Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial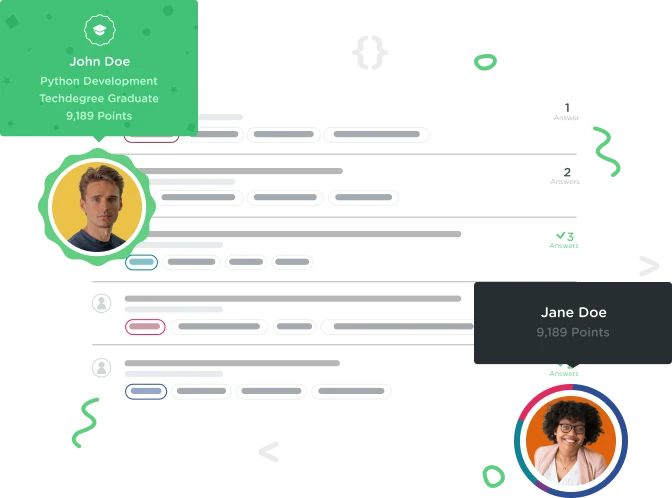

Juan Sosa
4,572 PointsTo generate a random number between 0 and 20.
The real answer must be Math.floor(Math.random()*21)
<!DOCTYPE html>
<html lang="en">
<head>
<title>JavaScript Foundations: The Math Object</title>
<link rel="stylesheet" href="core.css">
<script>
var diameter = 5.75,
a = 17,
b = 42,
c = 1337,
ageIfILiveToYear2100 = (new Date(2100, 0, 1) - new Date(1995, 5, 16, 7, 20)) / (1000 * 60 * 60 * 24 * 365.242);
var circumference = Math.PI * diameter;
var chance = Math.floor(Math.random()*20);
/*
The JavaScript Math object has the following
constant (among others):
Math.PI
Approximately 3.14159. Pi (π) is used
to determine the circumference of a circle
given its diameter by multiplying the diameter
by π. Geometery refresher: the circumference
of a circle is the distance around the outside
edge. The diameter is the width of the circle.
The JavaScript Math object also has the following
methods (among others):
Math.random()
Returns a random number between 0 and 1
Math.max(a, b, ...)
Returns the largest value of the passed
arguments
Math.floor(a)
Returns the value of a number rounded down to
the nearest integer
*/
</script>
</head>
<body>
<h1>JavaScript Foundations</h1>
<h2>The Math Object</h2>
<script src="viewer.js"></script>
</body>
</html>
2 Answers
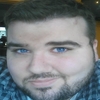
Marcus Parsons
15,719 PointsThe problem with the MDN implementation is that you would still have to use 21 in order to get a value from 0-20, because just as the article states: Returns a random number between min (inclusive) and max (exclusive).
The easy solution to make the max inclusive is just to add 1 inside of the Math.floor statement like so:
function getRandomInt(min, max) {
//Will return a number inside the given range, inclusive of both minimum and maximum
//i.e. if min=0, max=20, returns a number from 0-20
return Math.floor(Math.random() * (max - min + 1)) + min;
}
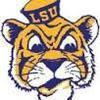
Cena Mayo
55,236 PointsThis code covers all possible ranges, without requiring you to hard code a value (such as 21):
// Returns a random integer between min (included) and max (excluded)
// Using Math.round() will give you a non-uniform distribution!
function getRandomInt(min, max) {
return Math.floor(Math.random() * (max - min)) + min;
}
This is from the MDN page on Math.random.
Juan Sosa
4,572 PointsJuan Sosa
4,572 PointsMath.floor(Math.random()*20), generate numbers between 0 and 19, not 20. Math.round(Math.random()*20), generate numbers between 0 and 20, but the numbers 0 and 20 have half of probability than the others.
The best respond must be Math.floor(Math.random()*21).