Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial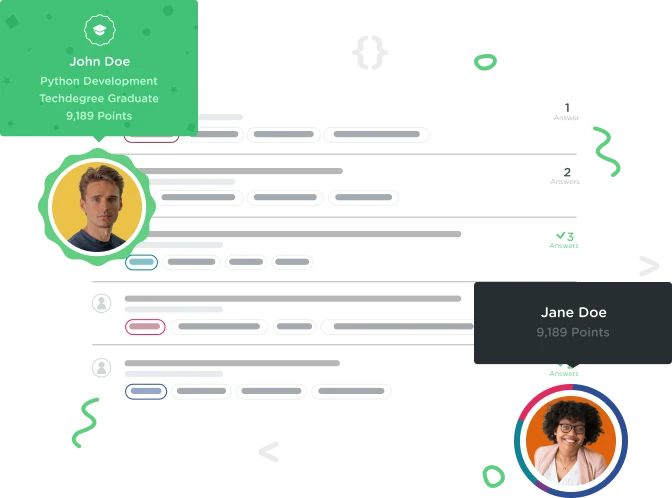

Andrei Oprescu
9,547 PointsThis question is confusing, help.
Currently I have this question:
Now in your new method, have it return a number representing the count of tiles that match the letter that was passed in to the method. Make sure to check Example.java for some example uses.
And I have this code for it:
public class ScrabblePlayer { // A String representing all of the tiles that this player has private String tiles;
public ScrabblePlayer() { tiles = ""; }
public String getTiles() { return tiles; }
public void addTile(char tile) { tiles += tile; }
public boolean hasTile(char tile) { return tiles.indexOf(tile) != -1; }
public int getCountOfLetter(char letter) { int countOfTiles = 0; for (int tiles : letter.toCharArray()) { if (tiles.IndexOf(letter) != -1) { countOfTiles++; } } return countOfTiles; }
}
it always says char cannot be dereferenced on line 23 (for (int tiles : letter.toCharArray()) {):
can you tell me how I can fix this and what it means?
Andrei.
public class ScrabblePlayer {
// A String representing all of the tiles that this player has
private String tiles;
public ScrabblePlayer() {
tiles = "";
}
public String getTiles() {
return tiles;
}
public void addTile(char tile) {
tiles += tile;
}
public boolean hasTile(char tile) {
return tiles.indexOf(tile) != -1;
}
public int getCountOfLetter(char letter) {
int countOfTiles = 0;
for (int tiles : letter.toCharArray()) {
if (tiles.IndexOf(letter) != -1) {
countOfTiles++;
}
}
return countOfTiles;
}
}
// This code is here for example purposes only
public class Example {
public static void main(String[] args) {
ScrabblePlayer player1 = new ScrabblePlayer();
player1.addTile('d');
player1.addTile('d');
player1.addTile('p');
player1.addTile('e');
player1.addTile('l');
player1.addTile('u');
ScrabblePlayer player2 = new ScrabblePlayer();
player2.addTile('z');
player2.addTile('z');
player2.addTile('y');
player2.addTile('f');
player2.addTile('u');
player2.addTile('z');
int count = 0;
// This would set count to 1 because player1 has 1 'p' tile in her collection of tiles
count = player1.getCountOfLetter('p');
// This would set count to 2 because player1 has 2 'd'' tiles in her collection of tiles
count = player1.getCountOfLetter('d');
// This would set 0, because there isn't an 'a' tile in player1's tiles
count = player1.getCountOfLetter('a');
// This will return 3 because player2 has 3 'z' tiles in his collection of tiles
count = player2.getCountOfLetter('z');
// This will return 1 because player2 has 1 'f' tiles in his collection of tiles
count = player2.getCountOfLetter('f');
}
}
2 Answers
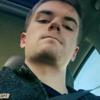
Kyle Shifflett
8,496 PointsYeah, looks like you need String tiles. You can't iterate over one char, or at least it's not what the problem is asking.
public int getCountOfLetter(String tiles) {
int countOfTiles = 0;
for (int tiles : letter.toCharArray()) {
if (tiles.IndexOf(letter) != -1) {
countOfTiles++;
}
}
return countOfTiles;
}

Andrei Oprescu
9,547 Pointskyle Shifflett,
Are you sure you code is correct?
It gives me 5 errors:
JavaTester.java:127: error: incompatible types: Character cannot be converted to String int actual = player.getCountOfLetter(entry.getValue()); ^ ./ScrabblePlayer.java:23: error: cannot find symbol for (int tiles : letter.toCharArray()) { ^ symbol: variable letter location: class ScrabblePlayer ./ScrabblePlayer.java:23: error: variable tiles is already defined in method getCountOfLetter(String) for (int tiles : letter.toCharArray()) { ^ ./ScrabblePlayer.java:24: error: cannot find symbol if (tiles.IndexOf(letter) != -1) { ^ symbol: variable letter location: class ScrabblePlayer ./ScrabblePlayer.java:24: error: int cannot be dereferenced if (tiles.IndexOf(letter) != -1) { ^ Note: JavaTester.java uses unchecked or unsafe operations. Note: Recompile with -Xlint:unchecked for details. Note: Some messages have been simplified; recompile with -Xdiags:verbose to get full output 5 errors
Can you please update you answer?
thank you.

Derek Hawkins
12,783 PointsHey,
You could try this instead:
public int getCountOfLetter(String tiles) { int countOfTiles = 0; for (int letter1 : tiles.toCharArray()) { if (letters1 == letters) { countOfTiles++; } } return countOfTiles; }
Oliver Hull
845 PointsOliver Hull
845 Pointsyou are trying to iterate over 'letter', which is a char. It looks like you want to iterate over 'tiles' instead