Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial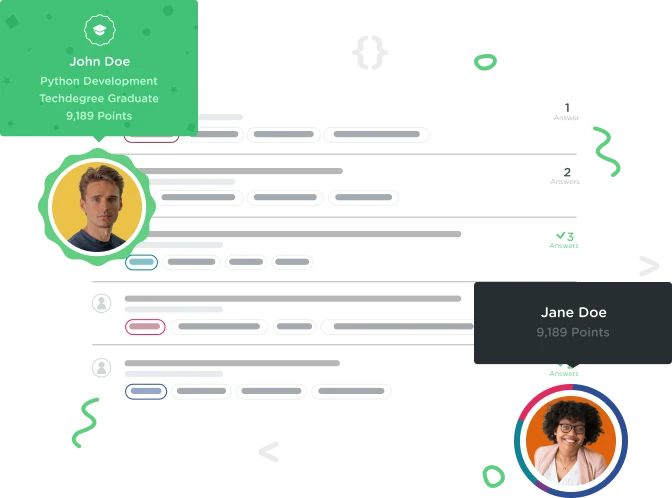

Damir Demirovic
Courses Plus Student 446 PointsThis is confusing, help please.
Okay, so let's use our new isFullyCharged helper method to change our implementation details of the charge method. Let's make it so it will only charge until the battery reports being fully charged. Let's use the ! symbol and a while loop. Inside the loop increment mBarsCount.
i already incremented mBarsCount but it gives error for some reason, why?
public class GoKart {
public static final int MAX_ENERGY_BARS = 8;
private String mColor;
private int mBarsCount;
public GoKart(String color) {
mColor = color;
mBarsCount = 0;
}
public String getColor() {
return mColor;
}
public void charge() {
mBarsCount = MAX_ENERGY_BARS;
}
public boolean isBatteryEmpty() {
return mBarsCount == 0;
}
public boolean isFullyCharged() {
while (mBarsCount != MAX_ENERGY_BARS)
mBarsCount++;
}
}
}
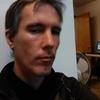
Mark Miller
45,831 PointsThe isFullyCharged should return a Boolean, so just tell it to return the answer to the test mBarsCount == MAX_ENERGY_BARS. Then use that method plus the while loop inside of the charge method. The instructions say to change the charge method so that it has the conditional happening with the charge. Your while loop can say while (!isFullyCharged()) inside of the charge method.
2 Answers
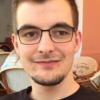
tobiaskrause
9,160 Pointspublic class GoKart {
public static final int MAX_ENERGY_BARS = 8;
private String mColor;
private int mBarsCount;
public GoKart(String color) {
mColor = color;
//sets it to 0
mBarsCount = 0;
}
public String getColor() {
return mColor;
}
public void charge() {
while(!isFullyCharged())
{
// adds +1 to mBarsCount so that the while-Loop will stop
mBarsCount++;
}
}
public boolean isBatteryEmpty() {
return mBarsCount == 0;
}
// Is wrong in the beginning since it gets set to 0
public boolean isFullyCharged() {
// mBarsCount is 0 and gets +1 since charge() adds +1 to mBarsCount as long
// as this method returns false
return mBarsCount == MAX_ENERGY_BARS;
}
}

Damir Demirovic
Courses Plus Student 446 PointsThis is error Tobias Krause
./GoKart.java:31: error: class, interface, or enum expected } ^ ./GoKart.java:26: error: incompatible types: int cannot be converted to boolean return mBarsCount; ^ 2 errors
tobiaskrause
9,160 Pointstobiaskrause
9,160 PointsPlease post the error message... "it gives error for some reason" is not rly helpfull.
I think that you need a return statement to return mBarsCount