Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial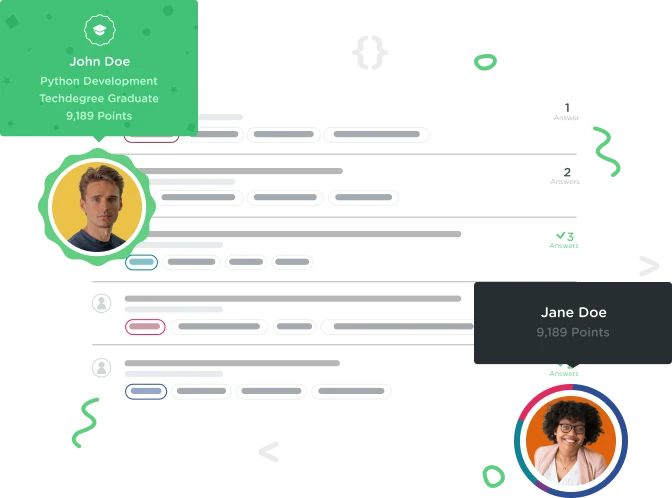

karan Badhwar
Web Development Techdegree Graduate 18,135 Pointsthis Keyword
Over here the 'this' keyword, is it referring to the class Pet or the new object that will be created.
So class pet itself is an Object as well right? and it is a Object blueprint as in it a structured Object for any new Object with predefined keys. Is my understanding of the classes is right?
One more questions, When we did this.animal = animal, why the animal after after the = operator is getting the passed value, not the this.animal Variable, generally that what happens right, we pass the value and it gets assigned to the variable with same name?
5 Answers
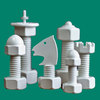
Steven Parker
231,275 PointsThe class defines the objects ("instances") that will be created, but it is not an object itself.
Inside an instance method, "this" refers to the instance that the method will be called on.

karan Badhwar
Web Development Techdegree Graduate 18,135 PointsI got it now thankyou

karan Badhwar
Web Development Techdegree Graduate 18,135 PointsThankyou Steven, Happy Holidays and merry charismas to you and your family

Daoud Merchant
5,135 PointsWith respect to the commenters thus far, I feel it important to clarify for everyone reading this that in JavaScript, a class
is an object itself, unlike almost every other programming language. This is because, as Ms. Boucher explains, class
is just syntactic sugar for prototypes.
I could give examples, but nothing I could say would surpass the superlative Objects and Classes from the 'You Don't Know JS' series. It's a dense read, you will likely revisit the book later, but if you really want to understand what's going on it's the place to go.
Specifically, Mr. Simpson explains what a JavaScript class
is (and isn't) here. Hope this helps!
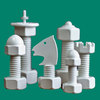
Steven Parker
231,275 PointsYou are correct that a class is fancy syntax for more basic facilities; but while a class contains a prototype object, the class is not an object itself. If you have any doubts, try defining a class and then do this:
console.log(typeof YOUR_CLASS_NAME); // of course, substitute your actual class name
... and see what you get.  
But I think we've already stepped beyond the scope of this question, further deconstruction is likely to be more confusing than enlightening.  

Daoud Merchant
5,135 PointsIndeed, the above returns function
, and:
In JavaScript, functions are first-class objects, because they can have properties and methods just like any other object. What distinguishes them from other objects is that functions can be called. In brief, they are Function objects.
https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Functions
I apologise for the lack of clarity if it was understood that I was stating that the class
itself is a prototype; I meant that the class
system is syntactic sugar for the prototype system in JavaScript.
Declaring a class is to declare a function (the constructor
) and to declare methods which are assigned to this function's prototype, and instantiating a class is to call the function as a constructor function (with the new
operator), the newly created object sharing a prototype with the original function and thereby being able to access that prototype's methods.
Consider the following:
class ClassPerson {
constructor(name) {
this.name = name;
}
greet() {
return `Hi, I'm ${this.name}!`;
}
};
const jim = new ClassPerson("Jim");
jim.name; // "Jim"
jim.greet(); // "Hi, I'm Jim!"
const FunctionPerson = function(name) {
this.name = name;
};
FunctionPerson.prototype.greet = function() {
return `Hi, I'm ${this.name}!`;
};
const bob = new FunctionPerson("Bob");
bob.name; // "Bob"
bob.greet(); // "Hi, I'm Bob!"
I hope this better communicates what I was trying to explain. Wishing all the very best.
karan Badhwar
Web Development Techdegree Graduate 18,135 Pointskaran Badhwar
Web Development Techdegree Graduate 18,135 PointsI did nor get it can you elaborate, Please?
Steven Parker
231,275 PointsSteven Parker
231,275 PointsThe class is like a blueprint, it is instructions for making an object, but it is not an object itself. When you use it to make an object, that object is known as an instance of the class.
In the class, "this" refers to the instance (that will be created later). When they wrote
this.animal = animal
, the term on the left is a variable inside the instance (also known as a "property"), and the one on the right is just a parameter that refers to the argument that will be passed in when the instance (the object) is created. If it seems confusing, consider that the parameter name could be anything. For example, this would work just as well: