Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial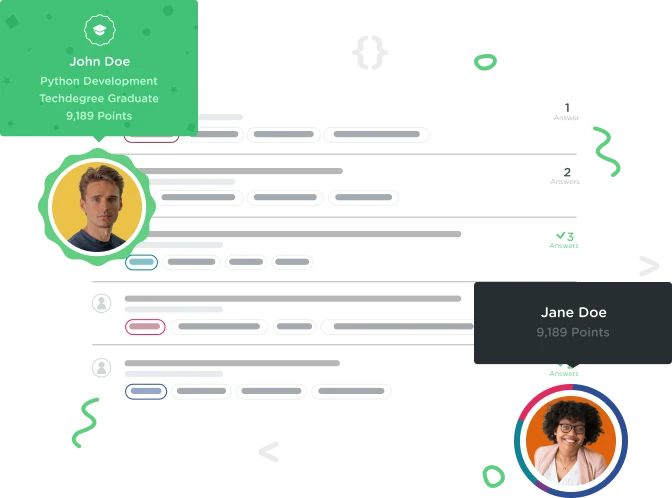
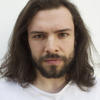
Sean Lafferty
3,029 PointsThis is nonsense. Been stuck for ages, please Help!
Hi Guys, really unsure of the syntax on this one. tried a thousand different ways, been stuck for 3 hours. Please can someone explain this problem to me at each part? cant even pass the first one! :(
enum ParserError: ErrorType {
case EmptyDictionary
case InvalidKey
}
struct Parser {
var data: [String : String?]?
func parse() throws {
guard let data = data else {
throw ParserError.EmptyDictionary
}
guard let key = data["somekey"] else {
throw ParserError.InvalidKey
}
}
}
let data: [String : String?]? = ["someKey": nil]
do {
let parser = try Parser(data: data)
try parser.parse()
} catch { }
4 Answers
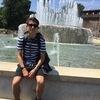
Mitch Little
11,870 PointsHi Sean,
For the first part of the code challenge you need to use two guard statements to unwrap and use two optionals. If these optionals contain nil, the error is thrown.
The guard statements should look like this:
func parse() throws {
guard let data = data else {
throw ParserError.emptyDictionary
}
guard data.keys.contains("someKey") else {
throw ParserError.invalidKey
}
}
Where the first statement throws the emptyDictionary error if the optional dictionary (data) returns nil. The second statement throws the invalidKey error if the optional key inside the optional dictionary returns nil.
In the second task, you are asked to add a generic do catch block.
do {
try parser.parse()
} catch let error {
print(error)
}
The do statement contains the method that may throw an error, and the catch statement prints the error constant if there is an error.
In the third task, you are asked to write a more specific do catch block.
do {
try parser.parse()
} catch ParserError.emptyDictionary {
print("You have an empty dictionary")
} catch ParserError.invalidKey {
print("You have an invalid key")
}
The catch statements in this block pattern match on any possible errors thrown from the parse method that we added in task 1.
I hope all of this helps.
Overall the solution should look like this:
enum ParserError: Error {
case emptyDictionary
case invalidKey
}
struct Parser {
var data: [String : String?]?
func parse() throws {
guard let someData = data else {
throw ParserError.emptyDictionary
}
guard someData.keys.contains("someKey") else { //the keys of the unwrapped dictionary can be checked
throw ParserError.invalidKey
}
}
}
let data: [String : String?]? = ["someKey": nil]
let parser = Parser(data: data)
do {
try parser.parse()
} catch ParserError.emptyDictionary {
print("You have an empty dictionary")
} catch ParserError.invalidKey {
print("You have an invalid key")
}
I hope all of this helps and you can make sense of this.
All the best,
Mitch
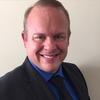
Eric Witowski
Courses Plus Student 92 Pointshow do I write code in the blackboard and then post it, does anyone here know?
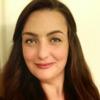
Jennifer Nordell
Treehouse TeacherHi Eric! You can look at the Markdown Cheatsheet at the bottom of the "Add an Answer" section for quick tips on how to do this. Alternatively, if you want to get really fancy with your markdown and have an hour to spare, you should definitely check out the Markdown Basics course here at Treehouse.
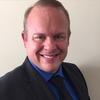
Eric Witowski
Courses Plus Student 92 Pointsseems like you need a switch I don't think case are on top of each other
for ______ in _____{
switch _____{
case _____where _____% 2 ==0:
print________________
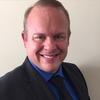
Eric Witowski
Courses Plus Student 92 Points//test code here
//end code here
Eric Witowski
Courses Plus Student 92 PointsEric Witowski
Courses Plus Student 92 PointsI see a question mark outside brackets, is that what you want?