Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial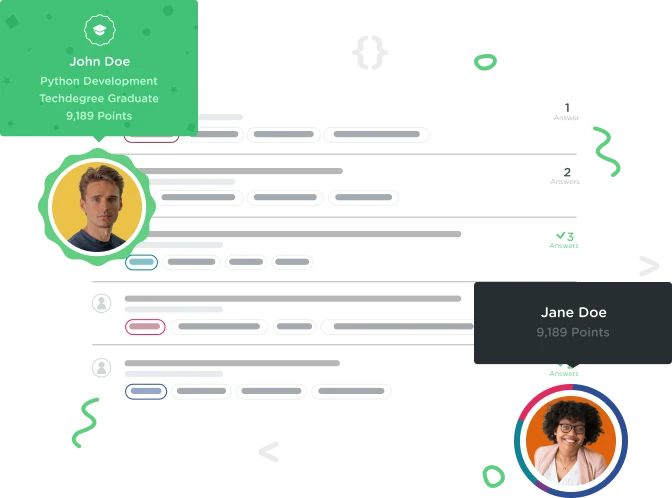

Kars Jansens
5,348 PointsThis is working in my IDE [Python] slices
The question is: You're on fire! Last one and it is, of course, the hardest.
Make a function named reverse_evens that accepts a single iterable as an argument. Return every item in the iterable with an even index...in reverse.
For example, with [1, 2, 3, 4, 5] as the input, the function would return [5, 3, 1].
You can do it!
In my IDE this works fine. I really don't understand why this doesn't work.
def first_4(variable):
return variable[:4]
def first_and_last_4(variable):
variable[:4] += variable[4:]
return variable[:4] + variable[-4:]
def odds(variable):
return variable[1::2]
def reverse_evens(variable):
return variable[::-2]
6 Answers
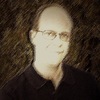
Jason Anders
Treehouse Moderator 145,858 PointsHi Kars Jansens
Yes, if you send in an odd number of items in the list (eg. 1, 2, 3, 4, 5)... that code will work. But, did you test that code with an even-numbered list (eg. 1, 2, 3, 4, 5, 6)? With that, the code won't work.
I'll give you a hint: You could use an if statement
to test if it's an even or an odd numbered list and then use the appropriate slice function on it.
The rest looks great. Nice work! :)

Kars Jansens
5,348 PointsI do not understand how I can do this with an if statement, so I tried it with a try statement.
def first_4(variable): return variable[:4]
def first_and_last_4(variable): variable[4:] += variable[:4] return variable[:4] + variable[-4:]
def odds(variable): return variable[1::2]
def reverse_evens(variable): try: variable[::-2] except TypeError: variable.remove(variable[-1]) return variable[::-2]
It works fine with every amount of numbers in my list in pycharm. But it still doesn't work.
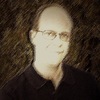
Jason Anders
Treehouse Moderator 145,858 PointsIn my opinion, an if statement
is best. A try
block will not work for this test, and you can't remove something from the list just to make it fit the criteria. If this were a real-life scenario, you wouldn't go removing items from a list just to make your code work, right?
With an if statement
you test the incoming list to see if it is an even length or an odd length. This would be done with the modulus
operator. Once you figure the length, you simply start the slice either at the last item (odd list) or at the second-to-last item (even list) and then progress the slice like you have in your code. Where to start the slice goes on the right of the :: and the incremental movement goes on the left.
The code will look something like this:
def reverse_evens(variable):
if len(variable) % 2 == 0: # testing the list
# even list code here
else:
# odd list code here
You should be able to get it from here. :)

Kars Jansens
5,348 PointsI hope :^)

Prashant Nayak
2,300 PointsThanks Jason for the help , not sure why it is not working for me, with below logic
if len(variable) % 2 !=0:
return variable[::-2]
else:
return variable[-2::-2]
Moderator edit: Added markdown for readable code block
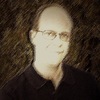
Jason Anders
Treehouse Moderator 145,858 PointsYou're logic is correct. I believe it is probably your indentation. When posting code to the forums, you need to use Markdown. There is a cheatsheet link above the post button, or a course on Markdown here at treehouse.
But, when I do copy/paste your code from above, the indents are off by a some spaces, and the else shold not be indented. When I correct the indentation, the code does pass. So, just double check indentation, as Python is very specific when it comes to that.
Nice work on the logic! :)

Prashant Nayak
2,300 Pointsthank you for kind on the logic .
Code is running fine in IDLE ,but not sure why i am getting an error while submitting the answer.
def reverse_evens (variable): if len(variable) % 2 == 0: return variable[-2::-2] else: return variable[::-2]
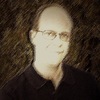
Jason Anders
Treehouse Moderator 145,858 PointsYour code does pass the challenge when indentation is corrected. Just because it works locally doesn't mean it will work in the challenge if you just copy/paste.
It may be because you are tabbing
the indentation instead of using spaces? Python uses 4 spaces to indent lines not a tab (in an editor, you may have the tab set to place 4 spaces). You can notice how off the indentation is in your above snippet, once I added the Markdown needed to make it readable. I can't see in your most recent code, as you didn't use Markdown to post it.
I recommend you manually type in the code to the challenge, and make sure you are using 4 spaces to indent the lines. Look for any red lines in the challenge, as this will indicate errors with spacing.

Prashant Nayak
2,300 PointsThanks, Jason it worked now :)