Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial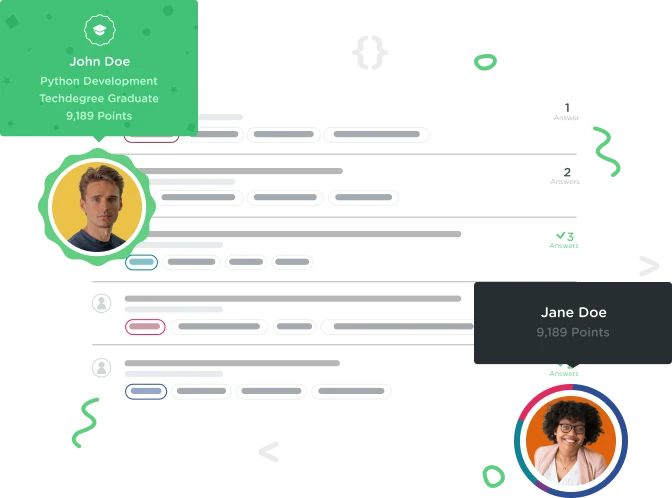

Neil Bircumshaw
Full Stack JavaScript Techdegree Student 14,597 Pointstic tac toe game - on hover event.
Trying to get the boxes to show a background image when they are hovered over (needs to be done in JS) but instead when I hover over one box, the image appears on all the boxes! I jsut want it on one of the boxes. So I tried to implement event bubbling and then target only LI items but I may have written it wrong, any pointers?
here's my code:
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Tic Tac Toe</title>
<link href='https://fonts.googleapis.com/css?family=Montserrat:400,700' rel='stylesheet' type='text/css'>
<link rel="stylesheet" href="css/normalize.css">
<link rel="stylesheet" href="css/style.css">
</head>
<body>
<div class="board" id="board">
<header>
<h1>Tic Tac Toe</h1>
<ul>
<li class="players active" id="player1">
<svg xmlns="http://www.w3.org/2000/svg" width="42" height="42" viewBox="0 0 42 42" version="1.1"><g stroke="none" stroke-width="1" fill="none" fill-rule="evenodd"><g transform="translate(-200.000000, -60.000000)" fill="#000000"><g transform="translate(200.000000, 60.000000)"><path d="M21 36.6L21 36.6C29.6 36.6 36.6 29.6 36.6 21 36.6 12.4 29.6 5.4 21 5.4 12.4 5.4 5.4 12.4 5.4 21 5.4 29.6 12.4 36.6 21 36.6L21 36.6ZM21 42L21 42C9.4 42 0 32.6 0 21 0 9.4 9.4 0 21 0 32.6 0 42 9.4 42 21 42 32.6 32.6 42 21 42L21 42Z"/></g></g></g></svg>
</li>
<li class="players " id="player2">
<svg xmlns="http://www.w3.org/2000/svg" width="42" height="43" viewBox="0 0 42 43" version="1.1"><g stroke="none" stroke-width="1" fill="none" fill-rule="evenodd"><g transform="translate(-718.000000, -60.000000)" fill="#000000"><g transform="translate(739.500000, 81.500000) rotate(-45.000000) translate(-739.500000, -81.500000) translate(712.000000, 54.000000)"><path d="M30 30.1L30 52.5C30 53.6 29.1 54.5 28 54.5L25.5 54.5C24.4 54.5 23.5 53.6 23.5 52.5L23.5 30.1 2 30.1C0.9 30.1 0 29.2 0 28.1L0 25.6C0 24.5 0.9 23.6 2 23.6L23.5 23.6 23.5 2.1C23.5 1 24.4 0.1 25.5 0.1L28 0.1C29.1 0.1 30 1 30 2.1L30 23.6 52.4 23.6C53.5 23.6 54.4 24.5 54.4 25.6L54.4 28.1C54.4 29.2 53.5 30.1 52.4 30.1L30 30.1Z"/></g></g></g></svg>
</li>
</ul>
</header>
<ul class="boxes">
<li class="box"></li>
<li class="box"></li>
<li class="box"></li>
<li class="box"></li>
<li class="box"></li>
<li class="box"></li>
<li class="box"></li>
<li class="box"></li>
<li class="box"></li>
</ul>
</div>
<script src="app.js"></script>
</body>
</html>
JS
const boxes = document.querySelector(".boxes");
const box = boxes.children;
const player1 = document.getElementById("player1");
boxes.addEventListener("mouseover", (e) => {
if (e.target.tagName == "LI"){
for (let i = 0; i < box.length; i++){
box[i].style.backgroundImage = 'url(img/o.svg)';
}
}
})
1 Answer
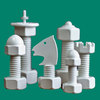
Steven Parker
231,271 PointsYou won't need a loop to change only one box.
Your event handler and filter are fine, but then you loop through and update every child element. Instead, just change only the event target, now that your filter has identified it as one of the list items:
boxes.addEventListener("mouseover", e => {
if (e.target.tagName == "LI") {
e.target.style.backgroundImage = "url(img/o.svg)";
}
});
Neil Bircumshaw
Full Stack JavaScript Techdegree Student 14,597 PointsNeil Bircumshaw
Full Stack JavaScript Techdegree Student 14,597 PointsYou're a life saver and you explain things so easily, thank you! They should pay you at Treehouse for how much help you have given Steven.
Currently trying to get my head around constructor functions and prototypal inheritance in JS, I sort of understand the concept, but applying it to projects is what I find difficult, I think to myself where would a constructor function and the prototype method come in handy? Haha, just part of learning I suppose!
Don't mind if I ask a personal question do you? Was wondering how long you've done this for and does it become like second nature after a while?
Steven Parker
231,271 PointsSteven Parker
231,271 PointsI've dabbled with JavaScript for over a decade, and have used it seriously at work for about 3 years. I'm not sure it will ever be quite "second nature", particularly if it continues to evolve at the pace it has recently. But I've gotten much practice in referring to documentation!