Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial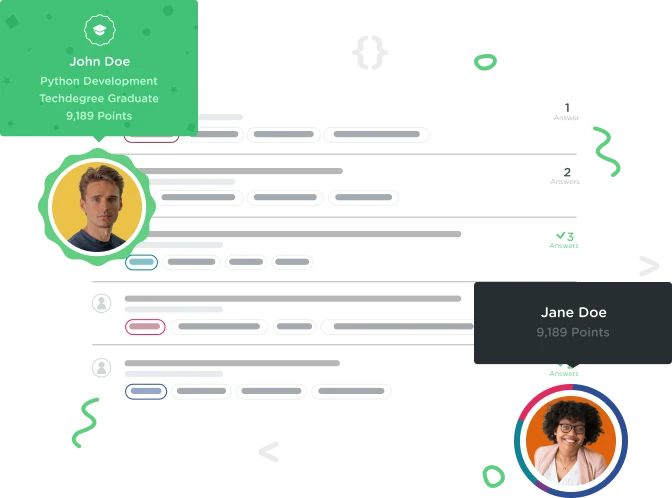

Ron Chan
10,987 Pointstimedelta input type
While attempting to solve the challenge, I passed in a string as an argument to the timedelta method and found it does not accept a string as an input. So I have 2 questions:
What exactly is the 'type' being passed in to the timedelta method?
Is there a way to parse the string into the type accepted by the method or is it necessary to use another method to achieve the same output required by the question?
def time_machine(time_int, int_string):
output = starter + datetime.timedelta("{}={}".format(int_string, time_int))
return output
1 Answer
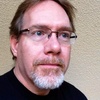
Chris Freeman
Treehouse Moderator 68,441 Pointsdatetime.timedelta()
takes numeric positional arguments or numerics as keyword arguments. The numerics can be integers or floats.
-
datetime.timedelta(1)
is one day -
datetime.timedelta(1, 2)
is one day, and 2 seconds -
datetime.timedelta(1, 2, 3)
is one day, 2 seconds, and 3 microseconds -
datetime.timedelta(days = 1)
is one day -
datetime.timedelta(0, 2)
is two seconds -
datetime.timedelta(seconds = 2)
is two seconds -
datetime.timedelta(0, 0, 3)
is three microseconds -
datetime.timedelta(microseconds = 3)
is three microseconds
More information at help(datetime.timedelta) or at timedelta
EDIT: One way to parse strings into your time_machine()
function is to define a dictionary to unpack in timedelta()
:
def time_machine(time_int, int_string):
kwargs = {time_int: float(int_string)}
output = starter + datetime.timedelta(**kwargs)
return output
EDIT #2: After running the challenge, I see that they toss "years" at you. Here is a version of the code above plus a check for "years":
import datetime
starter = datetime.datetime(2015, 10, 21, 16, 29)
# Remember, you can't set "years" on a timedelta!
# Consider a year to be 365 days.
## Example
# time_machine(5, "minutes") => datetime(2015, 10, 21, 16, 34)
def time_machine(tint, string):
if string == "years":
string = "days"
tint = tint * 365
kwargs = {string: tint}
return starter + datetime.timedelta(**kwargs)
Ron Chan
10,987 PointsRon Chan
10,987 PointsYou mentioned: datetime.timedelta(seconds = 2) is two seconds
So for instance int_string is defined as a string "seconds", and time_int is 2, how should I pass them into the timedelta method as an argument or arguments? I tried to do that with the code snippet above and apparently the format method turned everything into a string which the timedelta method does not accept.
Chris Freeman
Treehouse Moderator 68,441 PointsChris Freeman
Treehouse Moderator 68,441 PointsUpdated answer with
time_machine()
exampleRon Chan
10,987 PointsRon Chan
10,987 PointsThanks Chris, appreciate your help! It did not occur to me that the arguments for the timedelta method would be the same format as the way dictionaries are stored.
Chris Freeman
Treehouse Moderator 68,441 PointsChris Freeman
Treehouse Moderator 68,441 PointsMore precisely, it's not that the
timedelta()
function, or any function, takes a dictionary as an argument, it's the way a dictionary can be "unpacked" to be used as the keyword arguments for a function. Using**kwargs
works for any function that takes keyword arguments. It can also be in the function's defined argument list to accept an arbitrary number of arguments. This is covered in a one of the Treehouse Python modules, if you haven't hit it yet.**kwargs
is one of my favorite python magic bits.This StackOverflow post has more details.