Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial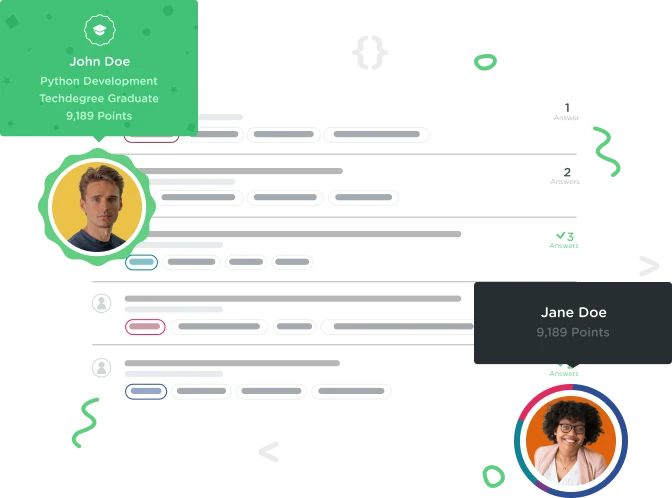

Nicholas Lee
1,098 PointsThis works in intelliJ but not in the brower. Please help
def covers(x): courselist=[] for k, values in COURSES.items(): if x.issubset(values): courselist.append(k) return courselist
COURSES = {
"Python Basics": {"Python", "functions", "variables",
"booleans", "integers", "floats",
"arrays", "strings", "exceptions",
"conditions", "input", "loops"},
"Java Basics": {"Java", "strings", "variables",
"input", "exceptions", "integers",
"booleans", "loops"},
"PHP Basics": {"PHP", "variables", "conditions",
"integers", "floats", "strings",
"booleans", "HTML"},
"Ruby Basics": {"Ruby", "strings", "floats",
"integers", "conditions",
"functions", "input"}
}
def covers(x):
courselist=[]
for k, values in COURSES.items():
if x.issubset(values):
courselist.append(k)
return courselist
3 Answers
William Li
Courses Plus Student 26,868 PointsAre you in part1? Part1 is looking for values overlap between x
& COURSES
. Your code is mostly correct, but you need to change the .issubset
into .intersectionn
method. That should fix the prob.
Also, you can simplify the code by making use of Python's list comprehension.
def covers(x):
return [key for key in COURSES if x.intersection(COURSES[key])]
That's another way to solve part 1. hope that helps, happy coding.

Nicholas Lee
1,098 PointsThanks William for your quick reply. Appreciate the tip! List comprehension is a cool feature. But is this generally a good practice? I am not sure if it would affect things like refactoring and of course when passing on your scripts forward to another programmer. Is this an explicit/implicit methodology question?
William Li
Courses Plus Student 26,868 PointsFor this particular problem, list comprehension approach is better. But not because it's a one-liner, your code gets better performance by using list comprehension because it doesn't need to load & call the append()
function each time the conditional is true. If COURSES happens to be a large dataset, the performance difference becomes very apparent. Python has many functional tools built-in, choosing the right tools for the job is the key to writing clean code.

Nicholas Lee
1,098 Pointsahh, i get it. Thanks William!