Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial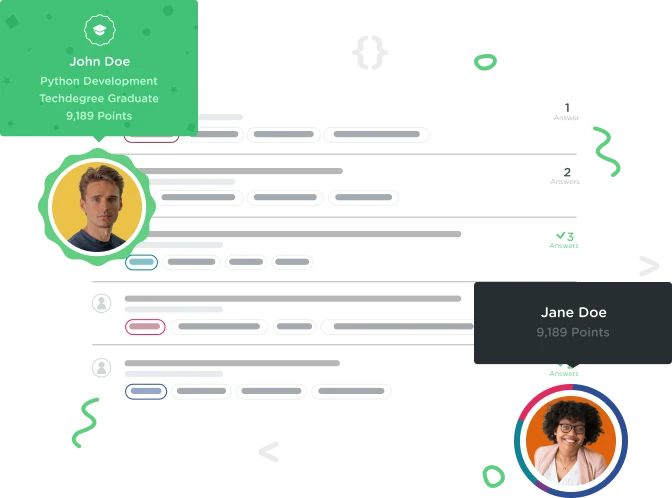
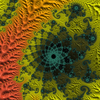
Wes Chumley
6,385 PointsTococat Challenge - Cheese shows as yes every time.
So I passed all the tests and completed the challenge just fine.
However, clearly there wasn't a test for this.
Everytime I make a new taco, it shows Cheese? - Yes . I've tried a few different things to get it to display the Cheese BooleanField correctly but I always get YES in the cheese field.
I will be seeking to figure this out on my own while I await a reply on this, but any help or insight would be appreciated.
NOTE: I have only included the code related to my Taco(Model) class and the rendering of it for the sake of not giving away the code challenge answer. Therefore, this code will not run as it has no imports or database. But I think everything necessary to the issue is there and should be enough for someone to see what I'm doing wrong.
@app.route('/taco', methods=('GET', 'POST'))
@login_required
def taco():
form = forms.PostTacoForm()
if form.validate_on_submit():
models.Taco.create(user=g.user.id,
protein=form.protein.data,
cheese=form.protein.data,
shell=form.shell.data,
extras=form.extras.data.strip())
flash("Taco Posted! Thanks!", "success")
return redirect(url_for('index'))
return render_template('taco.html', form=form)
@app.route('/')
def index():
tacos = models.Taco.select().limit(100)
return render_template('index.html', tacos=tacos)
class Taco(Model):
timestamp = DateTimeField(default=datetime.datetime.now())
user = ForeignKeyField(
rel_model=User,
related_name='tacos'
)
protein = CharField(max_length=25)
shell = CharField(max_length=25)
cheese = BooleanField()
extras = TextField()
class Meta:
database = DATABASE
order_by = ('-timestamp',)
class PostTacoForm(Form):
protein = StringField('Protein', validators=[DataRequired()])
cheese = BooleanField('Cheese', default=False)
shell = StringField('Shell', validators=[DataRequired()])
extras = TextAreaField('Extras', validators=[DataRequired()])
<!doctype html>
<html>
<head>
<title>Tacocat</title>
<link rel="stylesheet" href="/static/css/normalize.css">
<link rel="stylesheet" href="/static/css/skeleton.css">
<link rel="stylesheet" href="/static/css/tacocat.css">
</head>
<body>
{% with messages=get_flashed_messages() %}
{% if messages %}
<div class="messages">
{% for message in messages %}
<div class="message">
{{ message }}
</div>
{% endfor %}
</div>
{% endif %}
{% endwith %}
<div class="container">
<div class="row">
<div class="u-full-width">
<nav class="menu">
<!-- menu goes here -->
<a href="{{ url_for('register') }}">Sign Up</a>
<a href="{{ url_for('login') }}">Log In</a>
{% if current_user.is_authenticated() %}
<a href="{{ url_for('taco') }}">Add a New Taco</a>
<a href="{{ url_for('logout') }}">Log Out</a>
{% endif %}
</nav>
{% block content %}{% endblock %}
</div>
</div>
<footer>
<p>An MVP web app made with Flask on <a href="http://teamtreehouse.com">Treehouse</a>.</p>
</footer>
</div>
</body>
</html>
{% extends 'layout.html' %}
{% block content %}
<h2>Tacos</h2>
{% if tacos.count() %}
<table class="u-full-width">
<thead>
<tr>
<th>Protein</th>
<th>Cheese?</th>
<th>Shell</th>
<th>Extras</th>
</tr>
</thead>
<tbody>
{% for taco in tacos %}
<tr>
<!-- taco attributes here -->
<td>{{ taco.protein }}</td>
<td>{{ taco.cheese }}</td>
<td>{{ taco.shell }}</td>
<td>{{ taco.extras }}</td>
</tr>
{% endfor %}
</tbody>
</table>
{% else %}
<!-- message for missing tacos -->
<h5>no tacos yet</h5>
{% endif %}
{% endblock %}
1 Answer
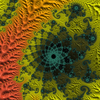
Wes Chumley
6,385 PointsWell I discovered in my '/taco' view that i had cheese = form.protein.data
DUH!
I remember doing that for some testing reason, and forgot to change it back.
Also, before making this discovery, i added to my cheese attribute in my PostTacoForm:
cheese = BooleanField('Cheese', false_values=('False', '', 'false', 'None'))
this may have been the ultimate fixer, but I couldn't see it until I discovered the view issue. Ha.
Chris Freeman
Treehouse Moderator 68,423 PointsChris Freeman
Treehouse Moderator 68,423 PointsGreat work figuring it out!