Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial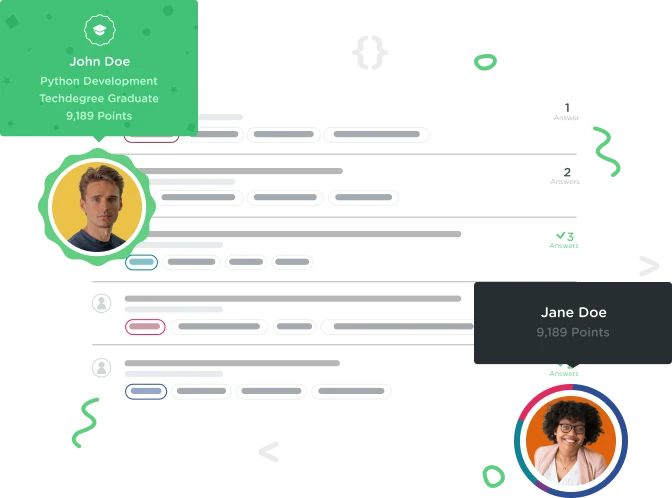

Shawn Mathes
1,826 Pointstimer_text
So, I'm currently following allowing with the Tinter workshop and whenever I try to run this with the block of code listed below, I get an error on pycharm, AttributeError: 'Pymodoro' object has no attribute 'timer_text'. I've been trying my best to pinpoint the problem, but from what I can see everything looks right.
import tkinter
DEFAULT_GAP = 60 * 25
class Pymodoro:
def __init__(self, master):
self.master = master
self.mainframe = tkinter.Frame(self.master, bg="white")
self.mainframe.pack(fill=tkinter.BOTH, expand = True)
self.build_grid()
self.build_banner()
self.build_buttons()
self.build_timer()
self.timer_text = tkinter.StringVar()
self.time_left = tkinter.IntVar()
self.time_left.set(DEFAULT_GAP)
self.running = False # When the app starts it's not running
def build_grid(self):
self.mainframe.columnconfigure(0, weight=1)
self.mainframe.rowconfigure(0, weight=0)
self.mainframe.rowconfigure(1, weight=1)
self.mainframe.rowconfigure(2, weight=0)
def build_banner(self):
banner = tkinter.Label(
self.mainframe,
background='black',
text='Pymodoro',
fg='white',
font=('Helvetica', 24)
)
banner.grid(
row=0, column=0,
sticky='ew',
padx=10, pady=10
)
def build_buttons(self):
buttons_frame = tkinter.Frame(self.mainframe)
buttons_frame.grid(row=2, column=0, sticky='nsew', padx=10, pady=10)
buttons_frame.columnconfigure(0, weight=1)
buttons_frame.columnconfigure(1, weight=1)
self.start_button = tkinter.Button(
buttons_frame,
text='Start',
command=self.start_timer
)
self.stop_button = tkinter.Button(
buttons_frame,
text='Stop',
command=self.stop_timer
)
self.start_button.grid(row=0, column=0, sticky='ew')
self.stop_button.grid(row=0, column=1, sticky='ew')
def build_timer(self):
timer = tkinter.Label(
self.mainframe,
text=self.timer_text.get(),
font=('Helvetica', 36)
)
timer.grid(row=1, column=0, sticky='nsew')
def start_timer(self):
self.time_left.set(DEFAULT_GAP)
self.running = True
def stop_timer(self):
self.running = False
if __name__ == '__main__':
root = tkinter.Tk()
Pymodoro(root)
root.mainloop()
Shawn Mathes
1,826 PointsShawn Mathes
1,826 PointsWell, I ended up figuring out a solution to the issue and it was because I was calling the methods before declaring the variables.