Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial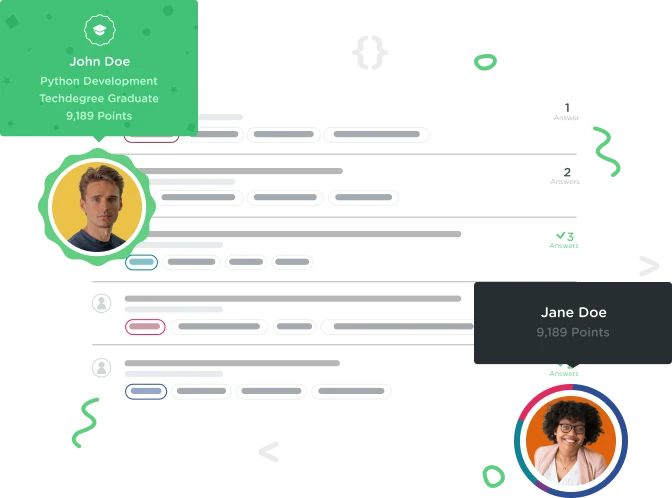

Anthony c
20,907 PointsThree steps to modularize?
We start with
function(){
}
Then we add (); to the end of the function to make it call:
function(){
}();
then we put that inside a variable with parenthesis
var myVariable = ();
Which results in the below (a variable holding an IIFE):
var myVariable = (function(){
}(););
and now we have module? Or at least one potential (and basic) pattern for a module?
2 Answers
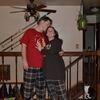
Julie Myers
7,627 PointsWhat you have is the super duper basics of the model pattern. There are different flavors of the module pattern. For example:
var yourModule = (function(){
var universe = "The Great Beyond";
var yourPublicFunction = function(){
return 'Hello World!';
};
return {
universal: universe,
yourPublicFunction: yourPublicFunction
};
})();
/*
When the object literal was returned it was returned into the yourModule
variable. What this means is that we’ll have a reference to the object
returned via the yourModule variable. We can execute ‘yourPublicFunction’
as follows:
*/
console.log(yourModule.yourPublicFunction()); // returns 'Hello World!'
console.log(yourModule.universal); // "The Great Beyond'"
/*
Remember, we can only access the ‘yourPublicFunction’ function object via
yourModule. We can’t access or invoke the ‘yourPublicFunction’ function object
directly. For example:
*/
console.log(yourPublicFunction()); // JavaScript error!
When all is said and done the module patter is essentially an IIFE assigned to a variable.
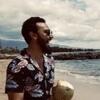
Ali Raza
13,633 PointsThere is a great article:
On Javascript Modular Patterns I would recommend a read. It covers the basics and some advance concepts.
Michael Kellogg
774 PointsMichael Kellogg
774 PointsHonestly, this video was the most confusing one I've watched on Treehouse. He jumps into things without showing how he sets them up (e.g. importing "underscore"), and skips onto a new pattern, referring to a previous pattern as something different, several times. What, for example, is the difference between "the module pattern" and "the module exports pattern" ? And that syntax with the double pipes (aka "or")? I didn't understand that at all, how it works or what it was supposed to be used for. Then he jumps into this "sub" pattern which just made it worse. I watched this video several times and it never got better. Moving on.