Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial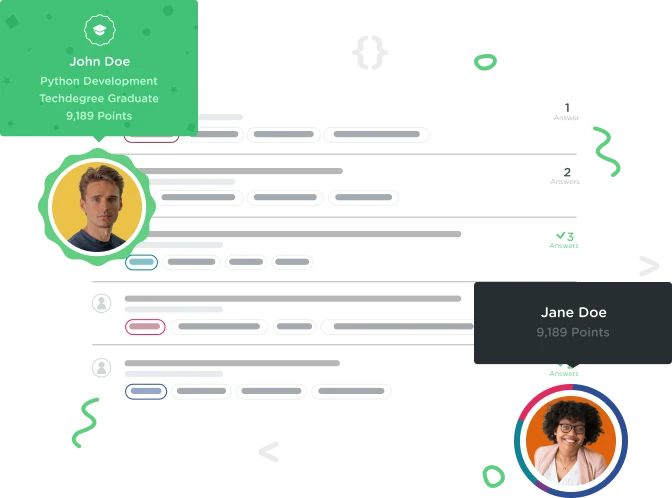

Kenneth Bagwell Jr
3,698 Pointstime delta coversion
how do I covert this time to a time delta
import datetime
def time_machine(num, "days"):
time =
starter = datetime.datetime(2015, 10, 21, 16, 29)
# Remember, you can't set "years" on a timedelta!
# Consider a year to be 365 days.
## Example
# time_machine(5, "minutes") => datetime(2015, 10, 21, 16, 34)
2 Answers
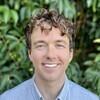
Asher Orr
Python Development Techdegree Graduate 9,409 PointsHi Kenneth!
The function, time_machine, should take an integer and a string of "minutes", "hours", "days", or "years". First:
def time_machine(num, "days"):
In your code above, the second parameter is literally the string "days". You want the parameter to represent any string that's passed into it- days, minutes, hours, or years. You should change that parameter to something generic, like:
def time_machine(num, time_unit):
Now, let's move on to the body of your function.
Your parameters are a number and a time unit that represent a timedelta (a gap in time.) When you click "Check Work," the Treehouse checker will pass in arguments like this:
def time_machine(5, "hours")
If you watch Kenneth's lesson on timedeltas, you'll see that you can add them to other datetime objects to get a new time.
For example:
now = datetime.datetime.now()
# >> now
datetime.datetime(2022, 7, 12, 12, 10, 31, 459833)
# here are what those numbers represent: year, month, day, hour, minute, second, microsecond.
new_object = now + datetime.timedelta(days=3)
# >> new_object
datetime.datetime(2022, 7, 15, 12, 10, 31, 459833)
#see the difference?
#we added 3 days to a datetime object (now)
#that's why the 12 (the third number in the parentheses, representing the day) became a 15.
The challenge asks to return a datetime object that is the timedelta's duration from the starter datetime.
Here's a simpler way to think about it:
Step 1: Convert your parameters to a datetime object that's a timedelta.
- Hint: you may need to use multiple if statements, since "minutes", "hours", "days", or "years" could be passed in for the time_unit parameter.
Step 2: Make a variable that stores an operation where you add that datetime object to the variable "starter".
- That's how you get the timedelta's duration from the starter datetime.
Step 3: Return that variable
I hope this helps. Let me know if you have questions!
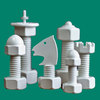
Steven Parker
231,269 PointsWhen you define a function, the parameter names should not have quotes around them (but when the function is called, it's OK for the argument to be a quoted literal string).
To make a timedelta using a num of units you would do something like mydelta = datetime.timedelta(units=num)
. But remember that timedelta doesn't recognise "years" for units, so you'll need to handle that specially.