Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial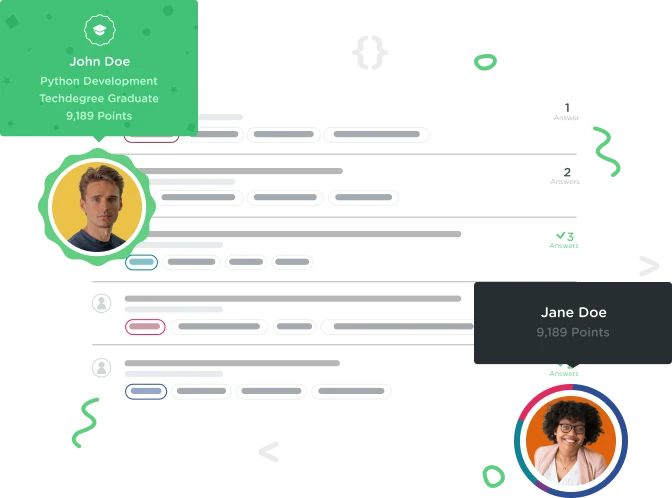

Justin Bonaccorso
2,781 PointsTo do this you're going to use a switch statement and switch on the key
The code challenges seem to be getting very complicated which is understandable, but in the videos we don't cover situations like this, and being a complete novice I get lost very quickly and then become frustrated that I don't have the resources in the videos to accurately address the problem. Any help would be greatly appreciated. I have tried a few different things, and nothing seems to work correctly.
var europeanCapitals: [String] = []
var asianCapitals: [String] = []
var otherCapitals: [String] = []
let world = [
"BEL": "Brussels",
"LIE": "Vaduz",
"BGR": "Sofia",
"USA": "Washington D.C.",
"MEX": "Mexico City",
"BRA": "Brasilia",
"IND": "New Delhi",
"VNM": "Hanoi"]
for (key, value) in world {
// Enter your code below
switch key in world {
case "BEL": results.append = (europeanCapitols[])
case "LIE": results.append(otherCapitals[])
case "BGR": results.append(otherCapitals[])
case "USA": results.append(otherCapitals[])
case "MEX": results.append(otherCapitals[])
case "BRA": results.append(otherCapitals[])
case "IND": results.append(asianCapitals[])
case "VNM": results.append(otherCapitals[])
}
// End code
}

Justin Bonaccorso
2,781 PointsMakes sense Gianpiero, I kept getting stuck at the 'switch key' step before even going further into it. Thank you!
1 Answer

Greg Kaleka
39,021 PointsHi Justin,
You're on the right track! Basically, what we want to do here is append the value to the correct array. Two things to remember (as an aside, remember you can always check the Apple documentation if you're not sure how a method works. This is a great habit to get into!):
- The append method works like this:
nameOfArray.append(valueToAddToArray)
. - One neat feature of switch statements is that you can combine cases if you're doing the same thing.
So putting these two things together, for the three european capitals, we can write:
case "BEL", "LIE", "BGR":
europeanCapitals.append(value)
Does that make sense? The last thing to remember is the default case. Since we have only three arrays to worry about, you can write two sets of cases, and then have the value appended to otherCapitals
in the default case, like this:
default:
otherCapitals.append(value)
I think you can put this all together. Let me know if you have trouble!
Happy coding
-Greg

Justin Bonaccorso
2,781 PointsAfter reading it in this way, it is a lot less confusing. Sometimes I get mixed up with the if/else statements and switch statements, and this outlined it a lot better. Thank You!!!
gianpierovecchi
15,043 Pointsgianpierovecchi
15,043 PointsI don't remember the exercise but probably the idea is that you use switch to select which City is in which regions, and adding them to the respective array,
So the for loop gives you "key" and "value".
The correct answer should be something like:
At the end of the for loop you will have the arrays full with the city names.
Enjoy