Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial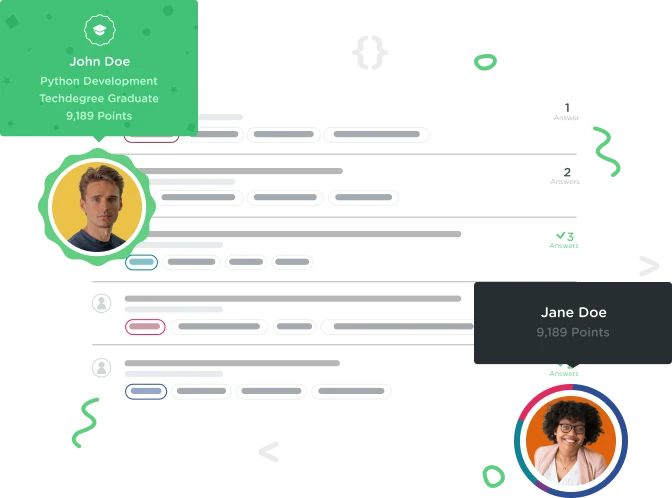

tytyty
4,974 PointsToDo app final exam - Please tell me if I'm on the right track. I don't want to watch the video answer yet.
import UIKit
enum Status {
case Doing
case Pending
case Completed
init() {
self = .Doing
}
}
struct Task {
let Description: String
let status: Status
init(Description: String, status: (Status)) {
self.Description = Description
self.status = Status()
}
func reminderMessage() -> String {
return "Would you like to add a new entry?"
}
}
var task = Task(Description: "Banking", status: Status())
task.Description
task.status
task.reminderMessage()
I spent all frikkn' day on this. I don't know why it was so difficult for me. I think it was because I was trying to add more methods and init values so I was making it harder for myself. Anyway I really tried. No errors in output or console so I think it's ok. I dissected previous challenges etc. I still don't quite know how I got everything together like I did but oh well.
1 Answer
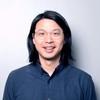
Eric Pan
13,398 PointsLooks like it's on the right track! I just finished it.
Couple of observations that might help you:
- You might want to use
var
instead oflet
for the stored propertiesdescription
andstatus
— at least for thestatus
since that's designed to change, right? - You might want to use lowercase
d
indescription
in the stored property to follow common convention for variable naming. - You don't need the parentheses around
(Status)
in the initialization parameters for theTask
struct. - You might want to indent your initializer in the
Task
struct to make the struct a little easier to read. Just like you did it with the methodreminderMessage
.
Hope that is helpful without giving too much away :)
tytyty
4,974 Pointstytyty
4,974 PointsI added init values for struct and enum for bonus points like it asked.