Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial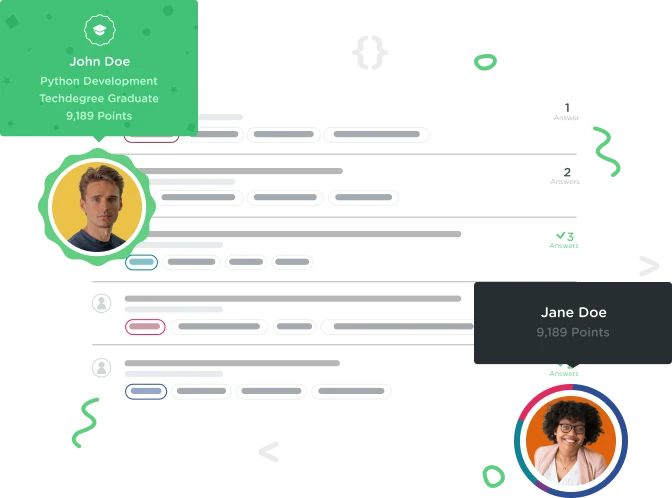

Heather Mathewson
10,912 PointsThrowing errors in Swift
I am honestly so lost. There are too many steps in step one, so I have no idea where i am screwing things up. This is the fifth version I tried so I am asking you guys: In the editor, you have a struct named Parser whose job is to deconstruct information from the web. The incoming data can be nil, keys may not exist, and values might be nil as well. In this task, complete the body of the parse function. If the data is nil, throw the EmptyDictionary error. If the key "someKey" doesn't exist throw the InvalidKey error. Hint: To get a list of keys in a dictionary you can use the keys property which returns an array. Use the contains method on it to check if the value exists in the array
enum ParserError: ErrorType {
case EmptyDictionary
case InvalidKey
}
struct Parser {
var data: [String : String?]?
func parse() throws {
throw ParserError.EmptyDictionary
throw ParseError.InvaildKey
}
}
let data: [String : String?]? = ["someKey": nil]
let parser = Parser(data: data)
4 Answers
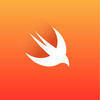
Steven Deutsch
21,046 PointsHey Heather Mathewson,
You can take a look at this post I just made on this challenge if you're looking for an explanation.
https://teamtreehouse.com/community/error-handling-2
Good Luck!
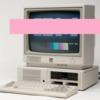
kols
27,007 PointsUnfortunately, none of the answers I found on the help forums passed this challenge as they were posted a while back. I'm adding the answer that worked for me below (Swift 4):
enum ParserError: Error {
case emptyDictionary
case invalidKey
}
struct Parser {
var data: [String : String?]?
func parse() throws {
guard data != nil else {
throw ParserError.emptyDictionary
}
guard data?.keys.contains("someKey") == true else {
throw ParserError.invalidKey
}
}
}
let data: [String : String?]? = ["someKey": nil]
let parser = Parser(data: data)
do {
try parser.parse()
} catch ParserError.emptyDictionary {
print("The dictionary is empty.")
} catch ParserError.invalidKey {
print("The key 'someKey' does not exist.")
}
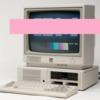
kols
27,007 PointsAlternative Solution >>> I'm working back through these code challenges as a refresher, and I came up with a different answer this time, so I'm providing it below as an alternative for anyone who struggles with this challenge.
Note: At the time of this posting [May 2018], both are still accepted as valid solutions; however, I prefer my previous answer (^^) as it's just a little cleaner in my opinion and demonstrates a better grasp of these concepts.
enum ParserError: Error {
case emptyDictionary
case invalidKey
}
struct Parser {
var data: [String : String?]?
func parse() throws {
guard let webData = data else {
throw ParserError.emptyDictionary
}
if webData.keys.contains("someKey") == false {
throw ParserError.invalidKey
}
}
}
let data: [String : String?]? = ["someKey": nil]
let parser = Parser(data: data)
do {
try parser.parse()
print("Congratulations; no errors encountered.")
} catch ParserError.emptyDictionary {
print("Danger, Wilma Robinson: This dictionary is empty af.")
} catch ParserError.invalidKey {
print("Danger, Wilma Robinson: This key doesn't even exist.")
}

Heather Mathewson
10,912 PointsNot sure how I got here after 3 hours of furiously googling and asking people IRL, but this seems to work: enum ParserError: ErrorType { case EmptyDictionary case InvalidKey }
struct Parser { var data: [String : String?]?
func parse() throws { if let dict = data{ if dict["someKey"] == nil { throw ParserError.InvalidKey}} else { throw ParserError.EmptyDictionary}
} }
let data: [String : String?]? = ["someKey": nil] let parser = Parser(data: data)
Would still love someone to walk it through if they can.
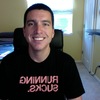
Stephen Printup
UX Design Techdegree Student 45,252 PointsHi Heather! It's Stephen from Greg's class!
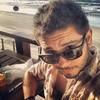
james rochabrun
Courses Plus Student 22,726 Pointsenum ParserError: Error { case emptyDictionary case invalidKey }
struct Parser { var data: [String : String?]?
func parse() throws {
guard (data?["someKey"]) != nil else {
throw ParserError.invalidKey
}
guard (data?.keys) != nil else {
throw ParserError.emptyDictionary
}
}
}
let data: [String : String?]? = ["someKey": nil] let parser = Parser(data: data)