Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial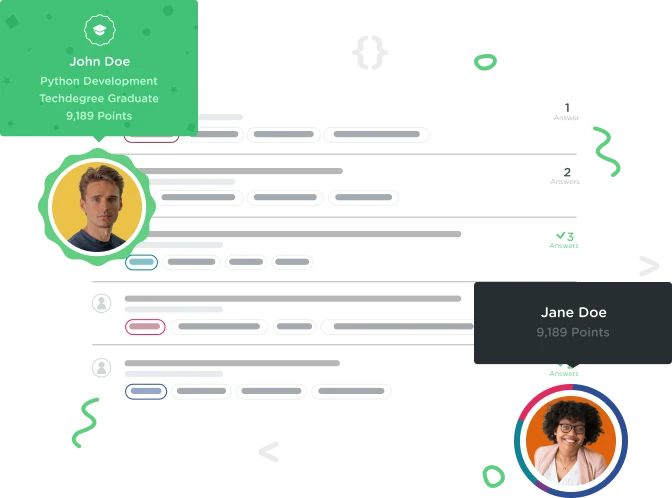

Jackson Monk
4,527 PointsThis question is very vague in some regards, I was hoping someone might be able to clear it up for me?
Does this objective want me to erase fullName completely? Does it want me to make them properties or variables? I doubt it's variables because it says erase 'var'. I have a lot of problems with this one
var contact = {
fullName: function() {
var firstName = "Andrew";
var lastName = "Chalkley";
console.log(firstName + " " + lastName);
}
}
3 Answers
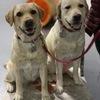
Alex Koumparos
Python Development Techdegree Student 36,887 PointsHi Jackson,
No, it doesn't want you to remove the fullName method completely, it says:
remove their variable declarations from the fullName method.
If you remove the variable declarations from the method then you will still have something in the method. Sure enough:
Don't do anything to the console.log() call right now.
So console.log()
will still be in the method after you remove the variables from the method.
So what do you do with the variables? You need to add firstName
and lastName
as properties in the object. Remember that a JavaScript object is of the form:
{
key: value,
another_key: another_value
}
Your keys are the variable names you removed from the method, and the values are the values that were in those variables.
Hope that's clear
Alex
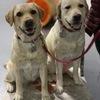
Alex Koumparos
Python Development Techdegree Student 36,887 PointsHi Jackson,
You've removed the letters 'var' but you haven't removed firstName
or lastName
from the function. If you look at line 2 of your code the opening brace at the end of the line marks where your function body begins. It runs to the corresponding closing brace on line 6. firstName
and lastName
, being on lines 3 and 4 respectively, are still in the function body.
Also note that they still end with semicolons (;
), which is correct for a JavaScript expression, but these are not expressions, they are elements in a JavaScript object, so should be terminated with a comma (,
) like I showed above.
Once you fix those two issues, your code will pass.
I think you are maybe getting confused by the fact that one of the items in the object is a function. Try not to think of it as a function and simply think of it as just another key value pair in the object. For example, suppose fullName was just a string instead of a function, the object would currently look like this:
var contact = {
fullName: "Some String"
}
You can add more items into a JavaScript object, either before or after the existing items, and it will look more like this:
var contact = {
some_value_I_added: 12345,
another_value: "This is a string",
fullName: "Some String",
yet_another_value: 3.14
}
Now let's go back to having fullName's value be a function, we would see something like this:
var contact = {
some_value_I_added: 12345,
another_value: "This is a string",
fullName: function() {
console.log("This is all that should be in the function");
},
yet_another_value: 3.14
}
Observe that the function is just another value in the object. Note also that in this example, because fullName
is not the last item in the object, it is terminated with a comma.
Hopefully this clears things up for you
Cheers
Alex

Jackson Monk
4,527 PointsThanks so much Alex, sorry to keep you here but the next question is pretty vague too. It says: 'Finally, finish off the code to correctly access the properties on the "contact" with the "this" keyword.'
My code:
var contact = {
fullName: function() {
console.log(firstName + " " + lastName);
},
firstName: "Andrew",
lastName: "Chalkley"
this.fullName;
this.firstName;
this.lastName;
}
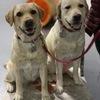
Alex Koumparos
Python Development Techdegree Student 36,887 PointsHi Jackson,
The question doesn't want you to add any new lines, instead it wants you to tweak the function inside contact
so that it is referring to the variables inside the object (basically, you just need to make a couple of small changes to your console.log
statement).
Cheers
Alex
Jackson Monk
4,527 PointsJackson Monk
4,527 PointsI think I have tried everything you told me, but it doesn't work. Here is what I did