Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial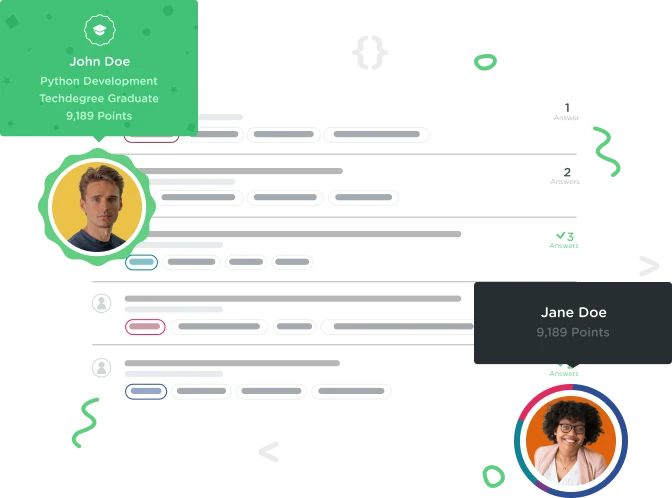

Cameron Wood
4,840 PointsThis works perfectly fine in my IDLE but not here...someone please help!
Create a function named stringcases that takes a single string but returns a tuple of different string formats. The formats should be: All uppercase All lowercase Titlecased (first letter of each word is capitalized) Reversed There are str methods for all but the last one.
YES. I understand I can use .title and .capitalize but that's not my question. My question is why isn't this working on the website but seems to be fine in IDLE.
def stringcases(s):
formats = []
first = ''
second = ''
for i in s:
first += i.upper()
second += i.lower()
formats.append(first)
formats.append(second)
second = second[0].upper() +second[1:]
formats.append(second)
s = s[::-1]
formats.append(s)
new = tuple(formats)
return new
1 Answer

andren
28,558 PointsThe issue is that your function does not really title-case the string. As the instructions state title-case is where the first letter of every word is capitalized. Your code capitalizes the first letter of the string, which will work fine if there is only one word passed in, but won't work if a sentence is passed in.
For example if I pass in the string "hello, world!" this is the result that should be returned by the function:
('HELLO, WORLD!', 'hello, world!', 'Hello, World!', '!dlrow ,olleh')
And here is the result your function returns:
('HELLO, WORLD!', 'hello, world!', 'Hello, world!', '!dlrow ,olleh')
Notice the lack of capitalization of the word "world" in the third string. That is the issue with your code.
Properly title-casing a string manually is a somewhat cumbersome process, which is why the title
method was added to python in the first place. If you make use of the built-in formatting function then you can solve the challenge with a single line of code:
def stringcases(s):
return (s.upper(), s.lower(), s.title(), s[::-1])