Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial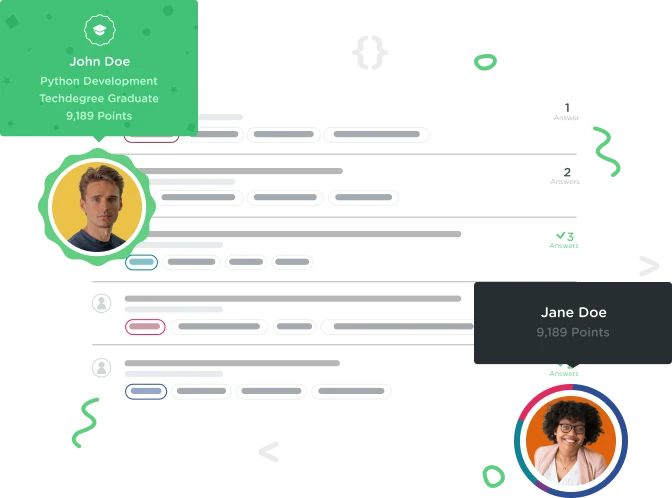

Jerry Schrader
4,396 PointsThis is where I seem to get confused. There are so many elements/names, can someone explain $array as $element?
I mean I kind of get it, but there is no way I could write this out in the real world out of my head. I need to grasp what's happening here. Thanks!
<?php
function mimic_array_sum($array) {
$sum = 0;
foreach($array as $element) {
$sum = $sum + $element;
}
return $element;
}
$palindromic_primes = array(11, 757, 16361);
$sum = mimic_array_sum($palindromic_primes);
echo $sum;
?>
4 Answers

Christian Andersson
8,712 PointsFor the sake of example, let's assume that $array
is an array that contains 5 elements. It could look something like this:
$array[0] = 'Apple'
$array[1] = 'Orange'
$array[2] = 'Banana'
$array[3] = 'Melon'
$array[4] = 'Strawberry'
When you want to iterate through an array in php, you usually want to use foreach
. The following syntax:
foreach($array as $element)
basically reads "Repeat the following code for each item inside the array, and call it (the current item) 'element'".
So on the first iteration $element
will contain the value "Apple", and on the second "Orange", and so on. The loop stops when it has iterated through all the items in the $array
array. So $element
is just a temporary variable that is used to store the array contents as you loop through them one-by-one.
Hope this helps :)

Jerry Schrader
4,396 PointsSo I guess ultimately in my example the variable $array could be called anything? It's just kind of a placeholder? I don't see anywhere that it's passed a value, I appreciate your response, and yes it does help.

Christian Andersson
8,712 PointsAny and all variables can be called anything (though there are a couple of rules that apply). In this case it's called $array just to make it obvious that it's an array. But you could call it $jerry if you prefer :D
In your example, $array is only seen in the function. The important thing about function is that any and all code within the function is almost like a bubble -- abstract from the rest of the code. For example, you cannot use a variable that you used ealier in the code, in contrast to "regular code". You must see functions and the "main code" as two separate and independent pieces of code that do not interract with eachother. The only common they have is that they are written in the same document.
If you want to send a variable (or as many as you want) you must send what's called a parameter to the function. Parameters are denoted by the values stated within the parenthesis next to the function name. In your example, your function is called mimic_array_sum
and accepts one variable which it calls $array.
What's important to note here that whenever you call the function mimic_array_sum
and pass it an argument, the function will still call that argument $array. When you pass an argument you essentially make the function make a copy of that variable. It then does the operations that are written in that function, and when done returns a result back to the "main code".
Here is a code example:
function find_double($nbr) {
$result = 2 * $nbr;
return $result;
}
$nbr = 5;
$variable = 9;
$test1 = find_double($nbr);
$test2 = find_double($variable);
$test3 = find_double(13);
echo $test1;
echo $test2;
echo $test3;
First, I declare a function which I call find_double
. This function accepts one variable, which locally (and only locally within the function) is called $nbr. Then I make two variables with values 5 and 9. Finally I call the function 3 times, each time giving it a different parameter. The $test1/2/3 will store the return-value of the function which I then echo out.
As you can see, when I call the function, I can pass any variable or value I want. Name doesn't matter. It can be the same name as used locally inside the function, or not. The function will be run 3 times because it's called 3 times. If we were to somehow pause the function midway and look up the value of $nbr, we would see that it has the value: 5, the first time it's called, then 9 and finally 13.
So to answer your question, $array
was never created because it's a parameter for a function -- it will just copy over whatever value you sent into it. So if you want to send it an array, you probably have created it elsewhere in your code. Indeed, it was created on the line:
$palindromic_primes = array(11, 757, 16361);
Here you create an array called "palindromic_primes" with the 3 values stored within it: 11, 757, and 16361. On the next line you send this array into the mimic_array_sum function. The function will return a result at some point which the "main program" awaits to retrieve and store in the variable $sum
.
If you haven't already, check out Treehouses PHP function course. It's quite small and easy to do within a day or two: http://teamtreehouse.com/library/php-functions-2

Jerry Schrader
4,396 PointsThank you so much! I will go through the php function course immediately, and your explanation did help a lot.

Ben Wong
19,426 PointsYou got it mostly right.
return $element;
chance ^^^ to return $sum