Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial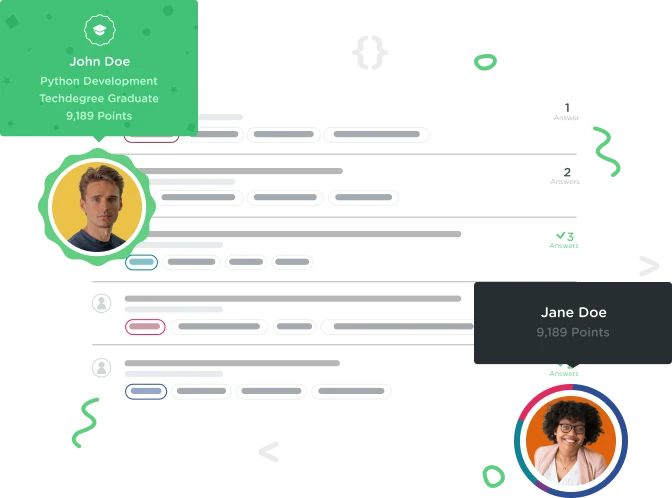

Ramy Elsaraf
7,633 PointsThis I cant solve
Oh right...we need a super-powered energy source before our time travel app will work! I've added one as an object in MainActivity. Fortunately it implements the Parcelable interface! Add it as another extra to the Intent in the onClick() method using the key "EXTRA_ENERGY".
import android.os.Bundle; import android.view.View; import android.widget.Button; import android.widget.EditText;
public class MainActivity extends Activity {
public Button goButton; public EditText yearField;
@Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main);
goButton = (Button)findViewById(R.id.goButton);
yearField = (EditText)findViewById(R.id.yearField);
final EnergySource source = new EnergySource();
goButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// Add your code in here!
Intent intent = new Intent(MainActivity.this, TimeTravelActivity.class);
intent.putExtra("EXTRA_YEAR", yearField.getText().toString());
startActivity(intent);
}
});
} }
2 Answers

Darnell Cephus
4,208 PointsBOOM! Got it!
public class MainActivity extends Activity {
public Button goButton;
public EditText yearField;
public static final String EXTRA_ENERGY = "EXTRA_ENERGY";
@Override protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
goButton = (Button)findViewById(R.id.goButton);
yearField = (EditText)findViewById(R.id.yearField);
final EnergySource source = new EnergySource();
goButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// Add your code in here!
Intent intent = new Intent(MainActivity.this, TimeTravelActivity.class);
intent.putExtra("EXTRA_YEAR", yearField.getText().toString());
intent.putExtra(EXTRA_ENERGY, source);
startActivity(intent);
}
});
} }

sean tatenda jack
6,127 Pointsit must be like this intent.putExtra("EXTRA_ENERGY", source);