Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial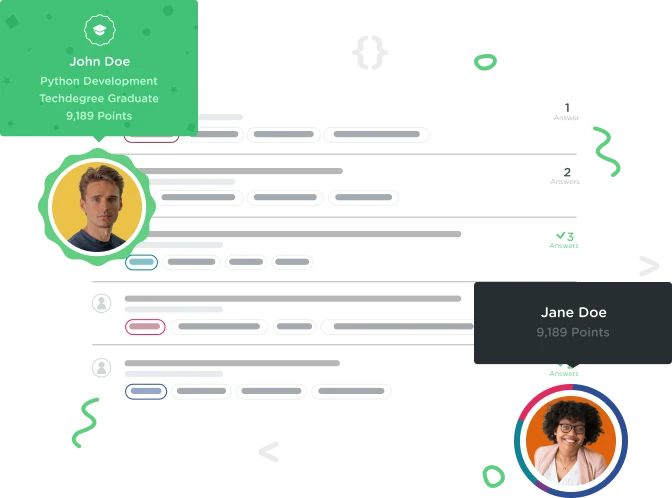
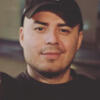
victor E
19,145 Pointsthis one was a really tough one for me
not sure how to approach this one to be honest
def sillycase(word):
half = (int.len(word))/2
word = upper.word[-1:-{}].format(half)
return(word)
3 Answers
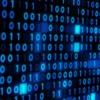
Alexander Davison
65,469 PointsThis one is a tough one indeed! The most effective way to solve a problem is to split it up into easy problems, and solve each one of those mini-problems. Perhaps this problem can be split up into five steps:
- Find the index of the midpoint
- Select the first half of the string
- Select the second half
- Convert first half to lowercase
- Convert second half to uppercase
Each one of these problems are pretty easy.
def sillycase(string):
midpoint = len(string) // 2 # Step 1
first_half = string[:midpoint] # Step 2
second_half = string[midpoint:] # Step 3
lowercase = first_half.lower() # Step 4
uppercase = second_half.upper() # Step 5
return lowercase + uppercase
Once you've got this, you can refactor (simplify) the code a little bit:
def sillycase(string):
midpoint = len(string) // 2
first_half = string[:midpoint].lower()
second_half = string[midpoint:].upper()
return first_half + second_half
The above code is the cleanest code I can think of, and I personally would solve the challenge with the code above, but if you are going for minimal lines (and minimum clarity), this snippet is the winner:
def sillycase(string):
return string[:(len(string)//2)].lower() + \
string[(len(string)//2):].upper()
I hope this helps! ~Alex

Kristian Vrgoc
3,046 PointsHey, why are you using the \ after the + in the last code ? Thanks. Kris
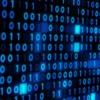
Alexander Davison
65,469 PointsHello Kristian,
The \
tells Python that the next line is actually part of the current line.
This would cause an error:
def sillycase(string):
return string[:(len(string)//2)].lower() +
string[(len(string)//2):].upper()
But this will work:
def sillycase(string):
return string[:(len(string)//2)].lower() + \
string[(len(string)//2):].upper()
You may say, "But wait! Python usually figures out if the line is complete or not, and it will automatically do this for me!". You're right; Python is clever. In certain cases, like this...
print(
"Hello world!"
)
Python appropriately prints "Hello world!" as you might expect. But in the case of something like this:
1 +
2
Python freaks and throws a SyntaxError
. In this case you might want to write
1 + \
2
# Or, even better:
1 + 2
I hope this helps!
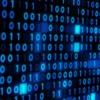
Alexander Davison
65,469 PointsGenerally, you should never use \
. Try to put all of the code on the same line. If the code looks too confusing (or if the line exceeds ~79 characters), split the line into multiple lines of code.
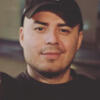
victor E
19,145 PointsThank you I appreciate this!
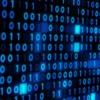
Alexander Davison
65,469 PointsYou're very welcome :)

Kristian Vrgoc
3,046 Points@ Alexander Davison Thanks you very much. Kris
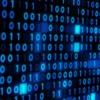
Alexander Davison
65,469 PointsNo problem.
Kent Åsvang
18,823 PointsKent Åsvang
18,823 PointsIf you could paste in more information about the challenge I could try and help out.