Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial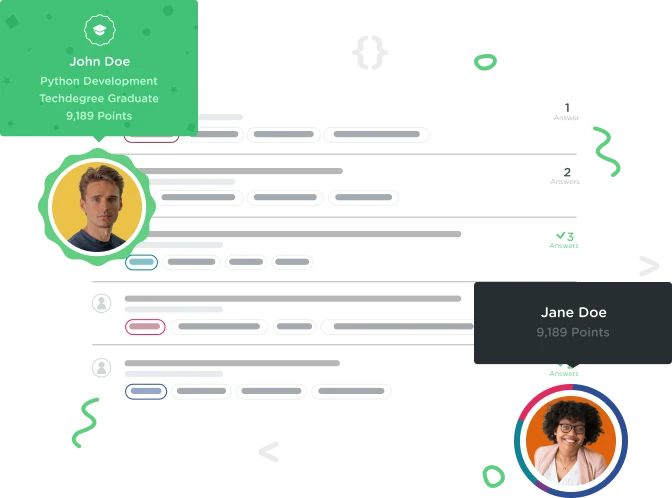

Vedang Patel
7,114 PointsThis is my articles exercise. This is what you made after I learnt Python Basics.
There might be some mistakes though I might have written something I learnt from Python collections because I am learning this right now.
#import
import os
#code
try:
#variables
passage_letters = ["a", "b", "c", "d", "e", "f", "g"]
#functions
def correct():
print("You got it right!")
def answers_and_corrections(letter, passage_letters):
answer = input("What is The answer for "+letter+": ").lower()
if answer == "a" or answer == "an" or answer == "the" or answer=="":
if answer == "an" and letter == "a":
correct()
elif answer == "a" and letter == "b":
correct()
elif answer == "" and letter == "c":
correct()
elif answer == "the" and letter == "d":
correct()
elif answer == "the" and letter == "e":
correct()
elif answer == "" and letter == "f":
correct()
elif answer == "a" and letter == "g":
correct()
else:
print("Sorry, wrong answer:(")
elif answer == "quit":
game_over()
elif answer == "help":
help()
answers_and_corrections(letter, passage_letters)
elif answer == "answers":
answer()
elif answer == "about":
clear()
print("Creator: Vedang Patel")
print("Editting help: Evan Demaris")
print("Inspired and tutored by: Kenneth Love")
else:
print("Invalid input")
answers_and_corrections(letter, passage_letters)
def clear():
if os.name == 'nt':
os.system('cls')
else:
os.system('clear')
def double_space():
print(" ")
print(" ")
def game_over():
print("Press enter to quit or you can type anything or take notes over here. Type 'reset' to try again.")
taken_input = input(" ").lower()
if taken_input == 'reset':
code(passage_letters)
clear()
def help():
clear()
print("Type 'quit' to close the exercise.")
print("Type 'help' to get this message and the questions.")
print("Type 'about' to get information about the app.")
print("Type 'reset' to reset")
print("Type 'answers' for the answers")
double_space()
print("My mother is a)_______ English teacher. I am b)______ student. When I get home from school, I watch c)_______ programs on TV. That's d)______ best part of my day. e)_________ programs I watch are for f)_______ children. I am g)______ child, so I think they are funny.")
double_space()
def answers():
clear()
print("Correct answers")
double_space()
print("My mother is an English teacher. I am a student. When I get home from school, I watch programs on TV. That's the best part of my day. The programs I watch are for children. I am a child, so I think they are funny.")
game_over()
def code(passage_letters):
help()
for letter in passage_letters:
double_space()
answers_and_corrections(letter, passage_letters)
answer()
game_over()
# code
code(passage_letters)
except ValueError:
print("The app has crashed due to wrong input!")
game_over()

Vedang Patel
7,114 PointsI've filtered some of my code. ;-)
1 Answer
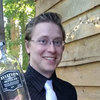
Evan Demaris
64,262 PointsHi Vedang,
Looks like you've got a pretty good grasp of Python.
In terms of suggestions for your code;
1) You have a large elif block to check what's essentially a dictionary of answers. I'd recommend refactoring the answers_and_corrections
function to use a for
loop to check the provided answer against a dictionary with the key being the passage letter.
2) Using a dictionary will allow you to more easily address another suggestion, which is to have the sentence used be one from several that is either randomly selected or selected by the user. Right now you've got the sentence hardcoded, but without switching away from that elif block it would be cumbersome to do anything else.
3) You may want to simplify your passage letters by including only the letter itself, not the parenthesis, and only including the parenthesis when printing to the console.
4) Your except
block catches all exceptions, best practice is to catch specific exceptions first before doing so in order to give the user as much information as possible.
5) You can chain the .lower() function to the value currently in unlowered_answer
and get rid of that variable altogether.
6) General console game mechanics; the game doesn't have any explanation of what its goal is, the user has no way to reset, quit, or find author/general info inside the game, and the user can't move past a wrong answer or be given the answer, which could be very frustrating for a grammar based game because correct English grammar is often debated.
Hope that helps!

Vedang Patel
7,114 PointsThanks Evan !!!!!
I will try to refactor the code to make it more efficient as a code and a good app for the users. But I have questions and replies for all your tips don't take them seriously if somehow its not polite.
- Its complicated could you tell me how to do it (though I know how to use dictionaries as I'm not good at.)
- Its complicated could you tell me how to do it (though I know how to use dictionaries as I'm not good at.)
- I didn't understand this one.
- I thought that it would be a good idea as I might have left out an exception and I don't want the people to get a big scary message.
- I want to know how to actually put in code.
- I copied this from Internet so I didn't think about debated grammar and of course I actually forgot to put more user interface.
Thanks for checking my code and taking your time to help me refactor the code
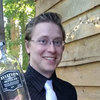
Evan Demaris
64,262 Points1) This course will teach how to work with dictionaries in Python
2) This course will teach how to read data from a file line by line, so you could use a text file to read sentences that you've pre-formatted. This not only makes it easy for you to add sentences, but it would allow a user to add their own sentences without knowing Python. You have a LOT of flexibility on how you do this, and no one way is going to be best. As an example though, you could have lines reflect:
This sentence _is_ meant to be an _example_ of an easy way to _flag_ specific words
When you parse the file with Python, you could use a regular expression to add the words surrounded by underscores to a dictionary and replace them with a)
, b)
, c)
... et cetera
3) If you use a
b
c
for passage letters instead of a)
b)
c)
it makes for easier to read code, at least to me personally, and having self-documenting code is important. You can leave the parenthesis when you write output for the user, so passage_letter + ")"
.
4) It is definitely a good idea to catch exceptions, but you want to catch specific exceptions before catching all of them. If your app crashes because of a ValueError
, the user should know that that's what caused the problem.
5) I'm not certain what you're asking on this one.

Vedang Patel
7,114 PointsI've got the 5th one so don't worry about it. I've edited the 4th one and the 3rd one to make it look neater. I will change the 2nd one and the 1st one after I complete the course. I will also edit the 6th one soon.
Vedang Patel
7,114 PointsVedang Patel
7,114 PointsAnd I forgot I want some feedback on how I can improve, change or filter my code.