Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial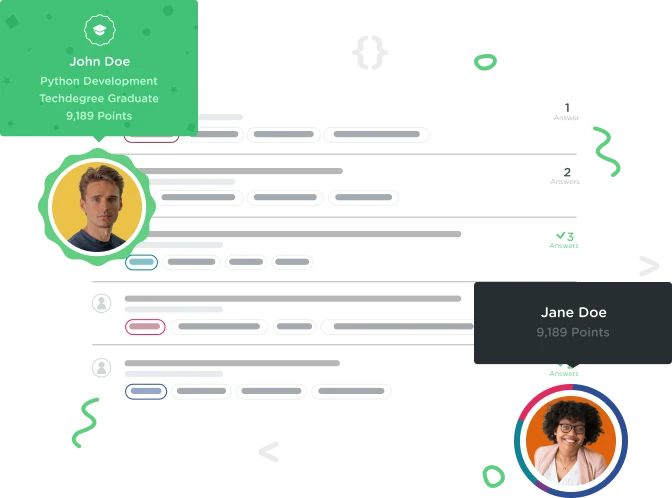

Michele Adami
5,691 PointsThis is my version... a little more complicated
The main difference from the original is that i used an array as a data type in the track property. I also used the for in loop to print out the properties' value.
var students = [
{
name: 'Michele',
tracks: ['HTML', 'CSS', 'JavaScript'],
achievment: 56,
points: 3000
},
{
name: 'Orazio',
tracks: ['PHP', 'Ruby', 'Developer Tools', 'Java'],
achievment: 275,
points: 14562
},
{
name: 'Natalia',
tracks: ['Design', 'Business'],
achievment: 12,
points: 1206
},
{
name: 'Pietro',
tracks: ['HTML', 'CSS', 'JavaScript', 'PHP', 'Python', 'Perl', 'Ruby', 'Java', 'Game Development', 'Developer Tools'],
achievment: 2752,
points: 123763
},
{
name: 'Gianni',
tracks: ['HTML', 'CSS'],
achievment: 5,
points: 576
}
];
var thing = document.getElementById('output');
for(var i = 0; i < students.length; i++){
thing.innerHTML += '<h2>' + students[i]['name'] + '</h2><ol>';
for(var prop in students[i]){
if(students[i][prop] === students[i]['name']) continue;
if(typeof students[i][prop] === 'object') students[i][prop] = students[i][prop].join(', ');
thing.innerHTML+= '<li>' + students[i][prop] + '</li>';
}
thing.innerHTML+='</ol>';
}

Michele Adami
5,691 PointsThank you for the advice about the typeof, i didn't know about the existence of the isArray method.
if(students[i][prop] === students[i]['name']) continue; Why not remove this line?
Because I want that the name property of the objects should be printed out in a h2 tag and not in a li tag like the other properties. So i told the for in loop to bypass that property.

Gunhoo Yoon
5,027 PointsOh I see great I missed that part.
Gunhoo Yoon
5,027 PointsGunhoo Yoon
5,027 Pointsif(students[i][prop] === students[i]['name']) continue;
Why not remove this line?typeof students[i][prop] === 'object'
is considered not so good practice since null, array-like object and object is considered type of object. If you want to check for an array use Array.isArray(), isinstanceof, tostring etc... You can find more on Google with keyword: javascript array checking.