Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial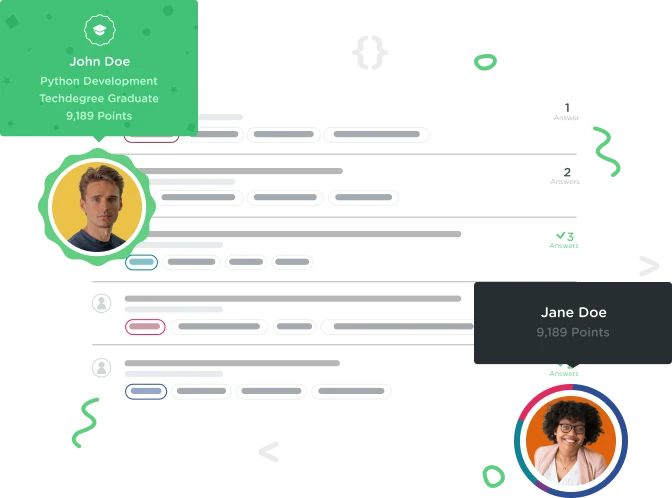

Patrick Munemo
14,892 PointsThreads and services
Finally, update MainActivity's 'latitude' and 'longitude' fields with the correct coordinates when the user taps the Button.
public class GeolocationService extends Service {
private IBinder mBinder = new LocalBinder();
@Override
public IBinder onBind(Intent intent) {
return mBinder;
}
public class LocalBinder extends Binder{
public GeolocationService getService(){
return GeolocationService.this;
}
}
//Client methods
public int getLatitude() { return getLat(); }
public int getLongitude() { return getLng(); }
}
public class MainActivity extends Activity {
private GeolocationService mGeolocationService;
int latitude;
int longitude;
boolean isBound;
Button updateLocationButton;
ServiceConnection serviceConnection = new ServiceConnection(){
public void onServiceConnected(ComponentName name, IBinder binder){
isBound = true;
GeolocationService.LocalBinder localBinder = (GeolocationService.LocalBinder) binder;
mGeolocationService = localBinder.getService();
}
public void onServiceDisconnected(ComponentName name){
isBound = false;
}
};
@Override
public void onCreate(Bundle savedInstanceState) {
setContentView(R.layout.activity_main);
updateLocationButton = (Button) findViewById(R.id.update_button);
updateLocationButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v){
if (isBound){
if (mGeolocationService.latitude()){
updateLocationButton.setText("Latitude");
}else{
mGeolocationService.longitude();
updateLocationButton.setText("Longitude");
}
}
}
});
}
@Override
public void onStart() {
Intent i = new Intent(this, GeolocationService.class);
bindService(i, serviceConnection, Context.BIND_AUTO_CREATE);
}
@Override
public void onStop() {
if (isBound) {
unbindService(serviceConnection);
isBound = false;
}
}
}
1 Answer
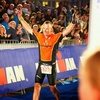
Steve Hunter
57,712 PointsHi there,
I'm out at the moment but I think you want to be using the two getter methods:
public int getLatitude() { return getLat(); }
public int getLongitude() { return getLng(); }
which are in GeolocationService
. Use those to set the latitude
and longitude
member variables of your activity.
Something like this inside onClick()
. There's no need to distinguish between lat & long with an if statement - there's only one button!
latitude = mGeolocationService.getLatitude();
longitude = mGeolocationService.getLongitude();
Try using the above inside onClick instead of where you have you if statement. Keep the isBound
if statement, though. As I said, I'm out at the moment but I'll get back to you with more when I am home again.
Steve.

Patrick Munemo
14,892 PointsYou are a real life saver. Thanks a million times
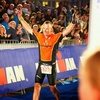
Steve Hunter
57,712 PointsIt worked, then! ?
Patrick Munemo
14,892 PointsPatrick Munemo
14,892 PointsSteve Hunter , please help am stuck here