Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial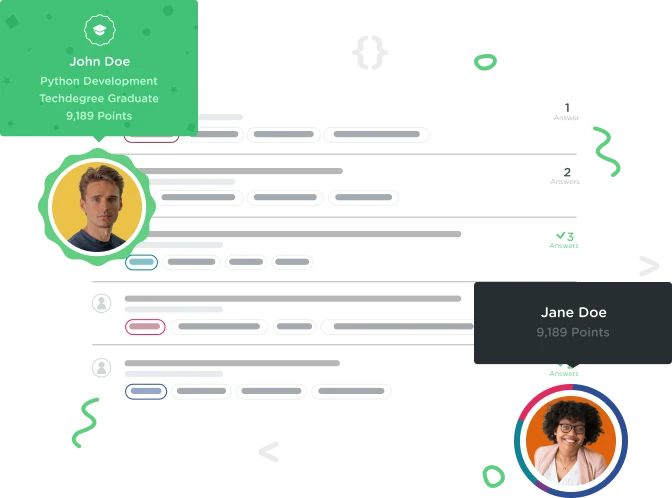
Gary Christie
5,151 PointsThis is a Javascript question that I'm learning from code academy. Help me please
Here are the instructions:
Print out the numbers from 1 - 20.
The rules:
For numbers divisible by 3, print out "Fizz".
For numbers divisible by 5, print out "Buzz".
For numbers divisible by both 3 and 5, print out "FizzBuzz" in the console.
Otherwise, just print out the number.
Why does this not work?
var numbers = [1,2,3,4,5,6,7,8,9,10,11,12,13,14,15,16,17,18,19,20]
for ( x=1; x < numbers.length; x++) {
switch(numbers) {
case ( numbers % 3):
console.log("Fizz");
break;
case (numbers % 5):
console.log("Buzz");
break;
case (numbers % 3 && numbers % 5):
console.log("FizzBuzz");
}
console.log(numbers);
}
3 Answers
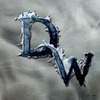
Hugo Paz
15,622 PointsHi Gary,
You cannot use a switch for this exercise. A switch evaluates a expression and depending on the result (case), executes a piece of code.
You can use something like this though:
var numbers = [1,2,3,4,5,6,7,8,9,10,11,12,13,14,15,16,17,18,19,20]
for ( x=0; x < numbers.length; x++) {
if( numbers[x] % 3 == 0 && numbers[x] % 5 == 0)
{
console.log('FizzBuzz ' + numbers[x]);
}else if( numbers[x] % 5 == 0){
console.log('Buzz ' + numbers[x]);
}else if ( numbers[x] % 3 == 0){
console.log('Fizz ' + numbers[x]);
}
}
Gary Christie
5,151 PointsI tired using this and it says "Oops, try again. It looks like you printed out the wrong number of items."
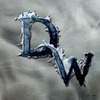
Hugo Paz
15,622 PointsThis is just a guide for your exercise, it is not the full answer. Try it like this:
var numbers = [1,2,3,4,5,6,7,8,9,10,11,12,13,14,15,16,17,18,19,20]
for ( x=0; x < numbers.length; x++) {
if( numbers[x] % 3 == 0 && numbers[x] % 5 == 0)
{
console.log('FizzBuzz ');
}else if( numbers[x] % 5 == 0){
console.log('Buzz ');
}else if ( numbers[x] % 3 == 0){
console.log('Fizz ');
}
else{
console.log(numbers[x]);
}
}
Gary Christie
5,151 PointsOh I see I had to take out the + numbers[x]. Thank you so much. I was stuck on that for days. How did you get so good at Java Script? Seriously? Its been 3 months and I'm still struggling. How can I develope some serious skills?
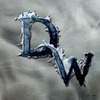
Hugo Paz
15,622 PointsLike most skills its all about repetition, I've been coding for years and there is still lots of things to learn. Just keep going.