Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial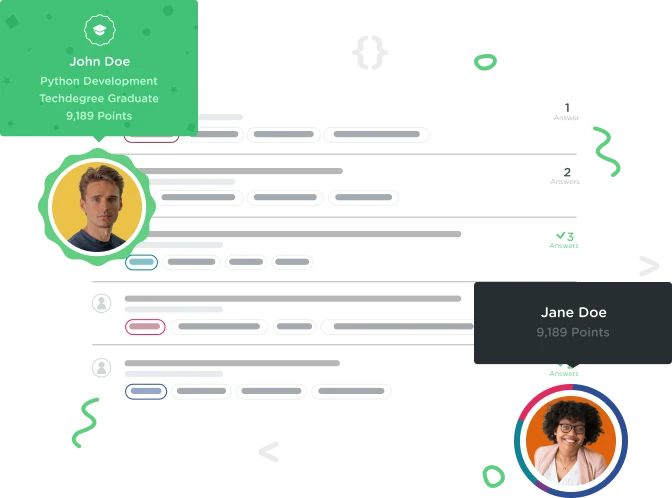
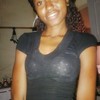
MUZ140820 Immaculate Ncube
4,611 PointsThis music app has three tabs. In MainActivity below, set the text for each tab using the method setText(). As the param
i didn't get this
import android.app.ActionBar;
import android.app.FragmentTransaction;
import android.os.Bundle;
import android.support.v4.app.FragmentActivity;
public class MainActivity extends FragmentActivity implements
ActionBar.TabListener {
// Some code has been omitted for brevity!
public ActionBar mActionBar;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
mActionBar = getActionBar();
mActionBar.setNavigationMode(ActionBar.NAVIGATION_MODE_TABS);
PagerAdapter pagerAdapter = new PagerAdapter(getSupportFragmentManager());
// Some code omitted for brevity...
for (int i = 0; i < pagerAdapter.getCount(); i++) {
ActionBar.Tab tab = mActionBar.newTab();
tab.setTabListener(this);
/*
* Add your code here to set the text!
*/
mActionBar.addTab(tab);
}
}
// These listener methods are intentionally blank
@Override
public void onTabSelected(ActionBar.Tab tab, FragmentTransaction fragmentTransaction) { }
@Override
public void onTabUnselected(ActionBar.Tab tab, FragmentTransaction fragmentTransaction) { }
@Override
public void onTabReselected(ActionBar.Tab tab, FragmentTransaction fragmentTransaction) { }
}
import android.app.ActionBar;
import android.app.FragmentTransaction;
import android.os.Bundle;
import android.support.v4.app.FragmentActivity;
public class ArtistActivity extends FragmentActivity implements
ActionBar.TabListener {
// Some code has been omitted for brevity!
public ActionBar mActionBar;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_artist);
mActionBar = getActionBar();
mActionBar.setNavigationMode(ActionBar.NAVIGATION_MODE_TABS);
PagerAdapter pagerAdapter = new PagerAdapter(getSupportFragmentManager());
// Some code omitted for brevity...
for (int i = 0; i < pagerAdapter.getCount(); i++) {
ActionBar.Tab tab = mActionBar.newTab();
tab.setTabListener(this);
/*
* Add your code here to set the icon!
*/
mActionBar.addTab(tab);
}
}
// These listener methods are intentionally blank
@Override
public void onTabSelected(ActionBar.Tab tab, FragmentTransaction fragmentTransaction) { }
@Override
public void onTabUnselected(ActionBar.Tab tab, FragmentTransaction fragmentTransaction) { }
@Override
public void onTabReselected(ActionBar.Tab tab, FragmentTransaction fragmentTransaction) { }
}
import android.content.Context;
import android.support.v4.app.FragmentManager;
import android.support.v4.app.FragmentPagerAdapter;
public class PagerAdapter extends FragmentPagerAdapter {
/*
* Some code has been omitted for brevity!
*/
public PagerAdapter(FragmentManager fm) {
// code omitted
}
@Override
public int getCount() {
return 3;
}
@Override
public CharSequence getPageTitle(int position) {
switch (position) {
case 0:
return "Songs";
case 1:
return "Albums";
case 2:
return "Artists";
}
return null;
}
public int getIcon(int position) {
switch (position) {
case 0:
return R.drawable.ic_tab_profile;
case 1:
return R.drawable.ic_tab_discography;
case 2:
return R.drawable.ic_tab_related_artists;
}
return R.drawable.ic_tab_profile;
}
}
2 Answers
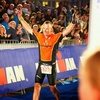
Steve Hunter
57,712 PointsHi there,
There's a for
loop already set up that iterates through all the tabs. The PagerAdapter class, and all its instances, has a method called getPageTitle()
. If you look at the method, it takes an integer as an argument. The method returns the required title depnding on the integer value passed to it.
Do, using the method looks like:
pagerAdapter.getPageTitle(i);
Where pagerAdapter
is declared in the MainActivity
. The i
is the counter in the for
loop that cycles through the tabs, creating them as it goes. So, each loop creates a new tab called tab
locally that needs a title which can be set with the above method.
The final code looks like:
tab.setText(pagerAdapter.getPageTitle(i));
That completes the first part of the challenge. The second part looks pretty similar.
Hope that helps and good luck!!
Steve.

Bhekimpilo Ncube
4,157 PointsTjovoo Stevooo