Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial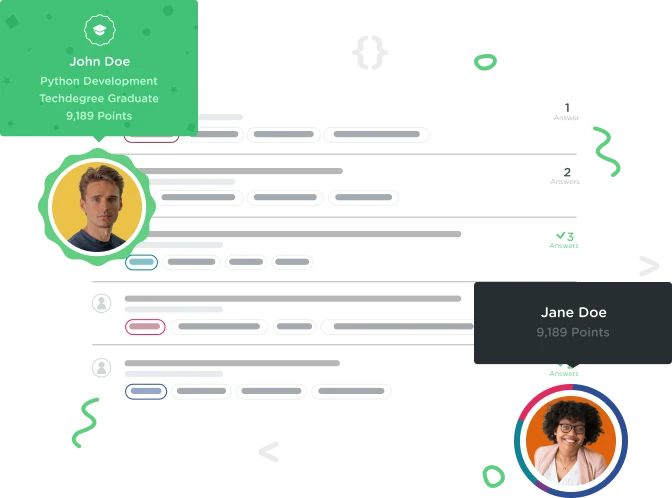

Tomas Rinblad
Full Stack JavaScript Techdegree Student 12,427 PointsThoughts about my code?
// questions
var question = [ ["Where do i live", "karlskrona"], ["Did i grow up here", "yes"], ["True or false, do i own a cat", "true"] ];
// counter var right=0;
// fthe asking function. function printAnswer ( quest ) { var listHTML = "<ul>"; var wrong = "<ul>"; for (var i=0; i<quest.length; i+=1) { var answer = prompt(quest[i][0]); if (answer === quest[i][1]) { listHTML += "<li>" + quest[i][0] + "</li>"; right += 1; }else{ wrong += "<li>" + quest[i][0] + "</li>"; } } listHTML += "</ul>"; wrong += "</ul>"; document.write("<p> You got " + right + " correct answers"); document.write("<p> You got the following correct answers: </p>" ); print(listHTML); document.write("<p> You got the following wrong answers: </p>" ); print(wrong);
// code for writing on page. } function print(message) { document.write(message); }
printAnswer(question);
i am sure it can be better coded somewhere but i am actually proud of this :) Started with Javascript (and have 0 experience with coding) abotu 3 days ago :)
question: how can i add my whole code without it being disformed? i have seen people add the code directly from the codepage?
1 Answer

Thomas Nilsen
14,957 PointsNothing really wrong with your code.
I've rewritten it a little bit just to show one way you could write this
Maybe there's a few concepts you haven't learned yet, but this is a great to way to do just that.
// questions
//Changed it from 2D array to an array of objects.
var questions = [
{ q: "Where do i live", a: "karlskrona" },
{ q: "Did i grow up here", a: "yes" },
{ q: "True or false, do i own a cat", a: "true" }
];
function print(message) { document.write(message); }
//Function that uses closure concept to store an object
//with right/wrong answers
//http://stackoverflow.com/questions/111102/how-do-javascript-closures-work
function createList() {
var result = {true: [], false: []};
return function(q, isCorrect) {
result[isCorrect].push(q);
return result;
}
}
//Outputs the HTML based on the result
//How does .map-function work?
//https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/map?v=control
function createHTML(result) {
var listCorrect = "<ul>" + (result[true].map(q => "<li> " + q + "</li>")) + "</ul>"
var listWrong = "<ul>" + (result[false].map(q => "<li> " + q + "</li>")) + "</ul>"
print("<p> You got " + result[true].length + " answer(s) correct </p>");
print("<p> You got the following correct answers: </p>");
print(listCorrect);
print("<p> You got the following wrong answers: </p>");
print(listWrong);
}
//Loops through the questions
//How does forEach work?
//https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/forEach?v=control
function askQuestions () {
var list = createList();
var result = {};
questions.forEach(obj => {
var answer = prompt(obj.q.toLowerCase());
result = list(obj.q, obj.a == answer);
});
createHTML(result);
}
askQuestions();
Tomas Rinblad
Full Stack JavaScript Techdegree Student 12,427 PointsTomas Rinblad
Full Stack JavaScript Techdegree Student 12,427 PointsFound a way to make the code better: function printAnswer ( quest ) { var listHTML = "<ul>"; var wrong = "<ul>"; for (var i=0; i<quest.length; i+=1) { var answer = prompt(quest[i][0]); if (answer === quest[i][1]) { listHTML += "<li>" + quest[i][0] + "</li>"; right += 1; }else{ wrong += "<li>" + quest[i][0] + "</li>"; } } listHTML += "</ul>"; wrong += "</ul>"; document.write("<p> You got " + right + " correct answers"); document.write("<p> You got the following correct answers: </p>" ); print(listHTML); if (right < 3) { document.write("<p> You got the following wrong answers: </p>" ); print(wrong); }