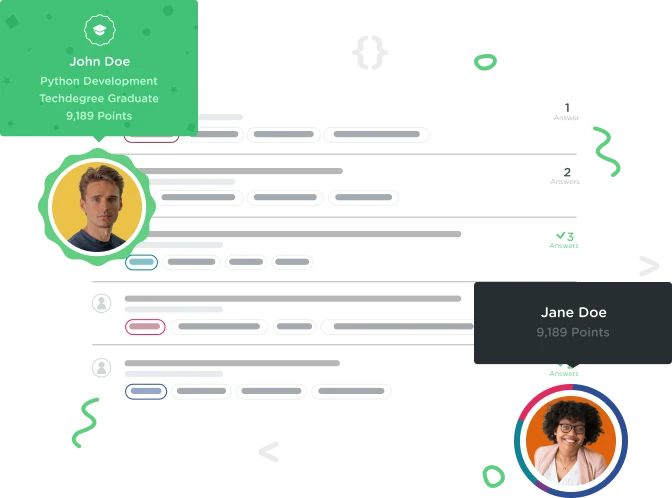

Pedro Meio-Dia
3,896 PointsThis function should remove all of the vowels ("a", "e", "i", "o", and "u") from the "word"(function's parameter). True?
def disemvowel(word): w = word vl = ['a','e','i','o','u'] vu = ['A','E','I','O','U'] lw = list(w) def new_word(): for r in lw: if r in vl or r in vu: now = True lw.remove(r) new_word() elif r not in vl or r not in vu: now = False new_word() novowels = ''.join(lw) print(novowels) disemvowel(word)
def disemvowel(word):
w = word
vl = ['a','e','i','o','u']
vu = ['A','E','I','O','U']
lw = list(w)
def new_word():
for r in lw:
if r in vl or r in vu:
now = True
lw.remove(r)
new_word()
elif r not in vl or r not in vu:
now = False
new_word()
novowels = ''.join(lw)
print(novowels)
disemvowel(word)
3 Answers

Antonio De Rose
20,885 PointsWhilst I agree with Steven, that your approach is correct, I'd still try to keep the code simple, the need of 2 functions is redundant, as you could do it in 1 function checking the vowels in a list, make it one list, true false checking not necessary and the lines of code, becomes very less, and the code becomes efficient
def disemvowel(word):
word = list(word)
lis = ['a','e','i','o','u','A','E','I','O','U']
li = []
for w in word:
if w not in lis:
li.append(w)
word = ''.join(li)
return word
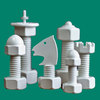
Steven Parker
231,269 PointsYou have the right idea, but the function should return the modified word
It doesn't need to "print" anything, and you don't need to call it yourself (the validator will do that).
Also, while it works, it's not a normal programming practice to define a function ("new_word") that is used only once. Also, the variable "now" is assigned (twice) but never used.
I'll bet you can get it now without an explicit code spoiler.

Pedro Meio-Dia
3,896 PointsOutstanding answer! I got it. Thank you very very much!
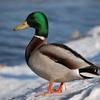
Robert Schaap
19,836 PointsOr something like this, using the try-except method Kenneth mentioned:
def disemvowel(word):
word = list(word)
vowels = ["a","e","i","o","u","A","E","I","O","U"]
for vowel in vowels:
while vowel in word:
try:
word.remove(vowel)
except ValueError:
pass
return "".join(word)
Pedro Meio-Dia
3,896 PointsPedro Meio-Dia
3,896 PointsTruly amazing! Simplicity and DRY code is key. I hope that I will obtain these skills quicker.
Blessings, my friend, and thank you for your help!
Take care!