Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial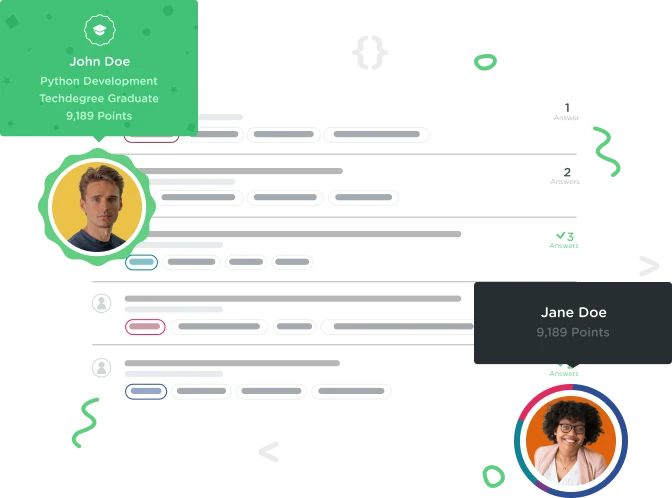

Bailey Smith
1,645 PointsTimestamp challenge
I am trying to do the timestamp challenge: Create a function named timestamp_oldest that takes any number of POSIX timestamp arguments. Return the oldest one as a datetime object.
Remember, POSIX timestamps are floats and lists have a .sort() method.
I am getting the error : 'NoneType' object is not subscriptable
I tried:
import datetime
def timestamp_oldest(*args):
args_list = list(args)
args_list = args_list.sort()
return datetime.datetime.fromtimestamp(args_list[0])
3 Answers

Matthew Carr
11,220 PointsLists get sorted in place. Try the following at a python prompt:
a_list = [5, 2, 1, 6]
b_list = a_list.sort()
print(b_list)
a_list.sort()
print(a_list)
Why does it happen this way? The sort() method for lists is designed to work on the list in place. According to the help in python "help(list.sort)" the sort method always returns None. The list is sorted still exists, and has been changed to a sorted version of itself.

Jing Zhang
Courses Plus Student 6,465 Pointsexample for sorted:
def timestamp_oldest(*timestamps):
return datetime.datetime.fromtimestamp(sorted(timestamps)[0])
or just use min:
def timestamp_oldest(*timestamps):
return datetime.datetime.fromtimestamp(min(timestamps))

wantingedwards
12,177 PointsI always get confused with sort() and the build in function sorted() but from definition, sort() doesn't return a list and that's probably why the Nonetype error. If you change your code to: args_list = sorted(args_list) that should work since it return a list