Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial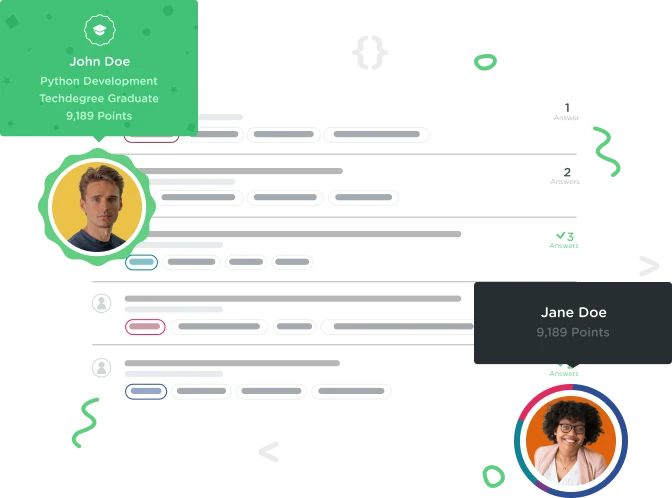
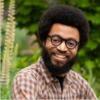
Nicolas Hampton
44,638 PointsThis Is The Droid You Are Looking For: How to get past this lesson while knowing the modern standard.
There's been a lot of questions regarding this lesson, and that's because of a recent update to the android API deprecating the tabs functions in API 21 as of November 2014. This is causing a lot of confusion. Clickable tabs have been replaced by something called PagerTabStrip that aren't clickable, they only use swipe to save space. Finish watching this video, using all the instructions in it, then compare your code to the code I leave as an answer below in the first half. I have devoted the second half of this answer to making the changes needed for the modern PagerTabStrip, which doesn't use thick clickable tabs that take up valuable space on the screen. I've also ran it on both 4.4.4 and 5.0.0, and both with the deprecated code in the lesson and the update I provide here, it runs. (Note: for limited tabs like menus, use FragmentPagerAdapter like we do here, for indeterminate lists like swiping through blog posts, use FragmentStatePagerAdapter. These are optimized for memory recovery for each task.)
6 Answers
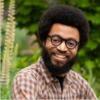
Nicolas Hampton
44,638 PointsFirst, I'll give you the working code before deprecation, so you know what it looked like before and what we'll need the change to update. At the end will be some comments on cleaning up the imports, a common problem people have been having in implementing the support libraries:
MainActivity working code before deprecation. I have marked the deprecated code with comments about what we'll change to update to the new standards and why. Those comments are surrounded by these asterisks. Everything else should be boilerplate comments android studio adds automatically :
package com.staggarlee.ribbit;
// ***These imports are a mess, and after trying everything to make this work***
// ***and auto-importing a lot of dependencies, yours probably are too. At ***
// ***the end of this, I'll go over cleaning this up to avoid any possible conflicts***
import java.util.Locale;
import android.content.Context;
import android.content.Intent;
import android.support.v4.app.FragmentActivity;
import android.support.v7.app.ActionBarActivity;
import android.support.v7.app.ActionBar;
import android.support.v4.app.Fragment;
import android.support.v4.app.FragmentManager;
import android.support.v4.app.FragmentTransaction;
import android.support.v4.app.FragmentPagerAdapter;
import android.os.Bundle;
import android.support.v4.view.ViewPager;
import android.support.v7.internal.widget.ActionBarContainer;
import android.util.Log;
import android.view.Gravity;
import android.view.LayoutInflater;
import android.view.Menu;
import android.view.MenuItem;
import android.view.View;
import android.view.ViewGroup;
import android.view.Window;
import android.widget.TextView;
import com.parse.ParseObject;
import com.parse.ParseUser;
// ***The TabListener has been deprecated, we don't need it anymore. ***
// ***Android has decided only to let us use the swipe function for tabs***
// ***to free up space, so the TabListener interface can be eliminated ***
// ***after update. ***
public class MainActivity extends ActionBarActivity implements ActionBar.TabListener {
public static final String TAG = MainActivity.class.getSimpleName();
/**
* The {@link android.support.v4.view.PagerAdapter} that will provide
* fragments for each of the sections. We use a
* {@link FragmentPagerAdapter} derivative, which will keep every
* loaded fragment in memory. If this becomes too memory intensive, it
* may be best to switch to a
* {@link android.support.v4.app.FragmentStatePagerAdapter}.
*/
SectionsPagerAdapter mSectionsPagerAdapter;
/**
* The {@link ViewPager} that will host the section contents.
*/
ViewPager mViewPager;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// Get the user cached on disk if present
ParseUser currentUser = ParseUser.getCurrentUser();
if(currentUser == null) {
// If no one is logged in, go to the login screen
navigateToLogin();
} else {
Log.i(TAG, currentUser.getUsername());
}
// Set up the action bar.
final ActionBar actionBar = getSupportActionBar();
// ***This is where setNavigationMode has been deprecated***
// ***Here, we're telling the action bar that we want the old ***
// ***deprecated, clickable tabs. ViewPager doesn't need ***
// ***them to operate, it can handle non-clickable, swipable ***
// ***tabs on it's own, so we're going to cut it, thus giving us***
// *** the thinner, non-clickable tabs only. ***
actionBar.setNavigationMode(ActionBar.NAVIGATION_MODE_TABS);
// Create the adapter that will return a fragment for each of the two
// primary sections of the activity. Remember to add the context of 'this'
// to the constructor, just like the video, since we factor out the
// SectionsPagerAdapter to a separate class.
mSectionsPagerAdapter = new SectionsPagerAdapter(this, getSupportFragmentManager());
// Set up the ViewPager with the sections adapter.
mViewPager = (ViewPager) findViewById(R.id.pager);
mViewPager.setAdapter(mSectionsPagerAdapter);
//***The next section is the OnPageChangeListener. This operates***
//***not to listen for the swipe, but to listen for when you tap a ***
//***tab, like a button. This is what google decided to eliminate in ***
//***order to save space and be similar to iOS, so we'll remove the***
//***entire deprecated method after the update. Though ***
//***OnPageChange Listener has not been deprecated, everything***
//*** that it's operating on, the tab clicks, has been, so we don't ***
//***need it. ***
mViewPager.setOnPageChangeListener(new ViewPager.SimpleOnPageChangeListener() {
@Override
public void onPageSelected(int position) {
// ***Again, setSelectedNavigationItem is deprecated***
actionBar.setSelectedNavigationItem(position);
}
});
// For each of the sections in the app, add a tab to the action bar.
for (int i = 0; i < mSectionsPagerAdapter.getCount(); i++) {
// Create a tab with text corresponding to the page title defined by
// the adapter. Also specify this Activity object, which implements
// the TabListener interface, as the callback (listener) for when
// this tab is selected.
// ***addTab has been deprecated***
actionBar.addTab(
actionBar.newTab()
.setText(mSectionsPagerAdapter.getPageTitle(i))
.setTabListener(this));
}
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.menu_main, menu);
return true;
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
// Handle action bar item clicks here. The action bar will
// automatically handle clicks on the Home/Up button, so long
// as you specify a parent activity in AndroidManifest.xml.
int id = item.getItemId();
//noinspection SimplifiableIfStatement
switch (id) {
case R.id.action_logout:
ParseUser.logOut();
navigateToLogin();
break;
}
return super.onOptionsItemSelected(item);
}
private void navigateToLogin() {
Intent intent = new Intent(MainActivity.this, LoginActivity.class);
intent.addFlags(Intent.FLAG_ACTIVITY_NEW_TASK);
intent.addFlags(Intent.FLAG_ACTIVITY_CLEAR_TASK);
startActivity(intent);
}
// ***These three tabs have deprecated tabs as arguments***
// ***They're handling what happens when that large tabs ***
// ***are selected and unselected. The new PagerTabStrip ***
// ***doesn't have tabs to select like that, so we'll remove ***
// ***these in the update. ***
@Override
public void onTabSelected(ActionBar.Tab tab, FragmentTransaction fragmentTransaction) {
// When the given tab is selected, switch to the corresponding page in
// the ViewPager.
mViewPager.setCurrentItem(tab.getPosition());
}
@Override
public void onTabUnselected(ActionBar.Tab tab, FragmentTransaction fragmentTransaction) {
}
@Override
public void onTabReselected(ActionBar.Tab tab, FragmentTransaction fragmentTransaction) {
}
/**
* A placeholder fragment containing a simple view.
*** This is referred to as "DummySectionFragment" in the video.
*** If you have the up-to-date android studio, this is the code that appears.
*** You need not change anything here, this is all non-deprecated code.
*/
public static class PlaceholderFragment extends Fragment {
/**
* The fragment argument representing the section number for this
* fragment.
*/
private static final String ARG_SECTION_NUMBER = "section_number";
/**
* Returns a new instance of this fragment for the given section
* number.
*/
public static PlaceholderFragment newInstance(int sectionNumber) {
PlaceholderFragment fragment = new PlaceholderFragment();
Bundle args = new Bundle();
args.putInt(ARG_SECTION_NUMBER, sectionNumber);
fragment.setArguments(args);
return fragment;
}
public PlaceholderFragment() {
}
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
View rootView = inflater.inflate(R.layout.fragment_main, container, false);
return rootView;
}
}
}
SectionsPagerAdapter working code before deprecation. Nothing to change here after the deprecation, we will use this exact code from video with the new standard, PagerTabStrip :
package com.staggarlee.ribbit;
/**
* Created by nicolas on 5/9/15.
*/
import android.content.Context;
import android.support.v4.app.Fragment;
import android.support.v4.app.FragmentManager;
import android.support.v4.app.FragmentPagerAdapter;
import java.util.Locale;
/**
* A {@link FragmentPagerAdapter} that returns a fragment corresponding to
* one of the sections/tabs/pages.
*/
public class SectionsPagerAdapter extends FragmentPagerAdapter {
// In the lesson, we factor this code out of MainActivity.class, and thus must add the context
// as a member variable that gets passed in through the constructor. Nothing about this
// will change from the video, no deprecation involved, no Android Studio conflicts.
protected Context mContext;
public SectionsPagerAdapter(Context context, FragmentManager fm) {
super(fm);
mContext = context;
}
@Override
public Fragment getItem(int position) {
// getItem is called to instantiate the fragment for the given page.
// Return a PlaceholderFragment (defined as a static inner class below).
return MainActivity.PlaceholderFragment.newInstance(position + 1);
}
@Override
public int getCount() {
// In the video we reduce this boilerplate value from 3
// to 2 tabs, one for Inbox and one for Friends. Again, no
// change needed from the video, follow along.
// Show 2 total pages.
return 2;
}
@Override
public CharSequence getPageTitle(int position) {
Locale l = Locale.getDefault();
// this is where we'll pass the context to variables that are now
// outside the scope of MainActivity.class after the refactor.
// again, nothing different than the video, follow the video.
// Remember to delete the third case in the boilerplate code
// and change the strings resources entries to Inbox and
// Friends, respectively, just like in the video.
switch (position) {
case 0:
return mContext.getString(R.string.title_section1).toUpperCase(l);
case 1:
return mContext.getString(R.string.title_section2).toUpperCase(l);
}
return null;
}
}
These are the sources I used to find a solution for the deprecated Tabs. It requires that we remove the implementation of TabListener from main activity, remove the excess clickable tab code, and create a new childview of the ViewPager called PagerTabStrip in the MainActivity xml layout file.
http://developer.android.com/training/implementing-navigation/lateral.html#horizontal-paging
http://mobile.cs.fsu.edu/overcoming-the-action-bar-tabs-deprecation-in-api-21/
The MainActivity.class file and activity_main.xml file are the only ones we need to change to do this, so I'm only posting the source of those two files for reference. I'm leaving the action bar, as we're still using it for logout and other functions, we're just not attaching our tabs to it. In the future, if Ben talks about swiping tabs, those are changes we'll make to the code, if he talks about tapping a tab, we can ignore, as that has been deprecated.
MainActivity.class source code with clickable action bar tabs removed:
package com.staggarlee.ribbit;
import android.content.Intent;
import android.support.v7.app.ActionBarActivity;
import android.support.v7.app.ActionBar;
import android.support.v4.app.FragmentPagerAdapter;
import android.support.v4.view.ViewPager;
import android.os.Bundle;
import android.util.Log;
import android.view.Menu;
import android.view.MenuItem;
import com.parse.ParseUser;
// ***The TabListener has been deprecated, will look for update later***
// ***The MainActivity class is implementing TabListener, so the ***
// *** activity itself is listening for tab changes. We'll have to ***
// *** replace this later. Remember that TabListener is an interface,***
// *** so we'll be looking for a replacement interface later. ***
public class MainActivity extends ActionBarActivity {
public static final String TAG = MainActivity.class.getSimpleName();
/**
* The {@link android.support.v4.view.PagerAdapter} that will provide
* fragments for each of the sections. We use a
* {@link FragmentPagerAdapter} derivative, which will keep every
* loaded fragment in memory. If this becomes too memory intensive, it
* may be best to switch to a
* {@link android.support.v4.app.FragmentStatePagerAdapter}.
*/
SectionsPagerAdapter mSectionsPagerAdapter;
/**
* The {@link ViewPager} that will host the section contents.
*/
ViewPager mViewPager;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// Get the user cached on disk if present
ParseUser currentUser = ParseUser.getCurrentUser();
if (currentUser == null) {
// If no one is logged in, go to the login screen
navigateToLogin();
} else {
Log.i(TAG, currentUser.getUsername());
}
// Set up the action bar.
final ActionBar actionBar = getSupportActionBar();
// ***This is where setNavigationMode has been deprecated***
// Create the adapter that will return a fragment for each of the three
// primary sections of the activity.
mSectionsPagerAdapter = new SectionsPagerAdapter(this, getSupportFragmentManager());
// Set up the ViewPager with the sections adapter.
mViewPager = (ViewPager) findViewById(R.id.pager);
mViewPager.setAdapter(mSectionsPagerAdapter);
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.menu_main, menu);
return true;
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
// Handle action bar item clicks here. The action bar will
// automatically handle clicks on the Home/Up button, so long
// as you specify a parent activity in AndroidManifest.xml.
int id = item.getItemId();
//noinspection SimplifiableIfStatement
switch (id) {
case R.id.action_logout:
ParseUser.logOut();
navigateToLogin();
break;
}
return super.onOptionsItemSelected(item);
}
private void navigateToLogin() {
Intent intent = new Intent(MainActivity.this, LoginActivity.class);
intent.addFlags(Intent.FLAG_ACTIVITY_NEW_TASK);
intent.addFlags(Intent.FLAG_ACTIVITY_CLEAR_TASK);
startActivity(intent);
}
/**
* A placeholder fragment containing a simple view.
* This is referred to as "DummySectionFragment" in the
* lesson.
*/
public static class PlaceholderFragment extends Fragment {
/**
* The fragment argument representing the section number for this
* fragment.
*/
private static final String ARG_SECTION_NUMBER = "section_number";
public PlaceholderFragment() {
}
/**
* Returns a new instance of this fragment for the given section
* number.
*/
public static PlaceholderFragment newInstance(int sectionNumber) {
PlaceholderFragment fragment = new PlaceholderFragment();
Bundle args = new Bundle();
args.putInt(ARG_SECTION_NUMBER, sectionNumber);
fragment.setArguments(args);
return fragment;
}
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
View rootView = inflater.inflate(R.layout.fragment_main, container, false);
return rootView;
}
}
}
activity_main.xml layout file with PagerTabStrip added:
<android.support.v4.view.ViewPager android:id="@+id/pager"
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
// *** Here, we add our child view PagerTabStrip***
<android.support.v4.view.PagerTabStrip
android:id="@+id/pager_title_strip"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_gravity="top"
android:background="#33b5e5"
android:textColor="#fff"
android:paddingTop="4dp"
android:paddingBottom="4dp" />
</android.support.v4.view.ViewPager>
All my action bar imports are support version 7. All my fragment imports are support version 4. Make sure you are only using these versions of the imports and that there are no other versions imported. In my SectionsPagerAdapter, import:
import java.util.Locale;
import android.content.Context;
import android.support.v4.app.Fragment;
import android.support.v4.app.FragmentManager;
import android.support.v4.app.FragmentPagerAdapter;
and in main activity, import:
import android.content.Intent;
import android.support.v7.app.ActionBarActivity;
import android.support.v7.app.ActionBar;
import android.support.v4.app.FragmentPagerAdapter;
import android.support.v4.view.ViewPager;
import android.os.Bundle;
import android.util.Log;
import android.view.Menu;
import android.view.MenuItem;
import com.parse.ParseUser;
these are the only imports being used in my program, so you can cut and paste and the code should run . I've tried it and it runs with only these imports. Let me know.
And that brings us up to date! I consolidated this into one LONG answer, but it should be pretty clear, and you won't find a better conversion on the net! I hope that tones down some of the confusion about the crossed out methods and how to deal with them. Happy coding!
Nicolas
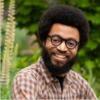
Nicolas Hampton
44,638 PointsBen Jakuben , you might want to take a look at this for class notes, I think it clears a lot up. I'm communicating with @LeJacque Gillespie to see if this helps and fixes all his problems and if he can follow it, and the last lesson of this class has almost been rendered obsolete. Everything other than that, with this fix, seems to be fine.

Stephen Little
8,312 PointsI get an error for this code I got from here
@Override
public boolean onCreateOptionMenu(Menu menu){
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.menu_main,menu);
return true;
}
Method doesn't over ride method from it's supercalss....
and this code
@Override
public Fragment getItem(int position) {
// getItem is called to instantiate the fragment for the given page.
// Return a PlaceholderFragment (defined as a static inner class below).
//return MainActivity.InboxFragment.newInstance(position + 1);
return MainActivity.PlaceholderFragment.newInstance(position +1);
}
``
error of
Incompatible type,
Required: android.support.v4.Fragment
Found: virtualtruro.com.ribbit.MainActivity.PlaeholderFragment.
any help would be great, this course is really starting to get to me and I wondering if I'm really learning anything. I understand enough to get into trouble but I am finding it hard having to look other places to fix this.
Cheers!
Stephen
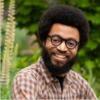
Nicolas Hampton
44,638 PointsYou're misspelling the OnCreateOptionsMenu function, so it's not overriding the method, first problem. Working on the other.
The second error seems to be spelling as well, as in the PlaceholderFragment method, you seem to have spelled it PlaeholderFragment, so the compiler doesn't know it's a fragment. Check your code for that method.

Stephen Little
8,312 PointsI fixed the first spelling problem (can't believe I missed that) but I can not find the second one you talked about. My PlaceholderFramgment method is as follows... from the main activity right..
public static class PlaceholderFragment extends Fragment {
/**
* The fragment argument representing the section number for this
* fragment.
*/
private static final String ARG_SECTION_NUMBER = "section_number";
public PlaceholderFragment() {
}
/**
* Returns a new instance of this fragment for the given section
* number.
*/
public static PlaceholderFragment newInstance(int sectionNumber) {
PlaceholderFragment fragment = new PlaceholderFragment();
Bundle args = new Bundle();
args.putInt(ARG_SECTION_NUMBER, sectionNumber);
fragment.setArguments(args);
return fragment;
}
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState){
View rootView = inflater.inflate(R.layout.fragment_inbox,container,false);
return rootView;
}
}
maybe I am just blind, been a long few days :(
Cheers! Stephen

roi shaul
3,196 Pointsneed halp. i get an error: " Caused by: java.lang.IllegalStateException: You need to use a Theme.AppCompat theme (or descendant) with this activity."
the lines with the error is: protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main);
what can i do?

Ricardo Sierra
14,920 PointsHi Nicolas! I was just wondering what to do after making the changes, do I go on with the videos, creating the InboxFragments and such? What code do I write on them??

Timothy Boland
18,237 Pointsneed help. i get an error: " Caused by: java.lang.IllegalStateException: You need to use a Theme.AppCompat theme (or descendant) with this activity."

CD Lim
4,782 PointsDear Nicolas, I really appreciate your time and effort in explaining all the details.
Regards
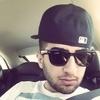
Vrezh Gulyan
11,161 PointsFirst of all Nicholas Hampton thank you for the video! Two issues I came across..
android.view.View;
android.view.ViewGroup
These are necessary imports and I don't know how you were able to compile the app without having errors in this section of MainActivity.java
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
View rootView = inflater.inflate(R.layout.fragment_main, container, false);
return rootView;
}
Secondly I had the same problem as Stephen Little above but it is not a typo.
@Override
public Fragment getItem(int position) {
// getItem is called to instantiate the fragment for the given page.
// Return a PlaceholderFragment (defined as a static inner class below).
return MainActivity.PlaceholderFragment.newInstance(position + 1);
}
The error is that this method requires a Fragment get returned but instead we return a PlaceholderFragment from MainActivity. How did you get this to work?
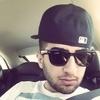
Vrezh Gulyan
11,161 PointsI fixed my own problem just messing around and figuring it out.. I added
import android.support.v4.app.Fragment;
to MainActivity.java
and changed this bit of code so the right type of fragment is being returned. I changed what PlaceholderFragment extends.. deleted import android.Fragment; since it conflicts with the new import
public static class PlaceholderFragment extends android.support.v4.app.Fragment {
/**
* The fragment argument representing the section number for this
* fragment.
*/
private static final String ARG_SECTION_NUMBER = "section_number";
/**
* Returns a new instance of this fragment for the given section
* number.
*/
public static Fragment newInstance(int sectionNumber) {
Fragment fragment = new Fragment();
Bundle args = new Bundle();
args.putInt(ARG_SECTION_NUMBER, sectionNumber);
fragment.setArguments(args);
return fragment;
}
public PlaceholderFragment() {
}
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
View rootView = inflater.inflate(R.layout.fragment_main, container, false);
return rootView;
}
}
Hope this helps someone, my app runs fine now with tabs and all.
hubertl
8,483 PointsYou read in my mind :) great work! Thank you for your contribution.

John Hughes
867 PointsThis was indeed the droid I was looking for. Thank you.

Nicholas Kotsiantos
8,827 PointsActionBarActivity was deprecated....so know what do I use?

Timothy Boland
18,237 Pointsmake sure you are using the correct one....make sure the following is imported:
import android.support.v7.app.ActionBarActivity;

Michael Fraser
11,229 PointsEven when specifying ActionBarActivity as android.support.v7.app.ActionBarActivity Android Studio still tells me it is deprecated. I instead extended AppCompatActivity, but may have needed some additional imports to get it working (android studio recommended the imports).

Andre Colares
5,437 PointsGuys, thank you so much for the example.
My code is working, but when I launch 1st time the app, the Title INBOX isn't showing. This title only shows when I scroll to left 1 one time. Yours are like mine?
jenyufu
3,311 Pointsjenyufu
3,311 PointsI'm using Android Studio 1.2.2 and mine works without having to change to PagerTabStrip. Do I still have to change it? Or can I continue on with the lesson this way?