Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial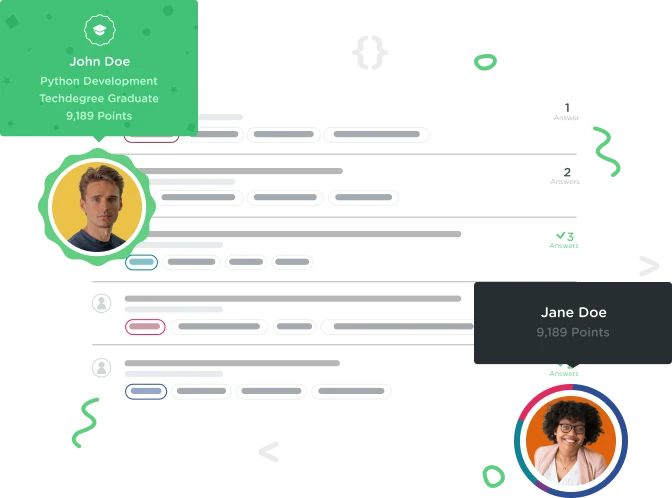

hubert chen
12,761 PointsTime quiz app
What is wrong with my code, it keeps saying there is a syntax error on the line with def init(self)
code:
import datetime
import random
from questions import Add, Multiply
class Quiz:
questions = []
answers = []
def __init__(self):
question_types = (Add, Multiply)
for _ in range(10):
num1 = random.randint(1, 10)
num2 = random.randint(1, 10)
question = random.choice(question_types)(num1, num2)
self.questions.append(question)
def take_quiz(self):
self.startime = datetime.datetime.now()
for question in self.questions:
self.answers.append(self.ask(question))
else:
self.end_time = datetime.datetime.now()
return self.summary
def ask(self, question):
correct = False
question_start = datetime.datetime.now()
answer = input(question.text + ' = ')
if answer == str(question.answer):
correct = True
question_end = datetime.datetime.now()
return correct, question_end - question_start
def total_correct(self):
total = 0
for answer in self.answers:
if answer[0]:
total += 1
return total
def summary(self):
print("You got {} out of {} right.".format(
self.total_correct(), len(self.questions)
))
print("It took you {} seconds total.".format(
(self.end_time-self.start_time).seconds
))
Quiz().take_quiz()
6 Answers
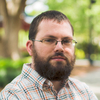
Kenneth Love
Treehouse Guest TeacherOK, here's what I've found so far:
-
return self.summary
needs()
on the end. - line 48 references
self.start_time
which doesn't exist, 'cause on line 20 you called itstartime
. Notice the misspelling and lack of underscore.
Once I fixed those things, it played fine and gave me the summary.
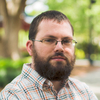
Kenneth Love
Treehouse Guest TeacherI don't actually see anything wrong with that code. Can you show me what's in questions.py
?

hubert chen
12,761 PointsI've updated my code.
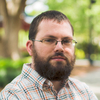
Kenneth Love
Treehouse Guest TeacherWell, if the new version is accurate, you have some indentation problems.
EDIT:
I copied the first part of your code, what you had originally posted, into Workspaces and had no problems (I adjusted the indentation but didn't change any code).

hubert chen
12,761 PointsI'm still getting syntax error when I put it the whole code, even when I changed the indent.

Jeremy Justus
2,910 PointsWhen I threw the code above into Workspaces, it refused to run until I I fixed the indentation of the take_quiz() method; I'm reasonably certain that's the culprit, at least with the current code not running at all.
I also noticed that the summary method isn't running, which may be because you defined start_time as startime up near the top. Even with that changed, the summary method didn't want to print while being returned. I changed it to a simple self.summary() call instead. When I changed that, the method runs, but it counts the number of wrong answers, not the correct ones. I'm not quite sure how to fix that, since I built my quiz quite a bit differently than Kenneth and used a number of global variables instead of methods like that (which means I was likely being un-pythonic, but I was feeling a bit lazy at the time).
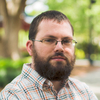
Kenneth Love
Treehouse Guest TeacherEww, don't use globals. They're so messy.

hubert chen
12,761 PointsThanks alot guys, I figured it out on my own just yesterday...took such a long time to find bugs.