Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial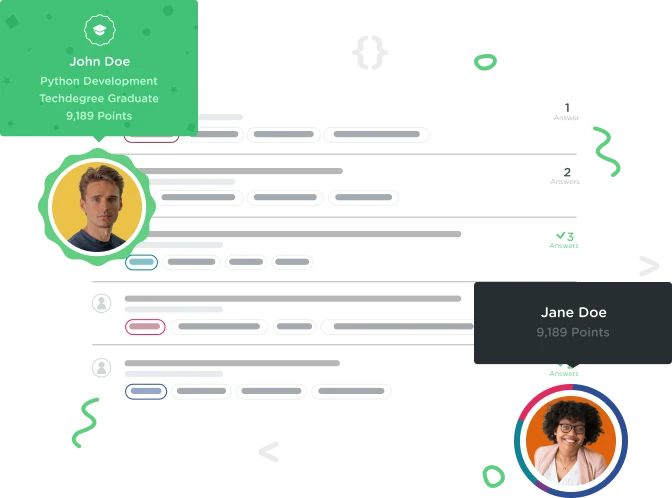
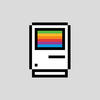
Hakim Rachidi
38,489 PointsTip: use the negation operator
To change the players active state property use the negation operator like this:
switchPlayers() {
this.players[0].active = !this.players[0].active;
this.players[1].active = !this.players[1].active;
}
instead of the for-loop and the ternary operator. Why would we ever have more than 2 player in four-in-a-row? (irony)
2 Answers

Heidi Puk Hermann
33,366 PointsOne of the great things with code is that there are often more than one solution. I also opted for another solution than the ternary operator, simply because I think it is complicated to read. But in the spirit of keeping my code dry, I kept the for-loop and ended with the following solution:
switchPlayers(){
for (let player of this.players) {
player.active = !player.active;
}
}
It is short and easy to read. I do get your point that a for-loop may be a bit overkill when there are only two objects, but to be honest, I disagree...

George Roberts
Full Stack JavaScript Techdegree Student 8,474 PointsI had the same approach but with forEach() rather than for..of:
switchPlayers() {
this.players.forEach(player => player.active = !player.active);
}
Gabbie Metheny
33,778 PointsGabbie Metheny
33,778 PointsThis is exactly what I did, and I agree it's the most readable of those three solutions, but like you said there's so many "right" ways to code the same thing!
Andrew Federico
7,498 PointsAndrew Federico
7,498 PointsI did this as well, but I can appreciate needing to practice ternary operations.
thomas shellberg
11,594 Pointsthomas shellberg
11,594 PointsYep, this was exactly my solution as well. Nice job!
Joe Elliot
5,330 PointsJoe Elliot
5,330 PointsCould someone please explain Heidi's code? To me it looks like it sets every active player to be non active. How does it make a non-active player......active?