Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial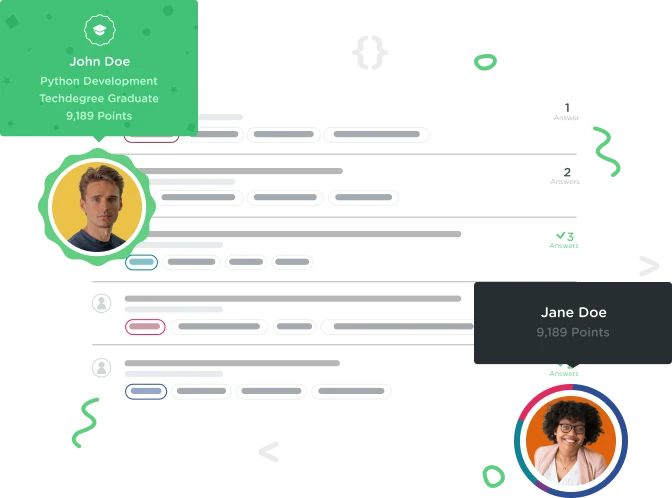
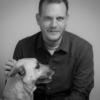
Tyler Dix
14,230 PointsThis is needlessly challenging.
I've spent several hours trying to solve this challenge on my own, and I actually got pretty close. After building this program piece-by-piece, I finally got it to print an ordered list and the number of correct responses. It's sloppy, poorly formatted, and contains several "undefined" messages, but it works.
I'm deflated after watching the solution, however. The instructor introduces a brand new concept, the document.getElementById (method?), stores this in a variable equal to the parameter, and then expects us to create empty arrays, push content to them, and then pass this content to a brand new function that loops through a brand new array? Seriously?
I'm all for a challenge, and I can Google as fast as anyone, but I think this is ridiculously challenging. Given what we know, I find it impossible to solve this challenge without reviewing the solution.
Let's review what we know already before introducing new concepts as you explain the solution please.
- A Frustrated Student
PS: The rest of the course was very good, and explained concepts in a clear and direct manner. I think this piece needs a bit of work though.
4 Answers
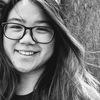
Rachel KTY Johnson
Treehouse Project ReviewerHey Tyler Dix , sorry you've having troubles with this challenge. It's not an easy challenge to get so I understand that you're getting frustrated. It's okay to feel this way, it's all a part of learning new things. If you're not challenged, you're not learning ;)
I've made some edits to your code to make it work. I've left comment notes throughout so you kinda get it.
var quizArray = [
["What is the name of earth's satellite? ", 'moon'],
["What color is grass? ", 'green'],
['German Shepherds are the best dogs: T/F ?', 'T']
];
let numCorrect = 0;
function print(message) {
document.write(message);
}
//this is to run the function itself.
quizFunction();
//the quiz function holding all logic
function quizFunction() {
let correctString = ""; //this is a string that will hold all the correct answers in LI tags.
for (let i = 0; i < quizArray.length; i++) {
var userPrompt = prompt(quizArray[i][0]);
if (userPrompt === quizArray[i][1]) {
numCorrect++;
let isRight = quizArray[i][0];
correctString += ("<li>" + isRight + "</li>"); //this adds the correct answer in LI tags to the string
} // add else statement here
} // ends for loop
//I've put your print functions in the function so it only runs once per function, AFTER all the logic has been done
print("You got " + numCorrect + " answers right." + "<br>");
print("<h3>" + "You got these questions correct:" + "</h3>");
print("<ol>" + correctString + "</ol>"); //this is where I put the string with all the correct answers in LI tags
} // ends function
The main thing causing the undefined was the fact that your print functions were outside of the game logic function. Things weren't changed before you printed them (especially the count of correct answers).
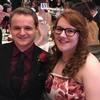
Alexander Besse
Full Stack JavaScript Techdegree Graduate 35,115 PointsHi again, Tyler Dix!
I took a look into your code.
The first glitch, where the numCorrect
only shows 0 on the site, this because quizFunction()
(Which adds to numCorrect
) doesn't get called until after the numCorrect
is printed on the page and at the time that numCorrect
is printed, it's equal to 0 because the function hasn't been called to increase it. Hope that makes sense, haha.
As for what undefined
keeps showing up, I'm not totally sure but I believe it has to do with your for loop and how information is being passed around among it. The reason I think this is because I did two print(quizFunction());
calls and so undefined
showed up twice. Hopefully that will give you somewhere to start.
Best of luck and let me know if you'd like more help!
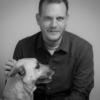
Tyler Dix
14,230 PointsOh boy, this is such a newbie mistake. Maybe I should actually call the function if I expect it to do anything! hahaha
Thanks for this, Alexander. I've pushed on to the next section about arrays and I somehow got it all right. For a moment there I thought this was a biological problem with my brain not creating new connections, but I suppose it's sticking.
Thanks for the review and update!
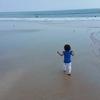
Richard Thomas
3,029 PointsIt's like they have no idea what the students have learned so far. It's laughable
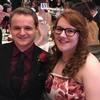
Alexander Besse
Full Stack JavaScript Techdegree Graduate 35,115 PointsHi, Tyler Dix!
I can spread some insight on how I did it. I'll post my solution below with a lot of comments, so that it walks you through the script step-by-step. Hopefully this helps!
Best wishes and let me know, happy coding!
// Creating an array of objects (list of objects).
// Question: is the question, obviously.
// Answer: is the correct answer,
// Given: is the answer given by user.
var qa = [
{
question: "What is the year I was born?",
answer: "1997",
given: "",
},
{
question: "What is my mother's first name?",
answer: "Jennifer",
given: "",
},
{
question: "What is my favorite color?",
answer: "Blue",
given: "",
},
{
question: "What is my favorite programming language?",
answer: "Javascript",
given: "",
},
];
// Array that will contain the answers that were correct
var correct = [];
// Array that will contain the answers that were not correct
var wrong = [];
// ==
// = Asking the Question
// ==
// This for loop is running through every Object inside of the "qa" array.
for(var i = 0; i < qa.length; i++){
// currentList is the current Object that is being looked at by the for loop.
var currentList = qa[i];
// Do While loops run at least once, and then run more if the condition is not met.
do{
// Prompt the user to answer the question, and assign it to the currentList's variable 'given'.
currentList.given = prompt(currentList.question);
// If the 'given' property is '' (blank), it will ask the question again.
}while(currentList.given == "");
}
// At this point in the code, all the questions are answered, and all the 'given' properties in the 'qa' array have values.. Now time to check if the answers are correct.
// Once again, this for loop is running through every Object inside of the "qa" array.
for(var i = 0; i < qa.length; i++){
// currentList is the current Object that is being looked at by the for loop.
var currentList = qa[i];
// Checking to see if the current object's answer property is equal to the given property.
// You can use .toUpperCase() or .toLowerCase() on BOTH properties to make the script not case sensitive. Like I did below.
if(currentList.answer.toUpperCase() == currentList.given.toUpperCase()){
// If the answer and the given are the same, add it to the 'correct' array.
correct.push(currentList);
}else{
// Else if the answer and the given are not the same, add it to the 'wrong' array.
wrong.push(currentList);
}
}
// Printing the amount of questions the user got correct
print("You got " + correct.length + " questions correct! <br><br>");
// Just some styling here by printing "Correct Answers:" as a title.
print("Correct answers: <br>");
// Run through all the correct answers, and print them to the page.
for(var i = 0; i < correct.length; i++){
print((i + 1) + ". " + correct[i].question + " - Answer: " + correct[i].answer + "<br>");
}
// More styling.
print("Incorrect answers: <br>");
// Run through all the wrong answers, and print them to the page.
for(var i = 0; i < wrong.length; i++){
print((i + 1) + ". " + wrong[i].question + " - Answer: " + wrong[i].answer + " - Your Answer: " + wrong[i].given + "<br>");
}
// Function to print the messages to the page.
function print(message) {
document.write(message);
}
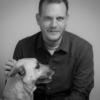
Tyler Dix
14,230 PointsIt worked! Great work here, Alexander, and thanks for sharing another possible solution to this challenge (particularly excluding the extra function).
At a certain point, I got an ordered list to print correctly, but now it's broken, as is my counter. It's running, so I can't find out what is going on. Here is my crack at the challenge:
var quizArray = [
["What is the name of earth's satellite? ", 'moon'],
["What color is grass? ", 'green'],
['German Shepherds are the best dogs: T/F ?', 'T']
];
function print(message) {
document.write(message);
}
var numCorrect = 0;
function quizFunction(quizParam) {
for (i = 0; i < quizArray.length; i += 1) {
var userPrompt = prompt(quizArray[i][0]);
if (userPrompt === quizArray[i][1]) {
numCorrect += 1;
var isRight = quizArray[i][0];
print("<li>" + isRight + "</li>");
} // add else statement here
} // ends for loop
} // ends function
print("You got " + numCorrect + " answers right." + "<br>");
print("<h3>" + "You got these questions correct:" + "</h3>");
print("<ol>" + quizFunction() + "</ol>");
// Note if you try to run this in your browser, I take no responsibility if it catches on fire.
I think I get the fundamental concepts that I'm expected to get, but manipulating the DOM with an ordered list is particularly tricky. And I have no idea why my counter isn't working...
I give up.
Tyler Dix
14,230 PointsTyler Dix
14,230 PointsThanks for the review, Rachael. I reviewed the edits and I think I get what I did wrong. I'm going to come back to this after I do more coursework and tinkering around on my own and try and solve it again. I appreciate you taking the time to review my code and explain where I went wrong.