Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial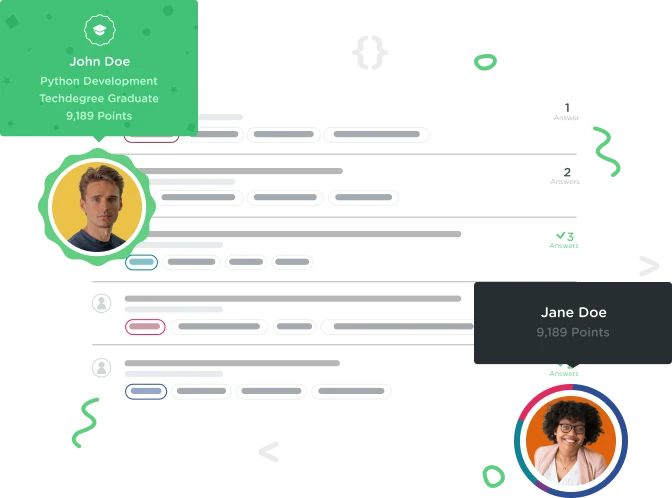

frank sebring
1,037 PointsThis look correct to me. It returns four strings in one tuple with the desired formats. Any ideas??
This code will return the correct results. If the word = "hello"
answer: ('HELLO', 'hello', 'Hello', 'olleh')
I added a test to the bottom of the code so it can be easily ran. When submitted I did not have the two test lines in the code.
def stringcases(word:str):
results = []
## This will change the input to the desired format ##
wtu = results.append(word.upper())
wtl = results.append(word.lower())
wtt = results.append(word.capitalize())
## This loop will put the user input into a list backwards / combine that input / add it to the list
newList = []
start = -1
count = 0
wordLength = len(word)
for i in word:
if count < wordLength:
newList.append(word[start])
count += 1
start -= 1
reverseWord = ''.join(newList)
results.append(reverseWord)
## Makes the new list into a tuple
new_results = tuple(results)
return new_results
test = stringcases("HeLlO")
print(test)
1 Answer

andren
28,558 PointsThe issue is the third string case. The task asks you to format it with title-casing. With title-casing you have to capitalize the first letter of each word. The capitalize
method only capitalizes the first letter of the string, not each word in the string.
So a string like "hello world" would become "Hello world" when passed though the capitalize
method, while it should be like this "Hello World" for it to be a valid title-cased string.
Luckily Python does also have a method for title-casing which is simply called title
. So if you replace capitalize
with title
like this:
def stringcases(word:str):
results = []
## This will change the input to the desired format ##
wtu = results.append(word.upper())
wtl = results.append(word.lower())
wtt = results.append(word.title())
## This loop will put the user input into a list backwards / combine that input / add it to the list
newList = []
start = -1
count = 0
wordLength = len(word)
for i in word:
if count < wordLength:
newList.append(word[start])
count += 1
start -= 1
reverseWord = ''.join(newList)
results.append(reverseWord)
## Makes the new list into a tuple
new_results = tuple(results)
return new_results
Then your code will work. Though I would like to point out that your string reversal code is a tad overkill. You learned about slices earlier in this course, when using slices you can define a step counter which determines how Python steps though the string or list. If you provide it with a negative step then it will go though the string backwards, which results in it becoming reversed.
So your code can be replaced with this more simple code:
def stringcases(word:str):
results = []
## This will change the input to the desired format ##
wtu = results.append(word.upper())
wtl = results.append(word.lower())
wtt = results.append(word.title())
wtr = results.append(word[::-1]) # Declare a slice with a step of -1
new_results = tuple(results)
return new_results
Since all of the text transform methods are now pretty short, you could even throw them all into one succinct line like this:
def stringcases(word:str):
return word.upper(), word.lower(), word.title(), word[::-1]
And the code would still be pretty easy to read.