Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial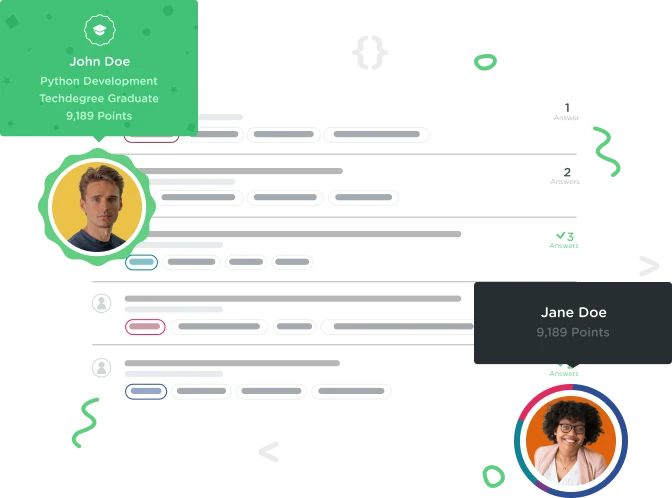

luke jones
8,915 PointsThis part goes way over my head.
I feel like I've hit a wall here and need some parts broken down a little more so i can understand it better. Some parts i understand and some parts i don't. Putting all the code together and once has completely fried my brain.
I've also customised the code slightly myself my implementing 'z' into the code. This is so i can hopefully learn it myself a little more rather than just copy and paste. Is this fine?
For some reason i can't seem to get the coding to layout all separately from the text. I'm guessing copying and pasting into playground would solve it.
struct Point {
let x: Int
let y: Int
let z: Int
func surroundingPoints(withrange range:Int = 1) -> [Point] {
var results: [Point] = []
for xCoord in (x-range)...(x+range){
for yCoord in (y-range)...(y+range){
for zCoord in (z-range)...(z+range){
let coordinatePoint = Point(x: xCoord, y: yCoord, z: zCoord)
results.append(coordinatePoint)
}
}
}
return results
}
}
class Enemy {
var life: Int = 2
var position: Point
init (x: Int, y:Int, z:Int) {
self.position = Point(x: x, y: y, z: z)
}
func decreaseHealth(factor: Int){
life -= factor
}
}
class Tower {
let position: Point
var range: Int = 1
var strength: Int = 1
init(x: Int, y:Int, z:Int) {
self.position = Point(x: x, y: y, z: z)
}
func fireAtEnemy(enemy: Enemy) {
if inRange(self.position, range: self.range, target: enemy.position) {
while enemy.life > 0 {
enemy.decreaseHealth(self.strength)
print("Enemy vanquished")
}
}
else {
print("Out of range")
}
}
func inRange(position: Point, range:Int, target: Point) -> Bool{
let availablePositions = position.surroundingPoints(withrange: range)
for point in availablePositions {
if (point.x == target.x) && (point.y == target.y) && (point.z == target.z) {
return true
}
}
return false
}
}
My two sticking points are the inRange function and the fireAtEnemy function. I feel like i briefly understand what its doing but i dont understand each individual part of the function. i've watched the video multiple times but when combining it all together in my head i just can't seem to make it click. Apologies in advance if the code layout on here looks messy.
1 Answer
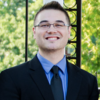
ianhan3
4,263 PointsNote: I've never watched the video but from your code I'll try to explain the functions in plain english.
-fireAtEnemy: So you have a function that has one input: a variable called enemy of type Enemy (defined above in the Enemy class). If the inRange function (which takes in three variables - the position, range, and target) is true, the function fires. The while loop states that while the enemies "life", the life property of the enemy (which stated in the class is 2 and not changed in the function), is greater than 0, the enemies health will decrease by the tower strength each time the function is called. It seems that the tower starting strength is 1 meaning that the enemy would have to be hit twice for the enemies health to hit 0 and the while loop to stop firing.
-inRange: A function that takes in three variables, two of class point and one Int, and returns whether the object is "inRange" or not (true or false). It iterates through the available positions constant (which is calculated from the input variables) and for each "point" (the temporary constant) in the availablePositions array it will do a check. In this case, the check is if the X coordinate point, y coordinate point, and z coordinate point are all equal to their respective target points (==), that point will return true.
agreatdaytocode
24,757 Pointsagreatdaytocode
24,757 PointsGreat brake down Ian.