Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial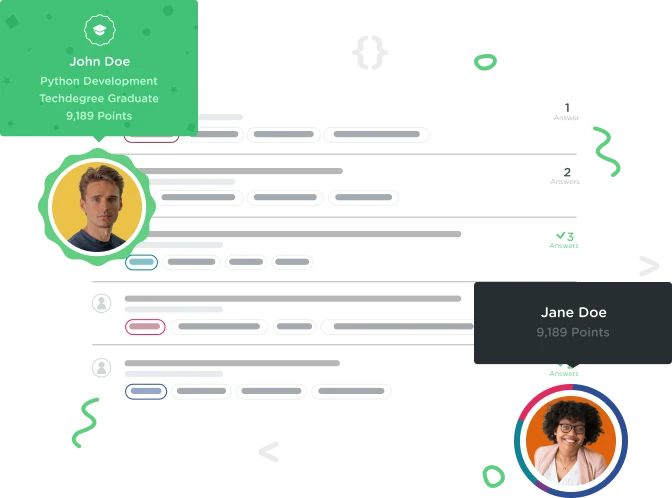
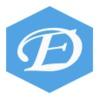
Dennis Elmore
10,547 PointsTips, Feedback, Ect Welcome
So I got to this random number generator challenge and was trying to come up with a solution to the accepting a min and max range. Problem is I've studied JS before and while a lot of this is refreshing my memory, my knowledge isn't as pure as one following the course and learning for the first time.
When I found this difficult, my brain defaulted back to older knowledge. It seems to work fine, but I feel like it's flawed. Here's what I have:
var minNum;
var maxNum;
var randNum = 0;
minNum = parseInt(prompt( 'Please enter the beginning range number' ));
maxNum = parseInt(prompt( 'Please enter the ending range number' ));
while ( randNum < minNum || randNum > maxNum ){
randNum = Math.floor(Math.random()*maxNum) + 1;
//console.log(randNum); -- For test
};
document.write('Your RNG number is ' + randNum + '!');
I feel like what I basically did was built in a fail safe to keep rolling until it hits in the correct range. Looking at it for a start I should have used "lesser/greater than or equal to" because I don't think it'll ever hit the specified range numbers dead on.
The main problem is I know that within the confines of the lessons learned so far in the course, there should be a more straightforward method to accomplish it and hit the right numbers but I'm not seeing it, at least for a min and max. Next video will probably show it, but I still wanted to throw this up.
2 Answers
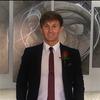
Liam Clarke
19,938 PointsHi Dennis
Yes sometimes overcomplicating makes you miss the easy option and although your way is correct, from a performance perspective, the number equation is a more efficient solution.
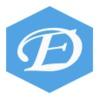
Dennis Elmore
10,547 PointsThat's kinda what I was thinking after I saw the solution video too. Thanks for the feedback.
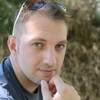
Doron Geyer
Full Stack JavaScript Techdegree Student 13,897 PointsSo I'm reviewing a few exercises here and my code is probably a bit more rudimentary but I believe it works as it should
var guess = parseInt(prompt(" choose your first number"));
var guess2 = parseInt(prompt(" choose your second number"));
if(guess> guess2 ){
var max = guess}else {var max = guess2};
if(guess< guess2 ){
var min = guess}else {var min = guess2};
var random =Math.floor(Math.random()*(max-min+1)+min);
alert(`your random number is ${random}`);
I just used 2 simple if statements to create a new variable to get around the greater and lesser inputs from the user.
also for easier formatting here in JS use three backticks followed directly by js to open and close at the end with another three backticks to format it like mine appears.
Dennis Elmore
10,547 PointsDennis Elmore
10,547 PointsYeah the next video explained it. Not going to post it here because I don't want to spoil it but the big thing I was missing was maxNum - minNum in my randNum equation which would have eliminated the need for the while loop.
Edit: Feedback still welcome.