Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial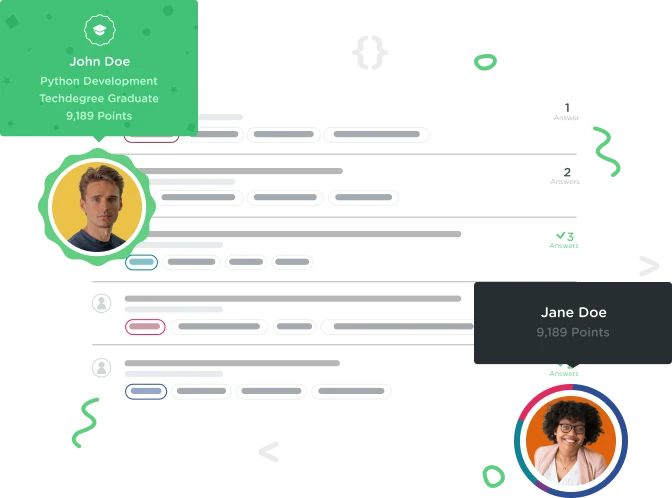
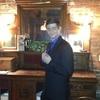
Carl Smith
8,185 PointsThis is confusing to me
Not sure how to perform this. Would I create my own "if let" and then try to pass it into leadActor? Is that possible? Or is there a simple solution?
let movieDictionary = ["Spectre": ["cast": ["Daniel Craig", "Christoph Waltz", "Léa Seydoux", "Ralph Fiennes", "Monica Bellucci", "Naomie Harris"]]]
var leadActor: String = ""
// Enter code below
if let movieStar = movieDictionary["cast"],
let leadStar = leadActor["Daniel Craig"] {
print(leadActor)
}
1 Answer
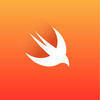
Steven Deutsch
21,046 PointsHey Carl Smith,
So what you're dealing with here is a dictionary of type [String: [String : [String]]]. That looks a bit intimidating writing it this way but lets break it apart.
This means you have a dictionary with a string as the key and another dictionary as a value. That value is a dictionary where the key is a string and the value is an array of strings.
What we need to do is get inside of that array of strings, and get the first element from the array, which is the lead actor. Since we will be extracting information from the dictionary, this will be a good situation to use optional binding. The reason for this being is when you return an element from a dictionary using a key, the value returned will always be wrapped in a optional. This is because it is possible for you to lookup a value using an invalid key and the query for that key to fail.
Now on to actually solving the problem. We have to access the value for the first key of the dictionary. This key is "Spectre", so using optional binding we extract the value.
if let movie = movieDictionary["Spectre"] {
/* Now the dictionary of ["cast": ["Daniel Craig", "Christoph Waltz",
"Léa Seydoux", "Ralph Fiennes", "Monica Bellucci", "Naomie Harris"]]
will be assigned to movie */
}
However, this is only the first part of the solution, now we need to go another level deeper: We now need to extract the value for the key "cast". We will use an optional binding chain so that we don't have to do nested if lets.
if let movie = movieDictionary["Spectre"], let cast = movie["cast"] {
/* Here if the assignment to movie succeeds, we will use it to extract the value for "cast"
Now the array of actors will be assigned to the constant cast */
}
Now we just have to get the first item out of that array and assign it to the leadActor variable. You can do this using subscript syntax:
if let movie = movieDictionary["Spectre"], let cast = movie["cast"] {
leadActor = cast[0]
}
Good Luck
Carl Smith
8,185 PointsCarl Smith
8,185 PointsThank you for the break down, it really helped a lot!