Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial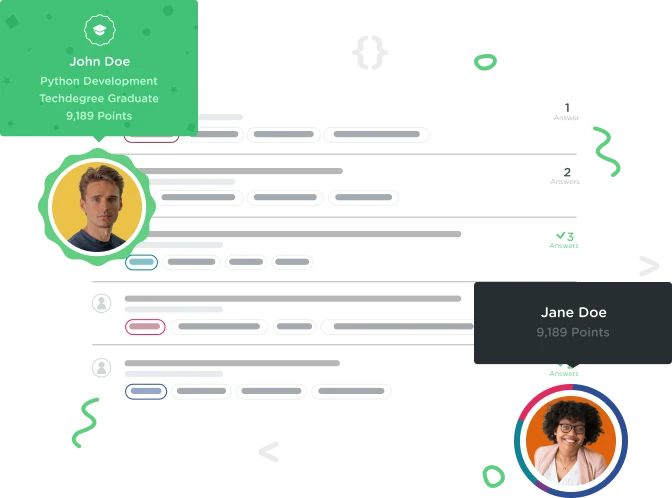

Thomas Katalenas
11,033 PointsThrowing an exception or throwing up....
what is the syntax or example of a throw method
throw(inserthere){};
? is that right.
public class GoKart {
public static final int MAX_BARS = 8;
private String mColor;
private int mBarsCount;
public GoKart(String color) {
mColor = color;
mBarsCount = 0;
}
public String getColor() {
return mColor;
}
public void drive() {
drive(1);
}
public void drive(int laps) {
// Other driving code omitted for clarity purposes
throw(IllegalArgumentException){
laps != 0
}
mBarsCount -= laps;
}
public void charge() {
while (!isFullyCharged()) {
mBarsCount++;
}
}
public boolean isBatteryEmpty() {
return mBarsCount == 0;
}
public boolean isFullyCharged() {
return mBarsCount == MAX_BARS;
}
}
7 Answers
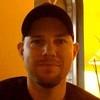
Jeremy Hill
29,567 PointsInside your drive(int laps) method you need to add an if statement that checks if the laps is more than the remaining battery. if the laps > mBarsCount you will need to add a throw exception; it should look like this:
throw new IllegalArgumentException("Not enough battery remains");

Thomas Katalenas
11,033 Pointsis there a difference between throw and throws?

Thomas Katalenas
11,033 Pointscant you do that with a try/catch block.
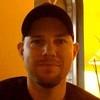
Jeremy Hill
29,567 PointsYou could but the assignment doesn't call for us to do it that way.

Thomas Katalenas
11,033 Pointsif(mBarsCount < laps){
throw new IllegalArgumentException("Not enough battery remains.");
}
so that did work!!
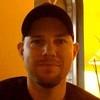
Jeremy Hill
29,567 PointsGood deal!

Thomas Katalenas
11,033 Pointswhat about this ? try/catch block doesn't work can you show me how it would work?
public void drive(int laps) throws IllegalArgumentException {
// Other driving code omitted for clarity purposes
mBarsCount -= laps;
try{
if (mBarsCount < laps);
} catch (IllegalArgumentException e){
throw new IllegalArgumentException("Not enough battery remains.");
}
}
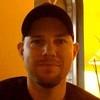
Jeremy Hill
29,567 PointsI actually misled you. the try/catch is more for runtime exceptions (errors). We actually threw an exception ourselves because the battery cannot have a negative charge; java doesn't think that way so it would have a negative charge which in reality isn't possible, so we had to send an error message to the user that they could not drive that many laps. We don't even have to use an exception we could have done something like this:
if(mBarsCount < laps){
System.out.println("Not enough battery remains");
System.exit(0);
}
It may not pass in the assignment because they are wanting us to throw an exception.
now a good reason to use a try/catch block would be to prevent someone from passing a letter as an argument when it is supposed to be a int (integer).
Like this:
try{
public void drive(int laps){
if(mBarsCount < laps)
throw new IllegalArgumentException("Not enough battery remains");
}
}catch(Exception e){
System.err.println("You can not pass letters as arguments!");
}

Thomas Katalenas
11,033 PointsIm going to continue this thread for the next week this is a topic for my notes. :)

Thomas Katalenas
11,033 Pointshttps://docs.oracle.com/javase/tutorial/essential/exceptions/ is what I am using to find exceptions to pass.