Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial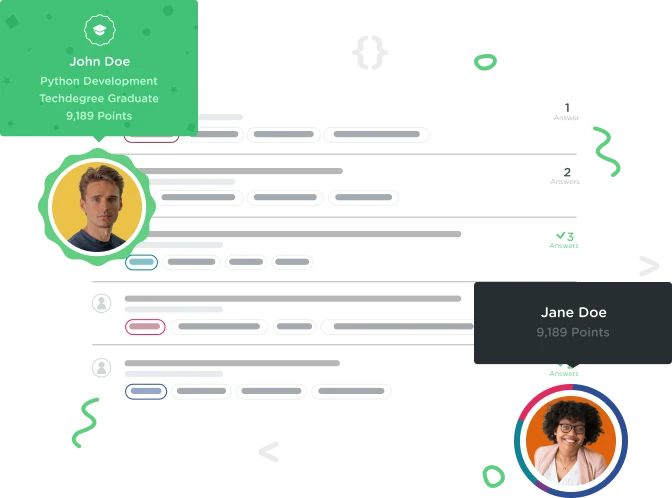

Trainer Workout
22,341 PointsToast does not show after Button click
Any ideas why the Toast does not show on Button click ?
(...)
public class MainActivity extends AppCompatActivity {
public static final String TAG = MainActivity.class.getSimpleName();
private Button downloadButton;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
downloadButton = (Button) findViewById(R.id.download_button);
downloadButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
String message = getString(R.string.downloading);
Log.d(TAG, message);
// Toast does not show after Button click
Toast.makeText(MainActivity.this, message, Toast.LENGTH_SHORT).show();
downloadSong();
} // onClick()
}); // OnClickListener()
} // onCreate()
private void downloadSong() {
(...)
} // downloadSong()
} // MainActivity
2 Answers

Trainer Workout
22,341 PointsThe problem is explained in the video by Ben. To solve this, the downloadSong() method has to be called in a separate thread than the UI thread. In order to this, we need to create a Runnable and start a Thread using that runnable.
downloadButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
String message = getString(R.string.downloading);
Log.d(TAG, message);
Toast.makeText(MainActivity.this, message, Toast.LENGTH_SHORT).show();
Runnable runnable = new Runnable() {
@Override
public void run() {
downloadSong();
} //run()
}; // Runnable()
Thread thread = new Thread(runnable);
thread.setName("DownloadThread");
thread.start();
} // onClick()
}); // OnClickListener()
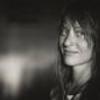
Anastasiia Karpenko
2,413 PointsAlso do not forget to include android:onClick="true" to the Button view in xml file. After I included it , the toast became visible after I clicked the button