Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial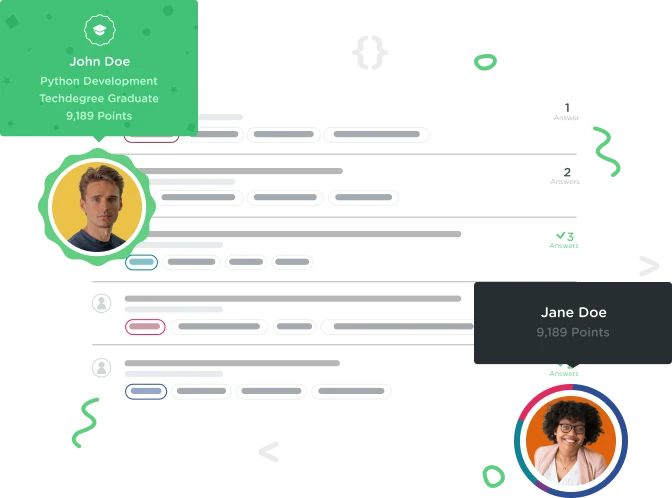
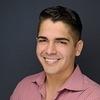
ian izaguirre
3,220 PointsThis is how I solved this challenge. I still have not looked at his answer, but I think I did not do what he expected?
Even though my program works, I do not think I solved it the way he expected.
I added the corresponding array number that matches each students name, so I do not think it is a flexible approach. Since it would not make sense to go through my approach if the list was really long.
Anyways, This is how I solved it:
var message = '';
var student;
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
function goFetch(foundName) {
for (var i = 0; i < 1; i += 1) {
student = students[foundName];
message += '<h2>Student: ' + student.name + '</h2>';
message += '<p>Track: ' + student.track + '</p>';
message += '<p>Points: ' + student.points + '</p>';
message += '<p>Achievements: ' + student.achievements + '</p>';
}
print(message);
alert('Close this prompt to view your results. To Search again, refresh your page');
}
do {
var response = prompt( 'Search student records: type a name [Jody] (or type "quit" to end)' );
var response = response.toLowerCase();
if ( response === 'dave' ) {
goFetch(0);
break;
} else if ( response === 'jody' ) {
goFetch(1);
break;
} else if ( response === 'jordan' ) {
goFetch(2);
break;
} else if ( response === 'john' ) {
goFetch(3);
break;
} else if ( response === 'trish' ) {
goFetch(4);
break;
} else if ( response === 'quit' ) {
break;
}
else {
alert('That is not the name of any current student. Please try again.');
}
} while ( response !== true );
I am going to try it again so it will be more flexible, but thought I would post this approach to see any feedback.
2 Answers

Rhys Kearns
4,976 PointsCould you do something like
var nameArray = ["dave", "jody", "jordan", "john", "trish"];
var indexNum = nameArray.indexOf(response);
if (indexNum != -1) {
goFetch(indexNum);
break;
} else if (response == "quit" {
break;
} else {
alert('That is not the name of any current student. Please try again.');
}
Im not sure if this works myself Im still learning too :) Just thought Id try fix that code up :D

Rhys Kearns
4,976 PointsAlso is there any reason for the for loop in the goFetch function as you seem to only plan to call it once and you aren't exactly looping through.
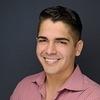
ian izaguirre
3,220 PointsYes the loop, loops too a separate file that was included in the challenge but I never pasted it here. The loop is connected to this array of objects:
var students = [
{
name: 'Dave',
track: 'Front End Development',
achievements: 158,
points: 14730
},
{
name: 'Jody',
track: 'iOS Development with Swift',
achievements: '175',
points: '16375'
},
{
name: 'Jordan',
track: 'PHP Development',
achievements: '55',
points: '2025'
},
{
name: 'John',
track: 'Learn WordPress',
achievements: '40',
points: '1950'
},
{
name: 'Trish',
track: 'Rails Development',
achievements: '5',
points: '350'
}
];
ian izaguirre
3,220 Pointsian izaguirre
3,220 PointsThanks for the input. Just to give a final update , I did it like this: