Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial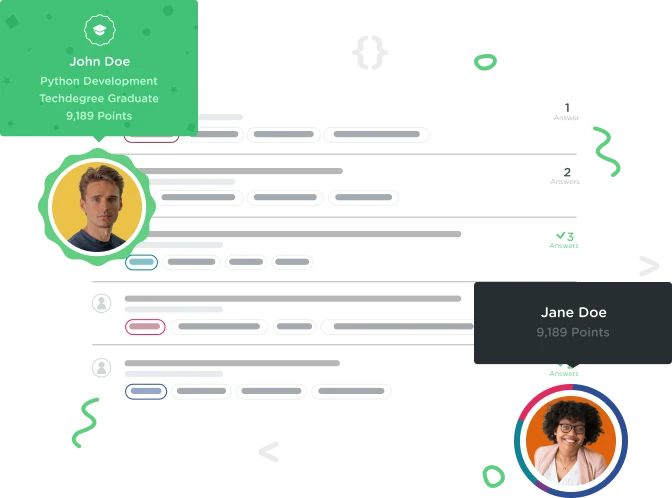
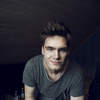
David Karlsson
4,180 Pointsthis.sides = sides?
Hey, I solved it basically the same way Andrew did, with the exception of the title of my question. I didn't add this.sides = sides; But I still get the same result. Why is it that we add that line? sides is the same anyways since we pass it into the constructor in the first place?
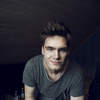
David Karlsson
4,180 PointsI can't seem to figure out how to write a reply to someone who has given an answer. However, I have already done the prototype challenges and I'm at the Playlist Project now. And I still don't know why he had to write out "this.sides = sides;",
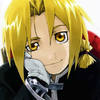
Gabriel D. Celery
13,810 PointsThen try to figure out why this code doesn't work
function Dice(sides) {
this.sides = sides;
}
Dice.prototype.roll = function () {
var randomNumber = Math.floor(Math.random() * sides) + 1;
return randomNumber;
}
var dice = new Dice(6);
console.log(dice.roll());
While this works
function Dice(sides) {
this.sides = sides;
}
Dice.prototype.roll = function () {
var randomNumber = Math.floor(Math.random() * this.sides) + 1;
return randomNumber;
}
var dice = new Dice(6);
console.log(dice.roll());
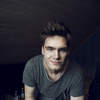
David Karlsson
4,180 PointsHm, so the value of the parameter isn't saved in the constructor if I don't type in this.(param)?
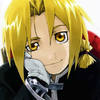
Gabriel D. Celery
13,810 PointsUhhh. The forum is not suited to explain how the "this" keyword works. I recommend this article: http://javascriptissexy.com/understand-javascripts-this-with-clarity-and-master-it/
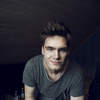
David Karlsson
4,180 PointsOkay, thanks. I understand how "this" works, I'm just not certain as to why it is needed here.
2 Answers

Fredrick Rogers
26,349 Pointsthis.sides = sides allows you to use the value of the "sides" variable outside of the constructor function. The sides property that is passed into the constructor function is only available inside that function. Once the function is finished, the variable is gone. The reason is due to a larger discussion on variable scopes that I think is addressed in the javascript bascis video series.
Check out the code below for an example.
function DiceWithoutThis(sides) {
// No this.sides = sides;
// Code removed for brevity
}
function DiceWithThis(sides) {
this.sides = sides;
// Code removed for brevity
}
var diceWithoutThis = new DiceWithoutThis(6);
var diceWithThis = new DiceWithThis(6);
console.log(diceWithoutThis.sides); // Returns undefined. The sides property is never set therefore it does not exist.
console.log(diceWithThis.sides); // Returns 6. The sides property was set.
The key here is to be able to access the 'sides' property outside of the constructor function.
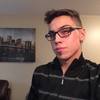
Josh Trill
4,867 PointsI may be wrong here, but when we are using 'this' as in Gabriel's example the first time { 'this.sides = sides' } can be thought of as setting a key/value, just like with an object, and the second instance of this.sides would be calling it after the argument has been sent.
Like I said, that may be inaccurate, but that's how I saw it working.
Gabriel D. Celery
13,810 PointsGabriel D. Celery
13,810 PointsI assume your code looks something like this.
There is nothing wrong with your solution, but later during this course when you will learn about prototypes you will understand why Andrew was using "this.sides".