Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial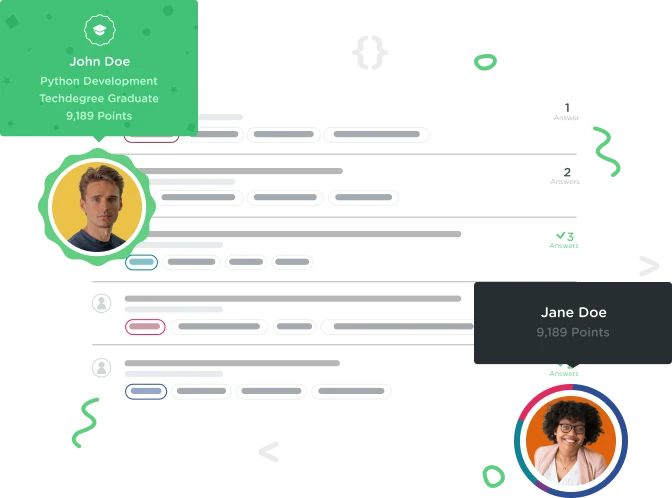

Rhys Kearns
4,976 PointsTips and help refactoring code
Just did the Javascript Loops, Arrays, Objects challenge -
var questions = [
["Who won the last CS:GO Major?", "gambit"],
["Which swedish team was the best in the world in 2015-2016?", "fnatic"],
["Who is currently the best CS:GO player?", "coldzera"],
["Which team has the longest proffesional win streak", "nip"],
["Who is famous for hitting a jumping double kill with the awp", "coldzera"]
]
var answer;
var correct = [];
var correctCount = 0;
var incorrect = [];
var listCorrect = "<ol>";
var listIncorrect = "<ol>";
for (var i = 0; i < questions.length; i++){
answer = prompt(questions[i][0]);
if (answer === questions[i][1]){
correctCount++;
correct.push(questions[i][0]);
}
else {
incorrect.push(questions[i][0]);
}
}
print("<p> You got " + correctCount + " question(s) right.</p>")
print("<h2> You got these questions correct: </h2>")
for (var i = 0; i < correct.length; i++){
listCorrect += "<li>" + correct[i] + "</li>";
}
listCorrect += "</ol>";
print(listCorrect)
print("<h2> You got these questions incorrect: </h2>")
for (var i = 0; i < incorrect.length; i++){
listIncorrect += "<li>" + incorrect[i] + "</li>";
}
listIncorrect += "</ol>";
print(listIncorrect)
function print(message) {
document.write(message);
}
I made this mess of code but im really not sure how to refactor it - it functions as intended but it just looks sloppy?? Also any tips?
1 Answer
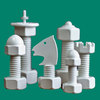
Steven Parker
230,274 PointsYou could create a function to avoid repeating the similar code for showing the two lists:
function printList(list) {
var html = "<ol>";
for (var i = 0; i < list.length; i++) {
html += "<li>" + list[i] + "</li>";
}
html += "</ol>";
print(html);
}
print("<h2> You got these questions correct: </h2>");
printList(correct);
print("<h2> You got these questions incorrect: </h2>");
printList(incorrect);
You would then no longer need the listCorrect and listIncorrect variables.