Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial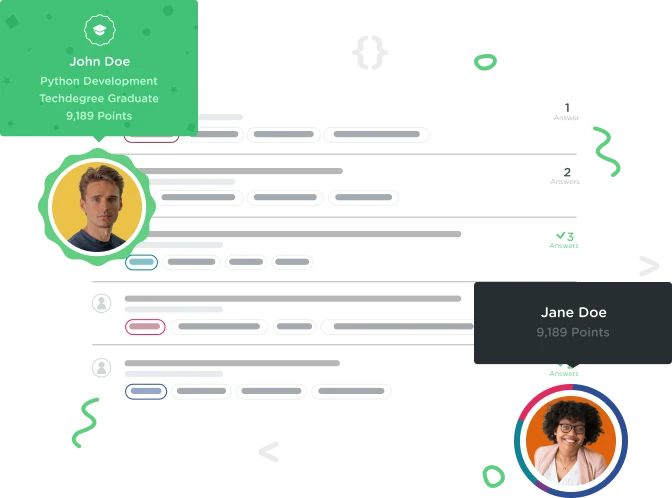
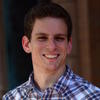
Jeff Lange
8,788 PointsTodo List Project: how does Ruby/Rails know each todo list item is an instance of the TodoList Class?
A huge theme in the Todo List project has been test-driven programming. We have written quite a few tests ensuring things like "title" and "description" for each todo list exist, and are not too short.
The requirements for the todo lists are defined by the TodoList Class in the todo_list.rb file (odot/app/models/todo_list.rb). The code looks like this:
class TodoList < ActiveRecord::Base
validates :title, presence: true
validates :title, length: { minimum: 3 }
validates :description, presence: true
validates :description, length: { minimum: 5 }
end
Simple enough. However, what I don't understand is how Ruby/Rails knows that each todo list item created on Rails--by navigating to localhost:3000/todo_lists and clicking "New Todo list" etc--is an instance of the TodoList Class. I don't remember writing any code to the effect of
when click_button("New Todo list")
new_todo = TodoList.new(title, description)
end
And since we haven't done that, I don't know how the tests we are writing are working, or how Rails knows to throw an error if one fails. Like, for example, we said that title length is a minimum of 3, but how is it that Ruby/Rails knows that filling in the Title box on Rails is defining the title for an instance of the TodoList class? There isn't any code (that I know of) connecting the two.
1 Answer
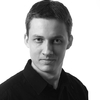
Maciej Czuchnowski
36,441 PointsWhen you created a form in your edit view, you specified what this form relates to - it's something like "form for Todo List". This binds the form and the Submit button to that model. Check your view. and try changing the 'form for' thing :).
Jeff Lange
8,788 PointsJeff Lange
8,788 PointsI went through the views folder and found some .erb files where, just like you said, there was a "form_for" section in one of them. It had an @todo_list, which I assume must be an instance of the TodoList Class.
I still don't totally understand it; however, I am still a newbie at this point and I'm sure it will come with time. Thank you, that definitely helps. At least it doesn't just seem like it "magically" happened anymore. :)
Maciej Czuchnowski
36,441 PointsMaciej Czuchnowski
36,441 PointsWell, Rails is full of magic either way :). Add some gems to it and it seems even more magical (my latest example would be the Decent Exposure gem):
http://easyactiverecord.com/blog/2014/10/16/clean-up-your-rails-controllers-with-decent-exposure/