Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial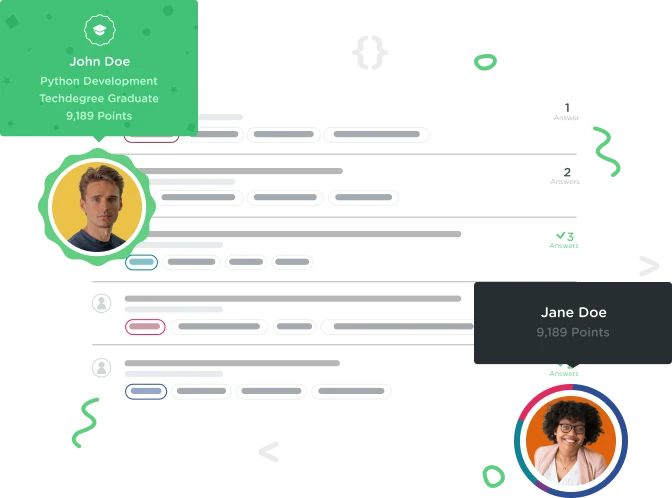
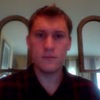
Casey Huckel
Courses Plus Student 4,257 PointsThought this would pass. Can someone explain why it doesn't?
Code:
package com.example;
import java.util.Date;
public class BlogPost implements Comparable{
private String mAuthor;
private String mTitle;
private String mBody;
private String mCategory;
private Date mCreationDate;
public BlogPost(String author, String title, String body, String category, Date creationDate) {
mAuthor = author;
mTitle = title;
mBody = body;
mCategory = category;
mCreationDate = creationDate;
}
public String[] getWords() {
return mBody.split("\\s+");
}
public String getAuthor() {
return mAuthor;
}
public String getTitle() {
return mTitle;
}
@Override
public int compareTo(Object obj) {
if(equals(obj)) {
return 0;
}
int compareDate = mCreationDate.compareTo(obj.mCreationDate);
return compareDate;
}
public String getBody() {
return mBody;
}
public String getCategory() {
return mCategory;
}
public Date getCreationDate() {
return mCreationDate;
}
}
[MOD: added ```java markdown formatting -cf]
6 Answers
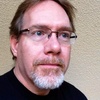
Chris Freeman
Treehouse Moderator 68,423 PointsYou are missing the casting of obj
to BlogPost
so it can be properly compared:
public int compareTo(Object obj) {
// Task 2 of 3
// In this step, cast the Object passed to the compareTo method
// to a com.example.BlogPost. Check for equality first, if they
// are equal return 0. Leave the default return of 1 in place
// for this step.
BlogPost blogpost = (BlogPost) obj;
if (equals(blogpost)) {
return 0;
}
// Task 3 of 3
// Alright, now let's order the blog post's creation date
// chronologically, oldest first.
// NOTE: The app where this class is being used ensures that no
// two blog posts are posted at the same time, so you do not need
// to worry about any edge cases.
return mCreationDate.compareTo(blogpost.mCreationDate);
}
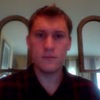
Casey Huckel
Courses Plus Student 4,257 PointsI added BlogPost blogpost = (BlogPost) obj; and it passed. Thanks Chris
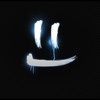
Grigorij Schleifer
10,365 PointsHi Casey,
inside your compareTo() method obj need to be downcasted to String or BlogPost. After that you need to compare the class itself with a String or a BlogPost using equals.
Grigorij
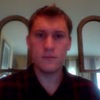
Casey Huckel
Courses Plus Student 4,257 PointsGrigorij, Can you show me what you mean?
Chris, I've tried this but I get this error:
./com/example/BlogPost.java:38: error: cannot find symbol
return mCreationDate.compareTo(blogpost.mCreationDate);
^
symbol: variable blogpost
location: class BlogPost
1 error
[MOD: added ```bash markdown formatting -cf]
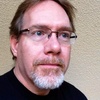
Chris Freeman
Treehouse Moderator 68,423 Pointsblogpost
should be defined if you've added the line:
BlogPost blogpost = (BlogPost) obj;
Can you post your current full compareTo
method?
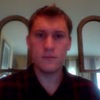
Casey Huckel
Courses Plus Student 4,257 PointsThe ^ should be pointing at the b in blogpost

Mehmet Arabaci
4,432 PointsHi could someone explain why the code below does not work
@Override
public int compareTo(Object obj){
BlogPost bp = (BlogPost) obj;
if (bp.equals(obj)) {
return 0;
}
return 1;
}
but this code does
@Override
public int compareTo(Object obj){
BlogPost bp = (BlogPost) obj;
if (equals(obj)) {
return 0;
}
return 1;
}
I cant seem to understand what
if (equals(obj))
means. To me the code
if (bp.equals(obj))
makes more sense, clearly comparing bp to obj. but what is equals(obj) comparing to? Where is the first half of that statement.